Week 13 - Networking and Communications
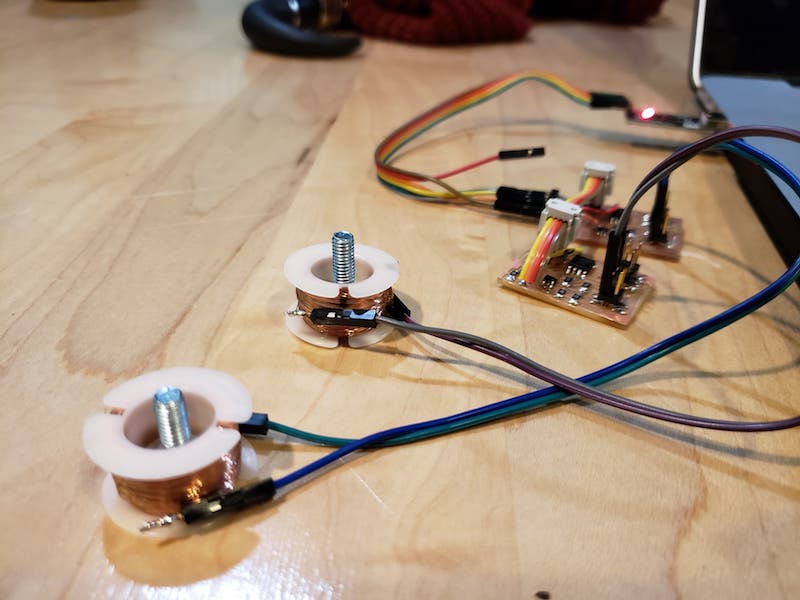
This week I wanted to get two magnetic pickup boards to talk to my computer at the same time, which meant I needed to get the magnetic pickups working first. This took the full week, but eventually I did get two boards working.
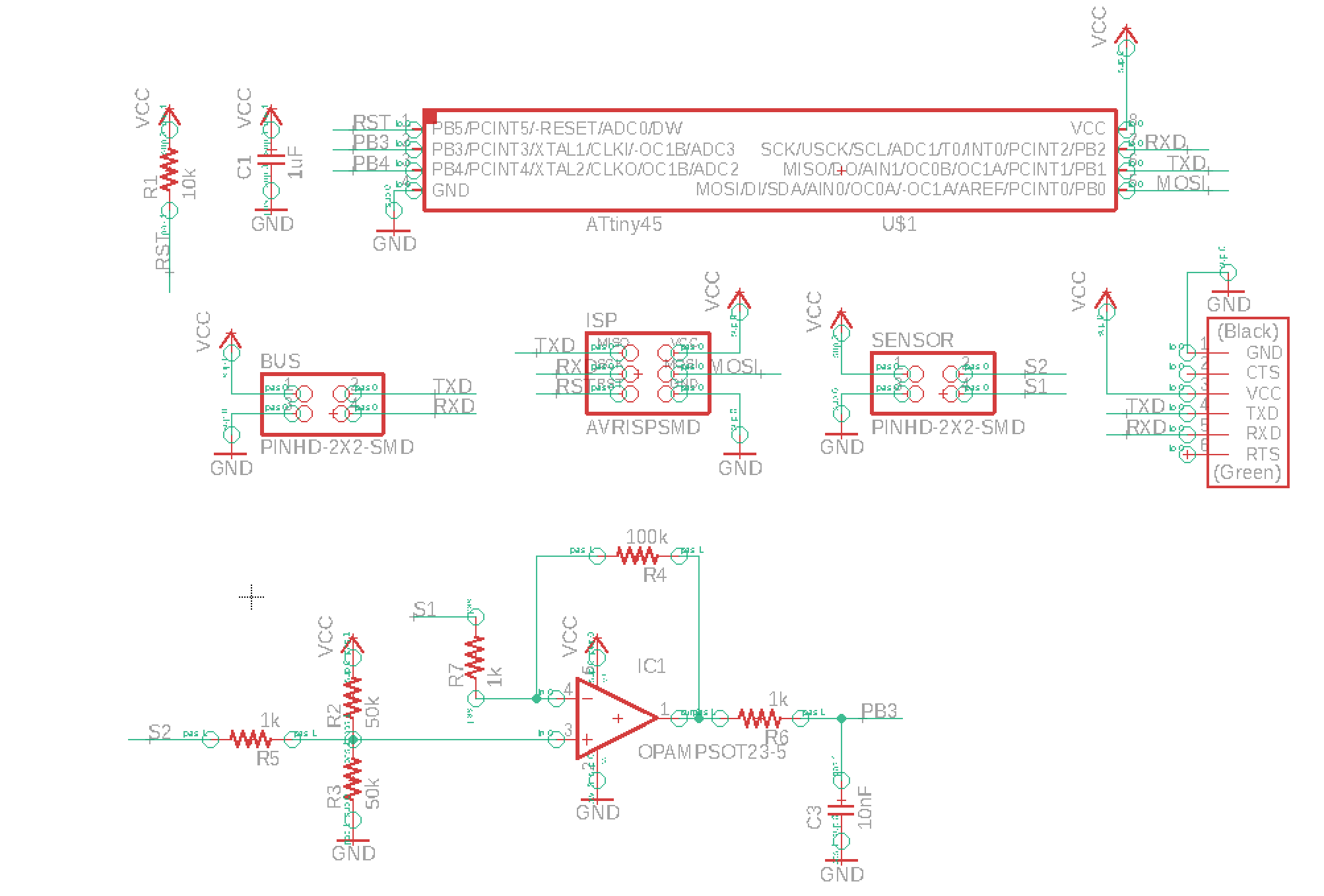

I started with week with some board issue – forgot to include a trace, and another ripped off while soldering, so this made for lots of jumper wires. There was also a short under the opamp that I had to take care of. Through this, I realized that I have indeed gotten better at debugging and fixing my boards by myself.
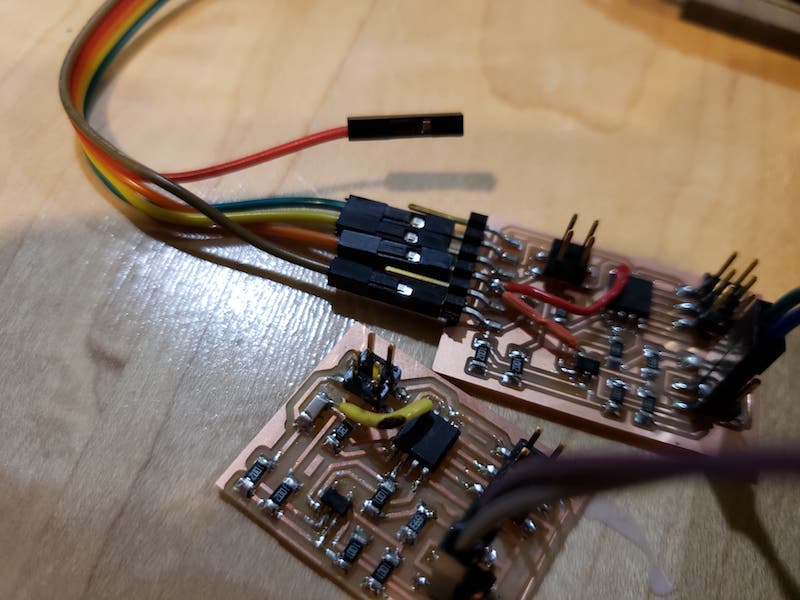
Once I got the boards working individually, sending readings to the computer, I started working on getting the boards to only send readings when prompted serially. I started working with Neil’s code, but for the longest time I wasn’t getting anything at all. I even went back to the hello-world code, that I knew worked, but that didn’t work either. Turns out, I didn’t have the TXD pin plugged in, since I didn’t need it for earlier iterations (output only). There goes an hour I’m never getting back. Oh well, you live and learn.
Once I got the blocking version working, I put the code in an interrupt, so the board can continue to take readings in the background, and report a “strum” later if it happened.
Interrupt:
ISR(PCINT0_vect) {
// pin change interrupt handler
static char chr;
get_char(&serial_pins, serial_pin_in, &chr);
if (chr == node_id) {
// send if a strum has happened and reset
output(serial_direction, serial_pin_out);
put_char(&serial_port, serial_pin_out, is_strum);
is_strum = 0;
input(serial_direction, serial_pin_out);
}
}
Main loop:
while (1) {
// initiate conversion
ADCSRA |= (1 << ADSC);
// wait for completion voltage analog -> digital
while (ADCSRA & (1 << ADSC));
chrl = ADCL;
chrh = ADCH;
int value = 256 * chrh + (unsigned char)chrl;
if (value < LOW_THRESHOLD|| value > HIGH_THRESHOLD) {
is_strum = 1;
} else {
is_strum = 0;
}
}
Python code that sends out prompts and reads output:
for i in range(NUM_BOARDS):
ser.flush()
ser.write(str(i))
out = ord(ser.read())
if out == 1:
print("strum", i)
This works! The program prints “strum” with the board number. I programmed a different board with a different node_id, plug them both in… and it doesn’t work. The python code was blocking on the ser.read(), which means I wasn’t getting any output from the board. After a bit of digging, I realized I had intialized the output_pin as output, not input. Changed it, and it works now!
Files
mag0.c
mag_bus.py
mag0_board.png
mag0_outline.png
mag1_board.png
mag1_outline.png