Project 12 - More Programming...
Processing is an open source programming language and development environment for visual graphics. It is very similar to Arduino, and the two programs can play together so that Processing creates a visual display for actions that are being controlled in Arduino.
I connected an Arduino script, which turned pins on/off and also output a serial message, to a program in Processing, which flashed a different image depending on the serial messages it read. The pins the Arduino code was controlling were connected to slices of a material called a SmartTint, which switches between transparent when powered on and opaque when off. I cut and stacked slices of this SmartTint, spaced them out with pieces of clear acrylic, and rotated through which ones were powered. I connected the pins to one side of a relay and a power supply to the other so that I could have a higher voltage go into the SmartTint sheets. Because I didn't do this project in Boston or in a FabLab I used a micro-controller I had around, it's the same thing though.
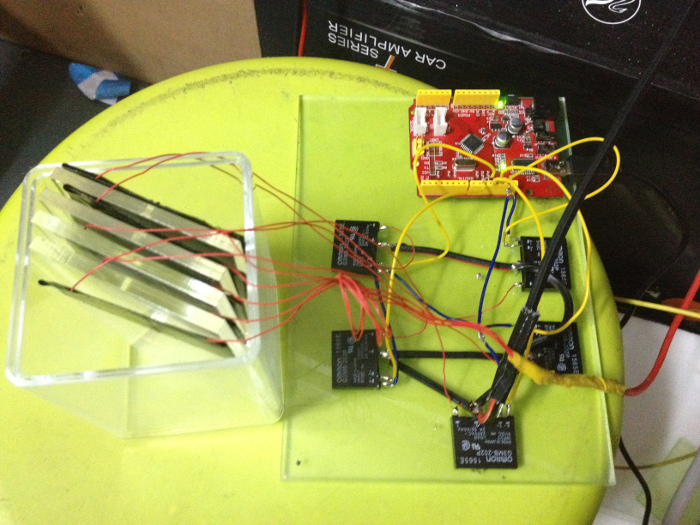
The Processing code then read which slice was on from the serial and displayed a different colored circle depending on which layer was opaque. This made a really crude 5 level display when I shined the Processing image on the stacks with a peco projector. The image would go through all the clear slices and display on the opaque one. The SmartTint takes about 25 milliseconds to become opaque again though, so it wasn't fully functional as a 3D display because you need a faster cycle rate for your persistence of vision.
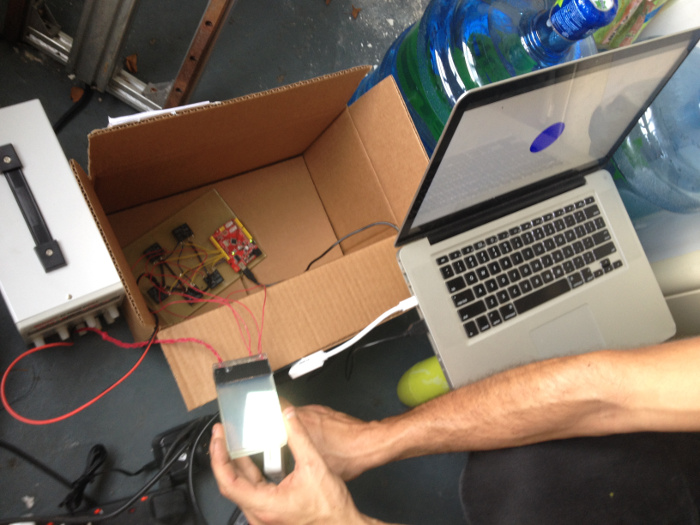
Here's a gif of the what the Processing code projects.
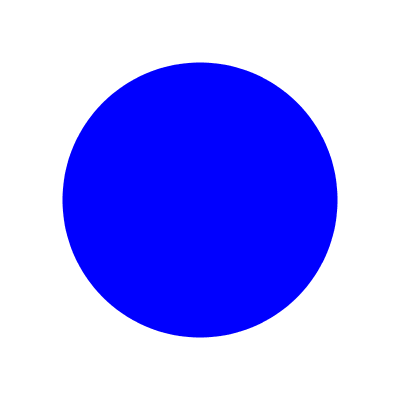
Aduino Code
/*Flashing between the different SmartTint boards, connect with Processing to make colored cylinder*/
int ln1 = 10;
int ln2 = 11;
int ln3 = 12;
int ln4 = 13;
int tm_dlay = 500; //in milliseconds (25 fastest for SmartTint)
// the setup routine runs once when you press reset:
void setup() {
//initialize serial communications at a 9600 baud rate
Serial.begin(9600);
// initialize the digital pin as an output.
pinMode(ln1, OUTPUT);
pinMode(ln2, OUTPUT);
pinMode(ln3, OUTPUT);
pinMode(ln4, OUTPUT);
digitalWrite(ln1,HIGH);
digitalWrite(ln2,HIGH);
digitalWrite(ln3,HIGH);
digitalWrite(ln4,HIGH);
}
// the loop routine runs over and over again forever:
void loop() {
digitalWrite(ln1, LOW);
Serial.println("ln1"); //send over the serial port
delay(tm_dlay); // wait
digitalWrite(ln1, HIGH); // turn the LED off by making the voltage LOW
digitalWrite(ln2, LOW);
Serial.println("ln2"); //send over the serial port
delay(tm_dlay); // wait
digitalWrite(ln2, HIGH); // turn the LED off by making the voltage LOW
digitalWrite(ln3, LOW);
Serial.println("ln3"); //send over the serial port
delay(tm_dlay); // wait
digitalWrite(ln3, HIGH); // turn the LED off by making the voltage LOW
digitalWrite(ln4, LOW);
Serial.println("ln4"); //send over the serial port
delay(tm_dlay); // wait
digitalWrite(ln4, HIGH); // turn the LED off by making the voltage LOW
}
Processing Code
//Multi-Colored Cylinder, connects w/ Arduino "flash" code, and saves a GIF
import processing.serial.*;
import gifAnimation.*;
Serial myPort; // Create object from Serial class
int tm_delay = 500; //in milliseconds (25 fastest for SmartTint)
int frames = 0;
GifMaker gifExport;
public void setup() {
smooth();
size(400, 400);
noFill();
background(255);
noStroke();
frameRate(1); //number of frames per second
println("gifAnimation " + Gif.version());
gifExport = new GifMaker(this, "circles.gif");
gifExport.setRepeat(0); // make it an "endless" animation
String portName = Serial.list()[0]; //change the 0 to a 1 or 2 etc. to match your port
myPort = new Serial(this, portName, 9600);
}
void draw() { // draw() loops forever, until stopped
if (frameCount % 4 == 0){
myPort.write('1');
fill(255,0,0);
ellipse(width/2,height/2,275,275);
} else if (frameCount % 4 == 1){
myPort.write('2');
fill(0,0,255);
ellipse(width/2,height/2,275,275);
} else if (frameCount % 4 == 2){
myPort.write('3');
fill(0,255,0);
ellipse(width/2,height/2,275,275);
} else if (frameCount % 4 == 3){
myPort.write('4');
fill(255,255,0);
ellipse(width/2,height/2,275,275);
}
export();
}
void export() {
if(frames < 4)
{
gifExport.setDelay(500);
gifExport.addFrame();
frames++;
}
else
{
gifExport.finish();
frames++;
println("gif saved");
exit();
}
}