11 Networking
I used the circuits I created (from week 5 and 9) to let them communicate with each other. The Rx, Tx pins are cross connected to each other. The master circuit (on the right) sends '1' and '0's to the slave circuit (on the left) and the slave circuit's LED blinks accordingly.
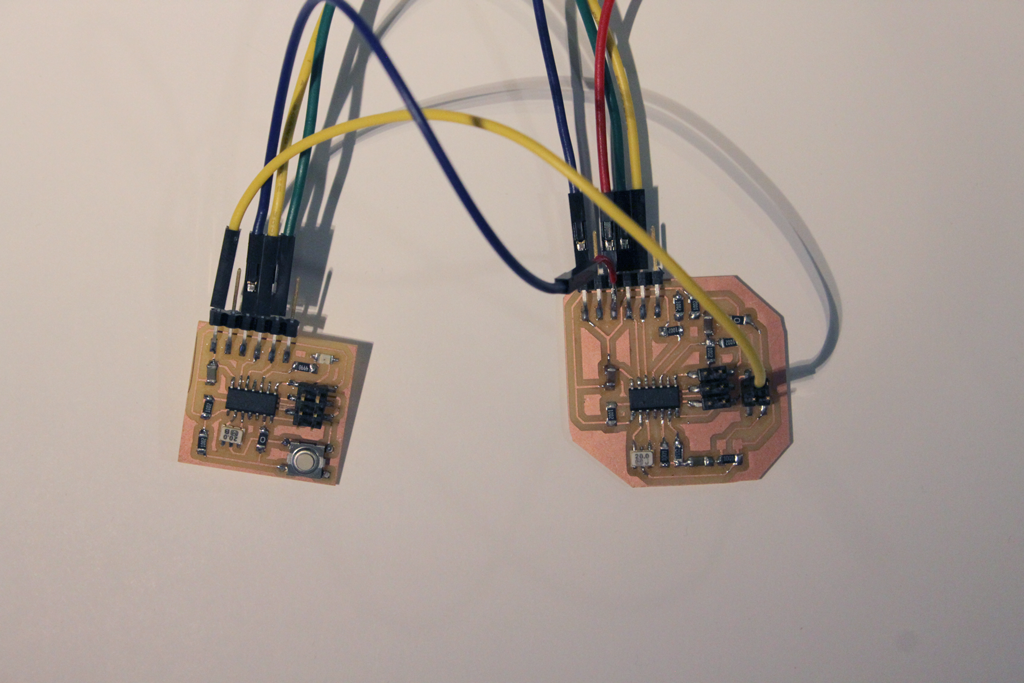
Code (master)
#include <SoftwareSerial.h>
#define rxPin 0
#define txPin 1
SoftwareSerial mySerial(rxPin, txPin);
void setup()
{
mySerial.begin(9600);
}
void loop()
{
mySerial.write('1');
delay(2000);
mySerial.write('0');
delay(2000);
}
Code (slave)
#include <SoftwareSerial.h>
#define rxPin 0
#define txPin 1
SoftwareSerial mySerial(rxPin, txPin);
int led = 3;
char r;
void setup()
{
mySerial.begin(9600);
pinMode(led, OUTPUT);
}
void loop()
{
while (mySerial.available())
{
r = mySerial.read();
if(r=='1'){
digitalWrite(led, HIGH); // turn the LED on (HIGH is the voltage level)
}
else if(r=='0'){
digitalWrite(led, LOW); // turn the LED off by making the voltage LOW
}
}
}
The blink speed changes based on the delay assigned from the master side.
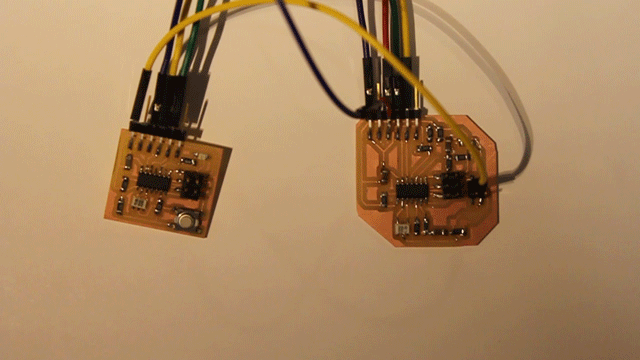
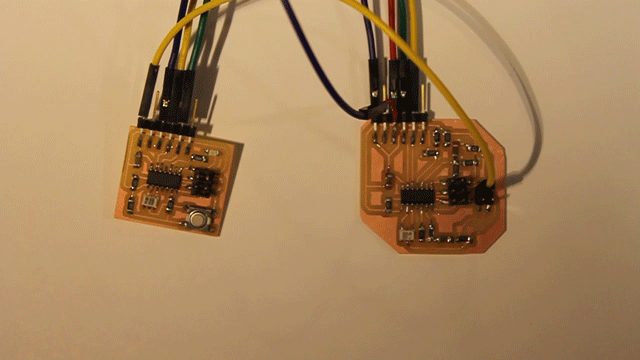
Copyright © 2014 Sang-won Leigh