Week 8
Embedded Programming
This week was a good introduction to Arduino and programming in C to learn how to make a board do something. Here are three quick examples of what I could do!
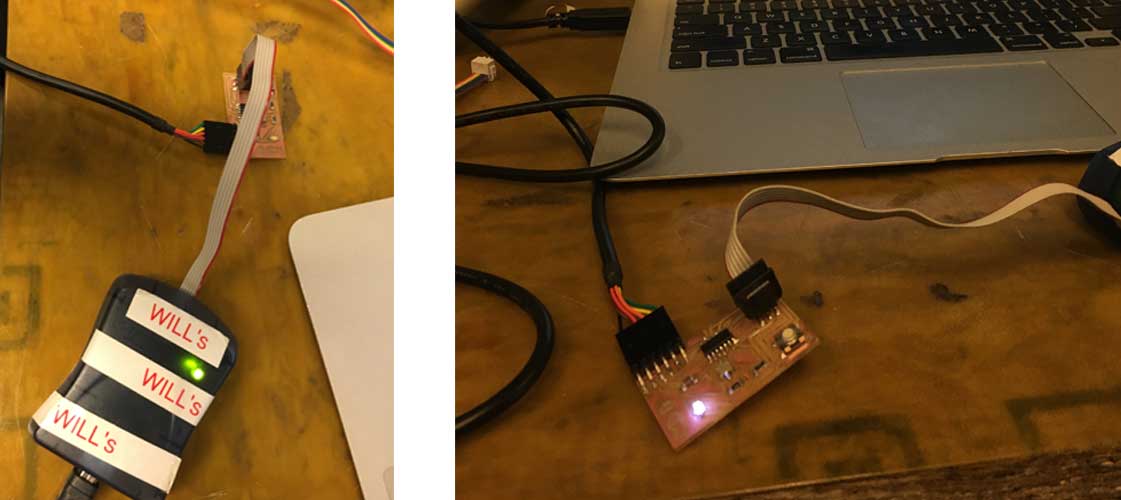
This week, I used Arduino to program my board. I found it to be pretty simple and intuitive to get around the environment, and it required relatively little effort to program the board.
First, I connected my board from two weeks ago to my own ISP, but it didn't work. I instead used Will's AVRISP mkII.
I set the board, processor, clock, and port (on Macs, you need to download a separate driver to find the port).
Finally, I found some code, played around and tried to get new things to happen. Here are some examples of what I have done!
int ledPin = 7; // the number of the LED pin int bPin = 2; int bState = 0; void setup() { // set the digital pin as output: pinMode(ledPin, OUTPUT); pinMode(bPin, INPUT); digitalWrite(bPin, HIGH); } void loop() { bState = digitalRead(bPin); if(bState == HIGH){ digitalWrite(ledPin, LOW); } else{ digitalWrite(ledPin, HIGH); } }
int ledPin = 7; // the number of the LED pin int bPin = 2; int bState = 0; int brightness = 0; // how bright the LED is int fadeAmount = 3; // how many points to fade the LED by void setup() { // set the digital pin as output: pinMode(ledPin, OUTPUT); pinMode(bPin, INPUT); digitalWrite(bPin, HIGH); } void loop() { bState = digitalRead(bPin); if(bState == HIGH){ analogWrite(ledPin, brightness); // change the brightness for next time through the loop: brightness = brightness + fadeAmount; // reverse the direction of the fading at the ends of the fade: if (brightness == 0 || brightness == 255) { fadeAmount = -fadeAmount ; } // wait for 30 milliseconds to see the dimming effect delay(30); } else{ digitalWrite(ledPin, LOW); // if button is pressed, then LED is off } }
int ledPin = 7; // the number of the LED pin int bPin = 2; int bState = 0; int randomNum = 0; void setup() { // set the digital pin as output: pinMode(ledPin, OUTPUT); pinMode(bPin, INPUT); digitalWrite(bPin, HIGH); } void loop() { if(digitalRead(bPin) == LOW){ analogWrite(ledPin, randomNum); } if(digitalRead(bPin) == HIGH){ randomNum = random(0,255); // if button is pressed, then LED is off } }