Week 10: Output Devices
For this week, I repurposed a board to control a servo. Right now, it just goes through some programmed movements as a test for my final project. It will need to be integrated with some 3D printed parts and the other mechanisms for a full test. The servo I'm using is the HKSCM9-5 from Hobby King.
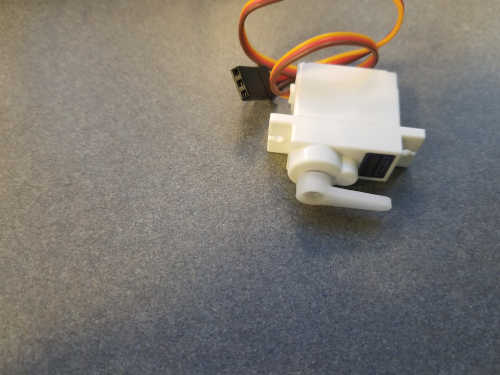
https://hobbyking.com/en_us/hobbykingtm-hkscm9-5-single-chip-digital-servo-1-4kg-0-09sec-10g.html
Unfortunately, a datasheet for this servo is not available, so I have to guess at the proper parameters to control it. These servos can be hacked to have 360 continuous rotation, but in their standard configuration, they are limited to 180 degrees of motion. In any case, these servos are usually controlled by PWM. The ATmega328 does have hardware support for PWM on certain pins, but I used a software solution to have flexibility to use any pin.
long MIN_PWM = 1000;
long MAX_PWM = 2000;
long PERIOD = 20000;
void setup() {
pinMode(DATA_PIN, OUTPUT);
}
void loop() {
for (int angle = 0; angle <= 180; i++) {
long start = micros();
digitalWrite(DATA_PIN, HIGH);
long duration = MIN_PWM + (angle / 180.0) * (MAX_PWM - MIN_PWM);
while (micros() - start < duration) {}
digitalWrite(DATA_PIN, LOW);
while (micros() - start < PERIOD) {}
}
for (int angle = 180; angle >= 0; i--) {
long start = micros();
digitalWrite(DATA_PIN, HIGH);
long duration = MIN_PWM + (angle / 180.0) * (MAX_PWM - MIN_PWM);
while (micros() - start < duration) {}
digitalWrite(DATA_PIN, LOW);
while (micros() - start < PERIOD) {}
}
}