Final Project Code
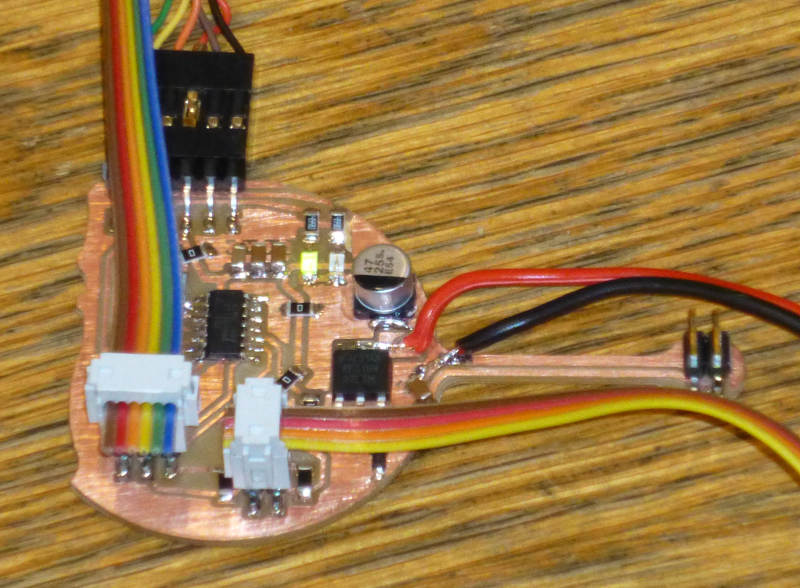
Fan Driver software
//
// fan driver software
//
//
// cobbled together by daniel on 12/13/14.
//
//
#include <avr/io.h>
#include <util/delay.h>
#include <avr/power.h>
#include
#include <avr/pgmspace.h>
#include
#define output(directions,pin) (directions |= pin) // set port direction for output
#define input(directions,pin) (directions &= (~pin)) // set port direction for input
#define set(port,pin) (port |= pin) // set port pin
#define clear(port,pin) (port &= (~pin)) // clear port pin
#define pin_test(pins,pin) (pins & pin) // test for port pin
#define bit_test(byte,bit) (byte & (1 << bit)) // test for bit set
#define bit_delay_time 100 // bit delay for 9600 with overhead
#define bit_delay() _delay_us(bit_delay_time) // RS232 bit delay
#define half_bit_delay() _delay_us(bit_delay_time/2) // RS232 half bit delay
#define led_delay() _delay_ms(100) // LED flash delay
#define char_delay() _delay_ms(10) // char delay
#define serial_port_in PORTB //modified defines from hello.
#define serial_direction_in DDRB
#define serial_pins PINB
#define serial_port_out PORTA
#define serial_direction_out DDRA
#define serial_pin_in (1 << PB2)
#define serial_pin_out (1 << PA7)
#define other_port PORTA // defines for board communication
#define other_direction DDRA
#define other_pins PINA
#define other_pin_in (1 << PA6) //reverse of tempsense definition
#define other_pin_out (1 << PA4)
#define node_id ’99’
#define max_buffer 25
void get_char(volatile unsigned char *pins, unsigned char pin, char *rxbyte) {
//
// read character into rxbyte on pins pin
// assumes line driver (inverts bits)
*rxbyte = 0;
while (pin_test(*pins,pin)); // wait for start bit
half_bit_delay();
bit_delay(); // delay to middle of first data bit
if pin_test(*pins,pin) // unrolled loop to read data bits
*rxbyte |= (1 << 0);
else
*rxbyte |= (0 << 0);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 1);
else
*rxbyte |= (0 << 1);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 2);
else
*rxbyte |= (0 << 2);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 3);
else
*rxbyte |= (0 << 3);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 4);
else
*rxbyte |= (0 << 4);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 5);
else
*rxbyte |= (0 << 5);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 6);
else
*rxbyte |= (0 << 6);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 7);
else
*rxbyte |= (0 << 7);
bit_delay(); // wait for stop bit
half_bit_delay();
}
void put_char(volatile unsigned char *port, unsigned char pin, char txchar) {
//
// send character in txchar on port pin
// assumes line driver (inverts bits)
clear(*port,pin); // start bit
bit_delay();
if bit_test(txchar,0) // unrolled loop to write data bits
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,1)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,2)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,3)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,4)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,5)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,6)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,7)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
set(*port,pin); // stop bit
bit_delay(); // char delay
bit_delay();
}
void put_string(volatile unsigned char *port, unsigned char pin, char *str) {
//
// print a null-terminated string
//
static int index;
index = 0;
do {
put_char(port, pin, str[index]);
++index;
} while (str[index] != 0);
}
void initLEDS(void){
DDRB |= (1 << PB2); //green led
DDRA |= (1 << PA7); //red led
}
void multi_flash(void){ //wagging the dog
uint8_t n = 0;
for (n = 0; n <= 4; n++){
PORTA = 0xff;
PORTB = 0;
_delay_ms(100);
PORTA = 0;
PORTB = 0xff;
_delay_ms(100);
}
}
void initSerialPins(void){ // had to specify port_in and port_out as they are different on my board
set(serial_port_out, serial_pin_out);
output(serial_direction_out, serial_pin_out);
set(serial_port_in, serial_pin_in);
input(serial_direction_in, serial_pin_in);
}
void initOtherPins(void){ // inter[-board communication
set(other_port, other_pin_out);
output(other_direction, other_pin_out);
set(other_port, other_pin_in);
input(other_direction, other_pin_in);
}
void initFan(void){
DDRA |= (1 << PA3);
}
int main(void){
clock_prescale_set(clock_div_1);
//static char chr;
//uint8_t i = 0;
initLEDS();
multi_flash();
initSerialPins();
initOtherPins();
initFan();
while(1){
if (PINA & (1 << PA6)) //When the tempsense module reads over 130 a.u.
PORTA |= (1 << PA3);
else
PORTA &= ~(1 << PA3);
_delay_ms(1000);
}
return(0);
}
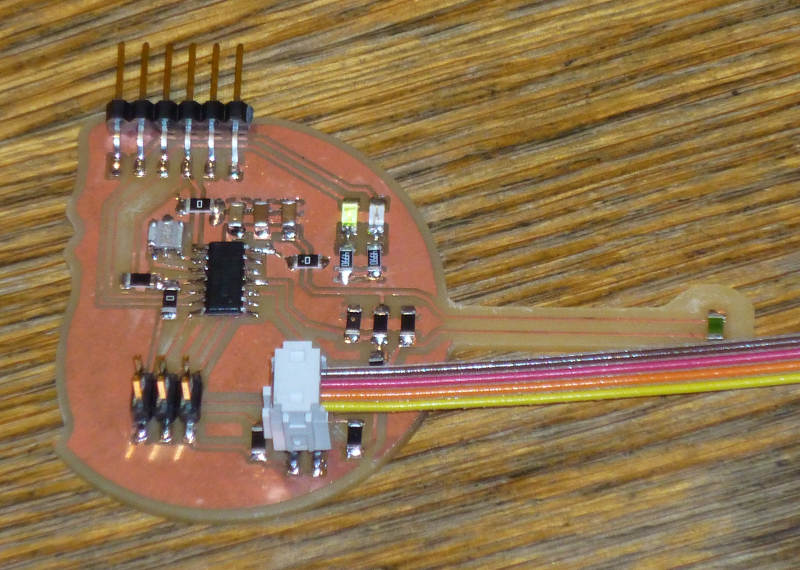
Temperature sensor software
//
// temperature sensor software
//
//
// lifted and altered by daniel on 12/4/14.
//
//
#include <avr/io.h>
#include <util/delay.h>
#include <avr/power.h>
#include
#include <avr/pgmspace.h>
#include
#define output(directions,pin) (directions |= pin) // set port direction for output
#define input(directions,pin) (directions &= (~pin)) // set port direction for input
#define set(port,pin) (port |= pin) // set port pin
#define clear(port,pin) (port &= (~pin)) // clear port pin
#define pin_test(pins,pin) (pins & pin) // test for port pin
#define bit_test(byte,bit) (byte & (1 << bit)) // test for bit set
#define bit_delay_time 100 // bit delay for 9600 with overhead
#define bit_delay() _delay_us(bit_delay_time) // RS232 bit delay
#define half_bit_delay() _delay_us(bit_delay_time/2) // RS232 half bit delay
#define led_delay() _delay_ms(100) // LED flash delay
#define char_delay() _delay_ms(10) // char delay
#define serial_port_in PORTB
#define serial_direction_in DDRB
#define serial_pins PINB
#define serial_port_out PORTA
#define serial_direction_out DDRA
#define serial_pin_in (1 << PB2)
#define serial_pin_out (1 << PA7)
#define other_port PORTA
#define other_direction DDRA
#define other_pins PINA
#define other_pin_in (1 << PA4)
#define other_pin_out (1 << PA6)
#define node_id ’99’
#define max_buffer 25
void get_char(volatile unsigned char *pins, unsigned char pin, char *rxbyte) {
//
// read character into rxbyte on pins pin
// assumes line driver (inverts bits)
*rxbyte = 0;
while (pin_test(*pins,pin)); // wait for start bit
half_bit_delay();
bit_delay(); // delay to middle of first data bit
if pin_test(*pins,pin) // unrolled loop to read data bits
*rxbyte |= (1 << 0);
else
*rxbyte |= (0 << 0);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 1);
else
*rxbyte |= (0 << 1);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 2);
else
*rxbyte |= (0 << 2);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 3);
else
*rxbyte |= (0 << 3);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 4);
else
*rxbyte |= (0 << 4);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 5);
else
*rxbyte |= (0 << 5);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 6);
else
*rxbyte |= (0 << 6);
bit_delay();
if pin_test(*pins,pin)
*rxbyte |= (1 << 7);
else
*rxbyte |= (0 << 7);
bit_delay(); // wait for stop bit
half_bit_delay();
}
void put_char(volatile unsigned char *port, unsigned char pin, char txchar) {
//
// send character in txchar on port pin
// assumes line driver (inverts bits)
clear(*port,pin); // start bit
bit_delay();
if bit_test(txchar,0) // unrolled loop to write data bits
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,1)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,2)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,3)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,4)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,5)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,6)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
if bit_test(txchar,7)
set(*port,pin);
else
clear(*port,pin);
bit_delay();
set(*port,pin); // stop bit
bit_delay(); // char delay
bit_delay();
}
void put_string(volatile unsigned char *port, unsigned char pin, char *str) {
//
// print a null-terminated string
//
static int index;
index = 0;
do {
put_char(port, pin, str[index]);
++index;
} while (str[index] != 0);
}
void initLEDS(void){
DDRB |= (1 << PB2); //green led
DDRA |= (1 << PA7); //red led
}
void runADC(void){
ADCSRA |= (1 << ADEN);
ADCSRA |= (1 << ADATE);
ADCSRA |= (1 << ADSC);
}
void multi_flash(void){
uint8_t n = 0;
for (n = 0; n <= 4; n++){
PORTA = 0xff;
PORTB = 0;
_delay_ms(100);
PORTA = 0;
PORTB = 0xff;
_delay_ms(100);
}
}
void initADC23(void){
ADMUX |= ((0 << REFS1)|(0 << REFS0)); //VCC ref.
ADMUX |= 0b00000011; //PA3 no gain
ADCSRA |= ((1 << ADPS2)|(1 << ADPS1)|(1 << ADPS0)); //ADC clock /128
ADCSRA |= (1 << ADEN); //enable!
ADCSRB &= ~(1 << BIN); //not bipolar
ADCSRB |= (1 << ADLAR);
DIDR0 |= ((1 << ADC2D)|(1 << ADC3D)); //turn off digital buffers on PA2 and PA3
initLEDS();
multi_flash();
}
void initSerialPins(void){ //modularizing example code
set(serial_port_out, serial_pin_out);
output(serial_direction_out, serial_pin_out);
set(serial_port_in, serial_pin_in);
input(serial_direction_in, serial_pin_in);
}
void initOtherPins(void){ //modifying and modularizing example code
set(other_port, other_pin_out);
output(other_direction, other_pin_out);
set(other_port, other_pin_in);
input(other_direction, other_pin_in);
}
void sendDecimal(uint8_t number){ //from Make:AVR programming
put_char(&serial_port_out, serial_pin_out, ‘0’ + (number/100));
put_char(&serial_port_out, serial_pin_out, ‘0’+ (number/10) % 10);
put_char(&serial_port_out, serial_pin_out, ‘0’ + (number % 10));
put_char(&serial_port_out, serial_pin_out, 32);
}
int main(void){
clock_prescale_set(clock_div_1); /* I can see why coding is so much like working in the machine shop. There are so many ways of getting to product. My code guru, Allen Crockett, is not happy with my mixing up styles. I respect his aesthetic, but must stay true to my own, which I confess could be called erratic. */
initADC23();
static char chr;
initSerialPins();
initOtherPins();
//uint16_t Tdata,T32 = 0;
// uint8_t i = 0;
//char what = 25;
// initLEDS();
// runADC();
while (1) {
//
// send framing
//
/* put_char(&serial_port_out, serial_pin_out, ‘1’);
char_delay();
put_char(&serial_port_out, serial_pin_out, ‘2’);
char_delay();
put_char(&serial_port_out, serial_pin_out, ‘3’);
char_delay();
put_char(&serial_port_out, serial_pin_out, ‘4’);
char_delay();
*/
_delay_ms(1000);
ADCSRA |= (1 << ADSC); // initiate conversion
while (ADCSRA & (1 << ADSC)); // send result chr = ADCH; sendDecimal(chr); char_delay(); if(ADCH > 130) {
PORTA &= ~(1 << PA6);
} //thermo-switch by arb.units: freezer=52,RT~120,finger=142
else PORTA |= (1 << PA6);
}
return(0);
}