Week 7: Embedded Programming
Hello World
For this week, I coded two boards to output "Hello World" into their serial output. The first was an easy modification of the board I made for the electronics design week which used an ATtiny45. The second was a new board which used the ATmega328. That board was a little more troublesome to setup, but I'll probably need the extra pins for my final project. I designed the new board in Eagle. Unfortunately, I forgot the resistor and capacitor needed on the reset line, so those had to be added in later. It's just a 10k resistor and a 0.1 uF capacitor. The capacitor looks strange because it's a foil which I used just because it was lying around.
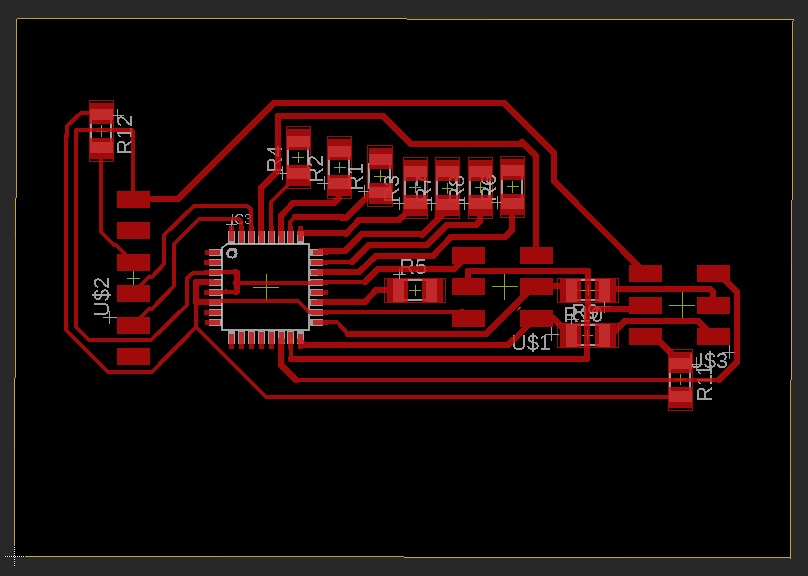
traces.png
outline.png
board.brd
schematic.sch
The ATtiny45 is coded similarly to the board in the electronics design week.
char text[] = {'H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', 'd'};
while (1) {
for (int i = 0; i < 11; i++) {
put_char(&serial_port, serial_pin_out, text[i]);
}
_delay_ms(1000);
}
main.c
The ATmega328 is basically the guts of an Arduino, so you can actually program it using the standard Arduino tools. I followed a nice guide from 2014 by Dan.
http://fab.cba.mit.edu/classes/863.14/people/dan_chen/output-devices/
void setup() {
Serial.begin(115200);
}
void loop() {
Serial.println("first");
delay(1000);
}
main.ino