Week 11: Networking and Communications
Goal: Design, build, and connect wired or wireless node(s) with network or bus addresses
Motivation and Design
Because my final project does not have any networking involved with it, this week I decided to have some fun with a project I worked on in the past. The goal was to set up addressable leds in my room that react to music playing on my spotify. The focus will be on setting up bluetooth communication between my computer running a local server + front end and an esp32 connected to the LEDs. In order to do this I used the ESP32-wroom hello world board with slight modifications to run a data line to the leds as well as power and ground.
PCB
I won't go through all the steps of making the board as this has been documented in past weeks so here is the final board.
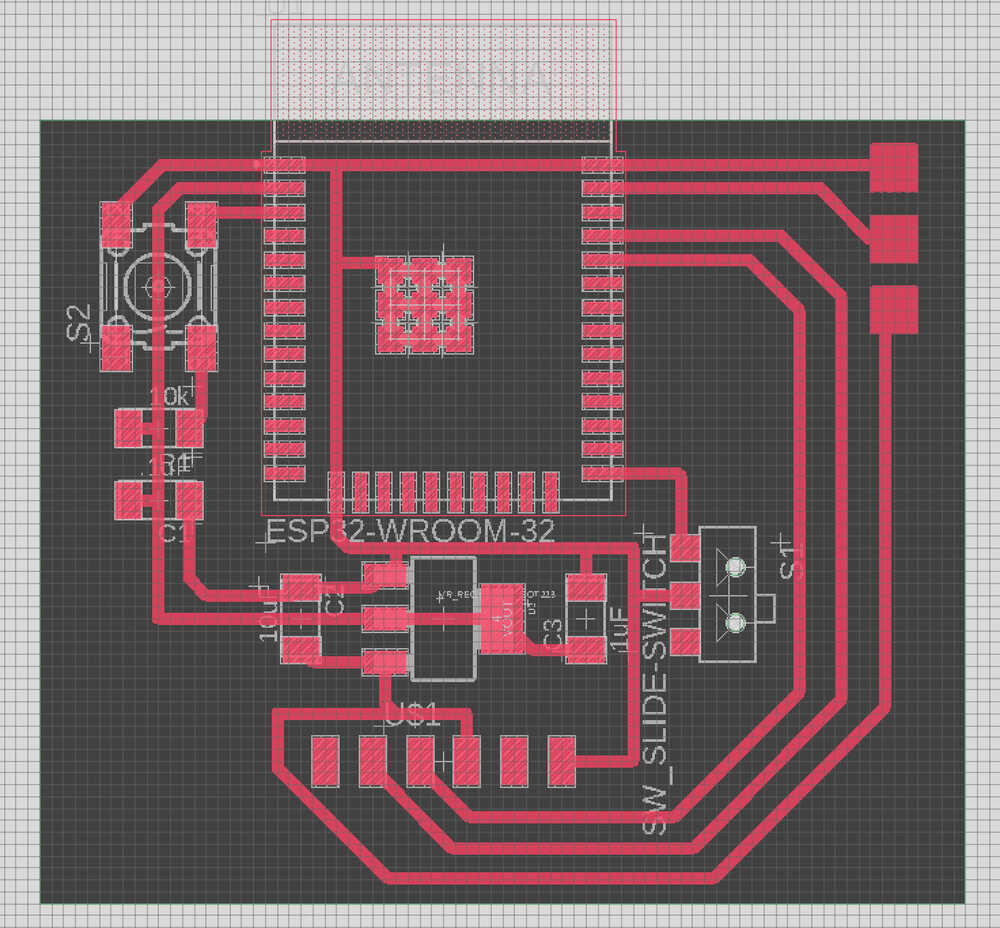
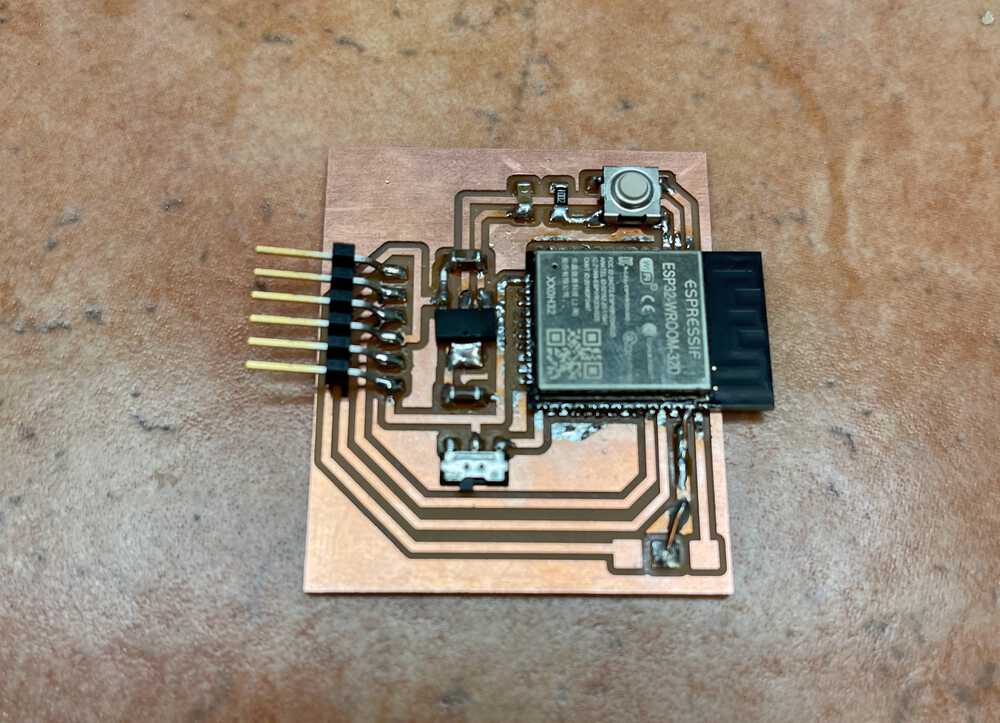
Problems (and Solutions)
I ran into a couple problems this week when making my board. First, the pads on the ESP32-wroom board I found online were way too close together so the design rule check was throwing errors and when I went to mill it, the paths would not go between pads. In order to fix this I learned how to edit the design footprint to have more room. However, when I switched out the old footprint with the new footprint, it happened to delete one of my traces which I did no catch so I ended up having to run a small piece of wire from pin 23 to where I planned to connect my LED strip's data pin. The second problem I faced was actually soldering the ESP32 to my pcb (those pins are tiny!). I attempted to do the strategy of soldering all of the pins together with a lot of solder then using the solder-wick to remove enough so that they were no longer touching. I thought I was successful, but after I finished the board and went to program it I ran into a couple problems with some of the pins still being shorted even though I couldn't really see that they were with my bare eyes. The worse culprit of this was that my IO0 pin was shorted to the neighboring IO4 pin which was causing the error shown to the right when running code on the device. It was a tricky bug however, because I was able to load code effectively even with this short.
I also ran into some problems connecting the 6-pin uart header to my computer for programming. I tried two different usb to uart boards, however neither worked so I ended up borrowing a dedicated cord to do it from Anthony and then things worked fine. For some reason the boards were not outputting a high enough voltage (3.3v instead of 5v) because they were converting on the boards themselves before hitting the voltage regulator on my pcb.
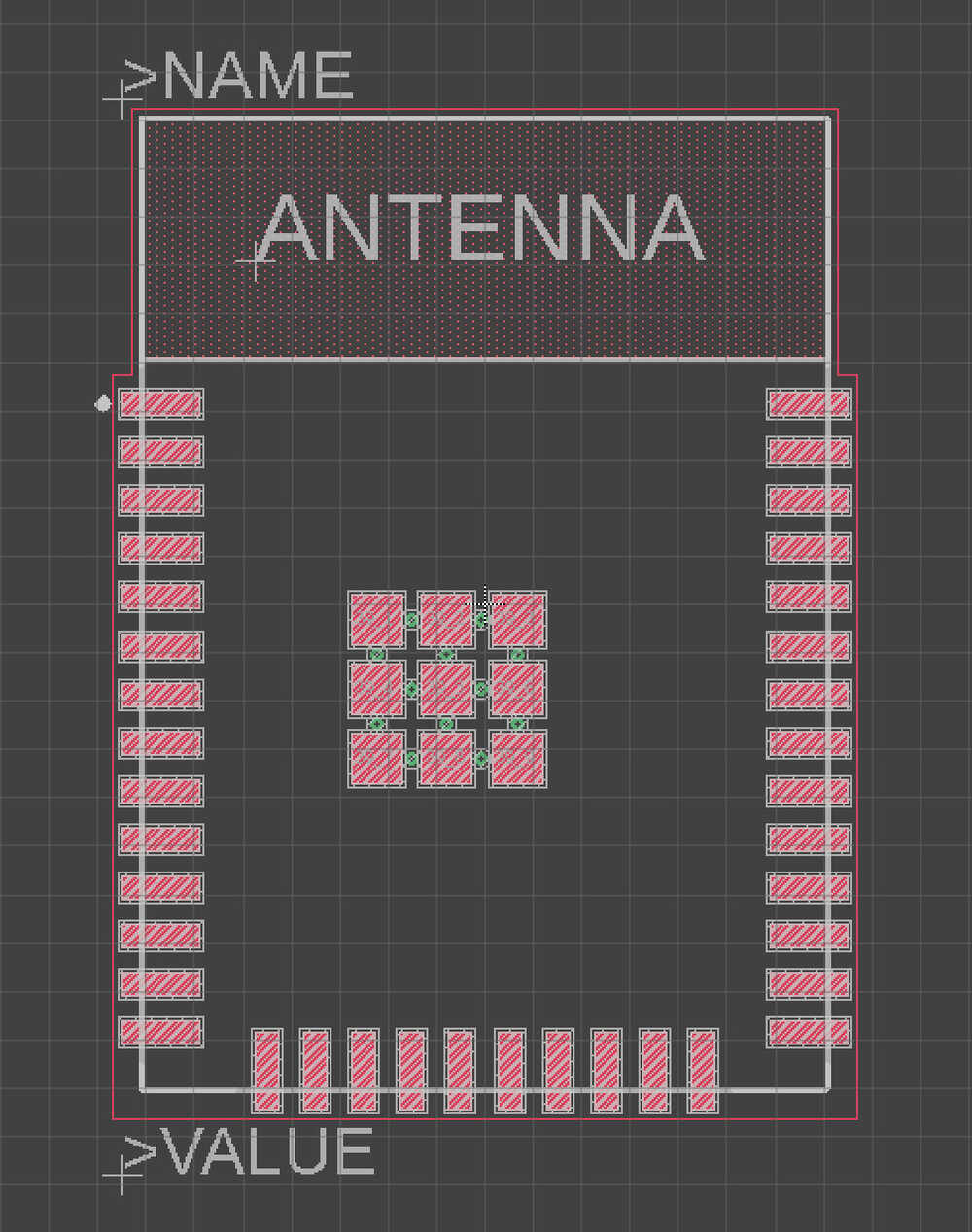
Problems Pictured
Here are some images of the problems/solutions. First, the edit of the footprint for the ESP32. Second, the jumper wire connecting my data pad with pin 23 on the ESP32. Third, the error I was running into when my IO0 pin was shorted making it to where the code would not run after being uploaded.
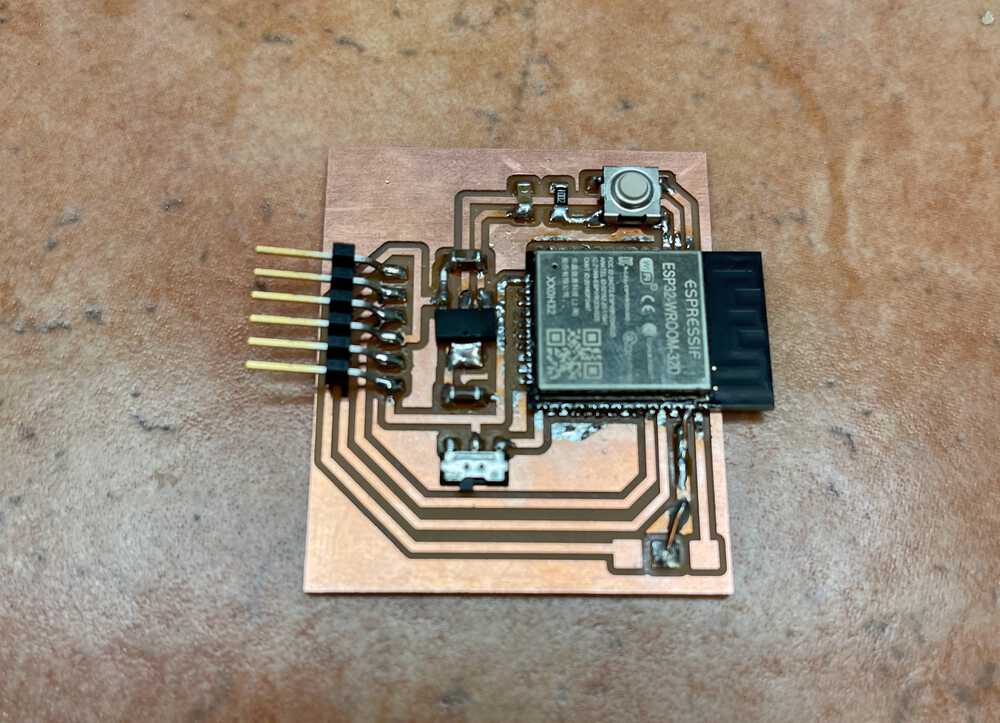

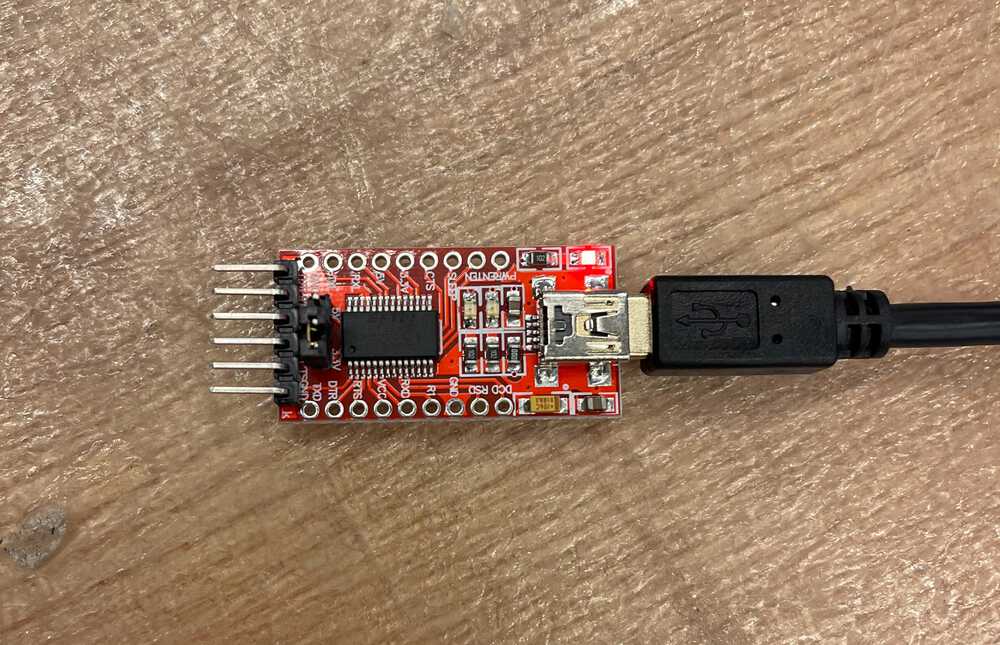
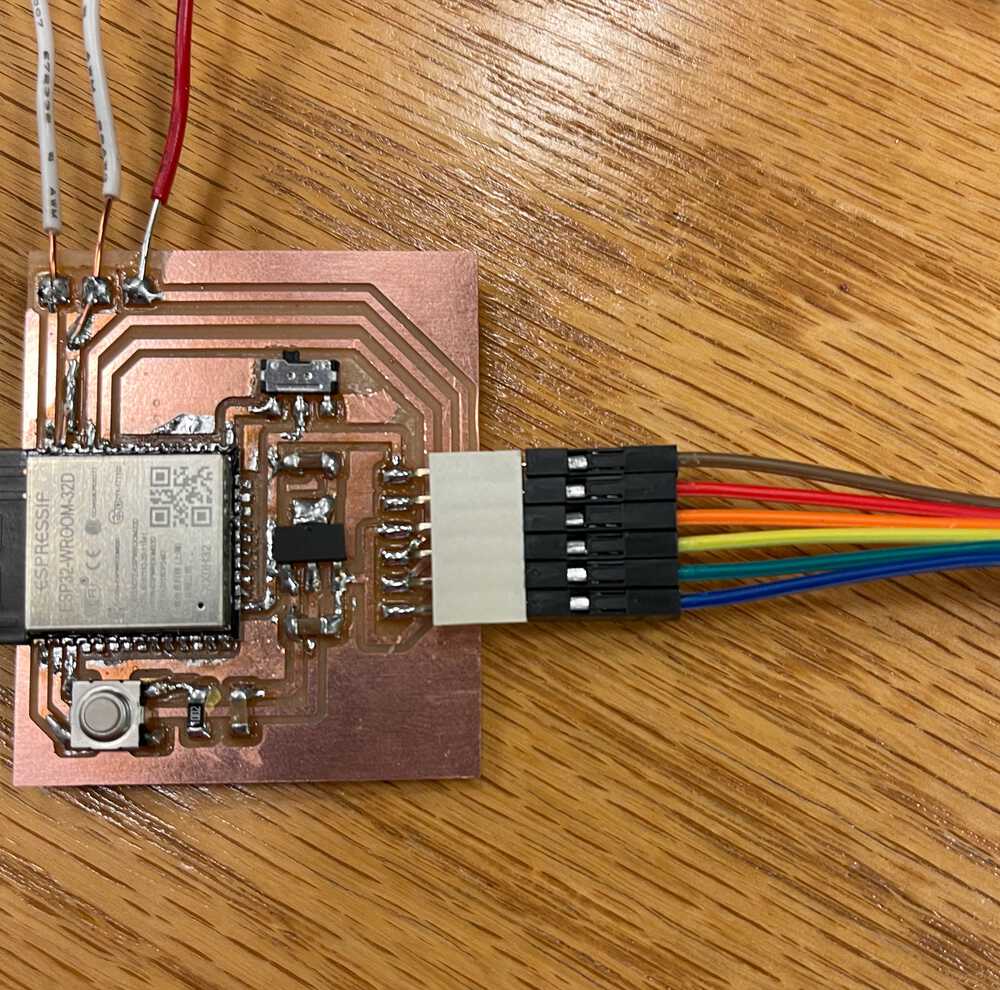
Programming
Programming the board this week I decided to make my LEDs react to the music currently playing on my spotify account and to give my computer control over the lights through bluetooth. To connect to my computer over bluetooth, on the ESP32 I created a service and characteristic that accepted json data from bluetooth communication with the computer (not sure if JSON was really the way to go here, but it worked for my purposes). Then on the computer side I used the Web Bluetooth API which is pretty neat, but is currently in beta, to spin up a quick front end with the ability to communicate over bluetooth. Mechanically, on the computer side, this consisted of requesting available bluetooth devices and filtering the the service I defined on my esp32, connecting to the device's GATT server, getting the service, then getting the characteristic from that. From there, I was able to write a function to write data over bluetooth directly to the characteristic in length 20 chunks which I could concatenate together on the esp32 side.
After getting bluetooth to work, I next set up a simple flask api to communicate with the Spotify api to grab data on the music currently playing on my Spotify account, and set up a loop in the front end to constantly poll for info on the currently playing song and transmit the data over bluetooth to the esp32. In order to create varied patterns I take into acount the bpm, genre, and time signature of the song currently playing. I also set up some buttons in the front end to turn on and off the lights, manually set the pattern, and start the script to make them react to the Spotify songs.
ESP32
I won't go through all the aspects of the code since there is a lot, but I will focus on the bluetooth aspects here. The following images show how this was set up on the ESP32. The first photo shows the instantiation of info regarding the bluetooth service and characteristic. The second photo details setting up the callback of the characteristic to handle receiving information over bluetooth. The third photo shows how everything was set up to use the code in the previous 2 photos.
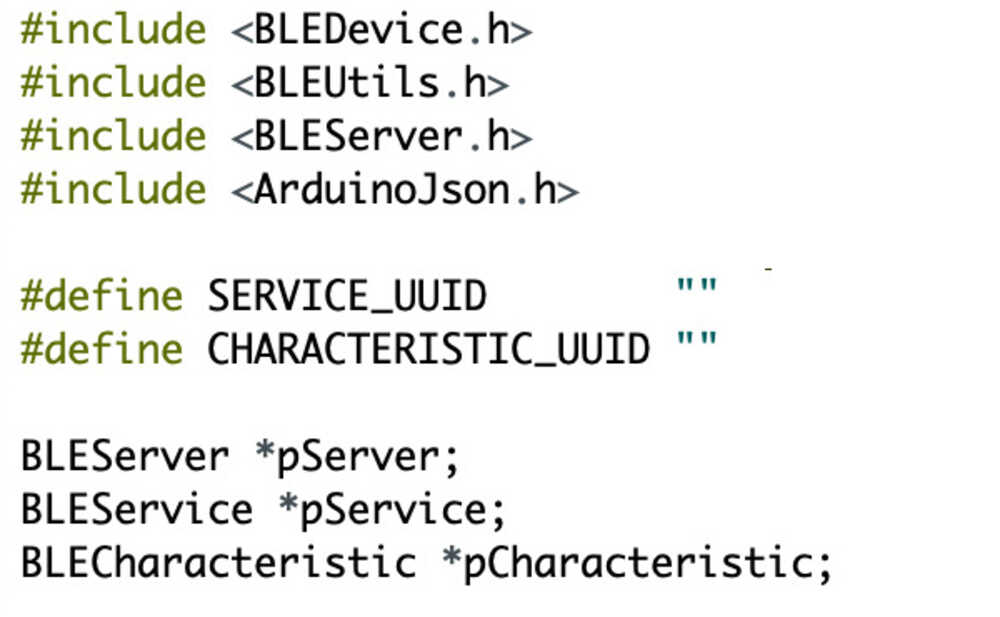
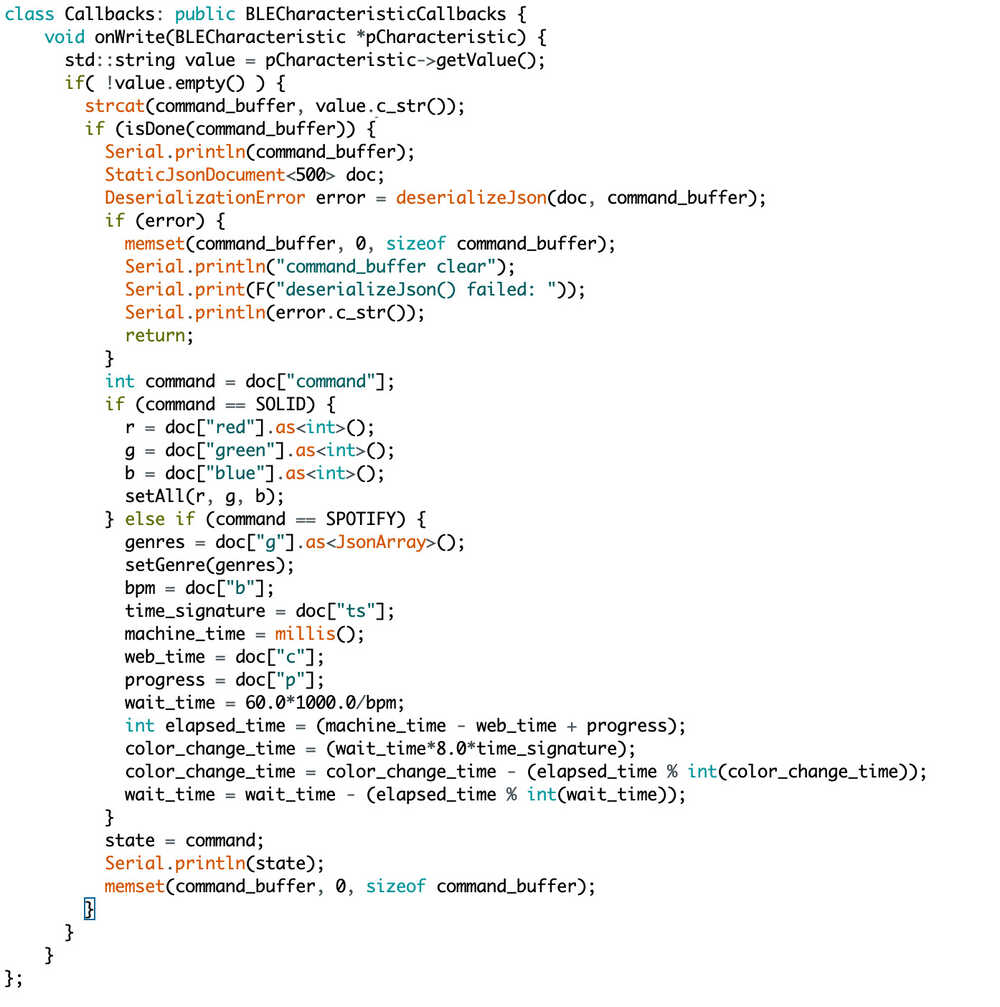
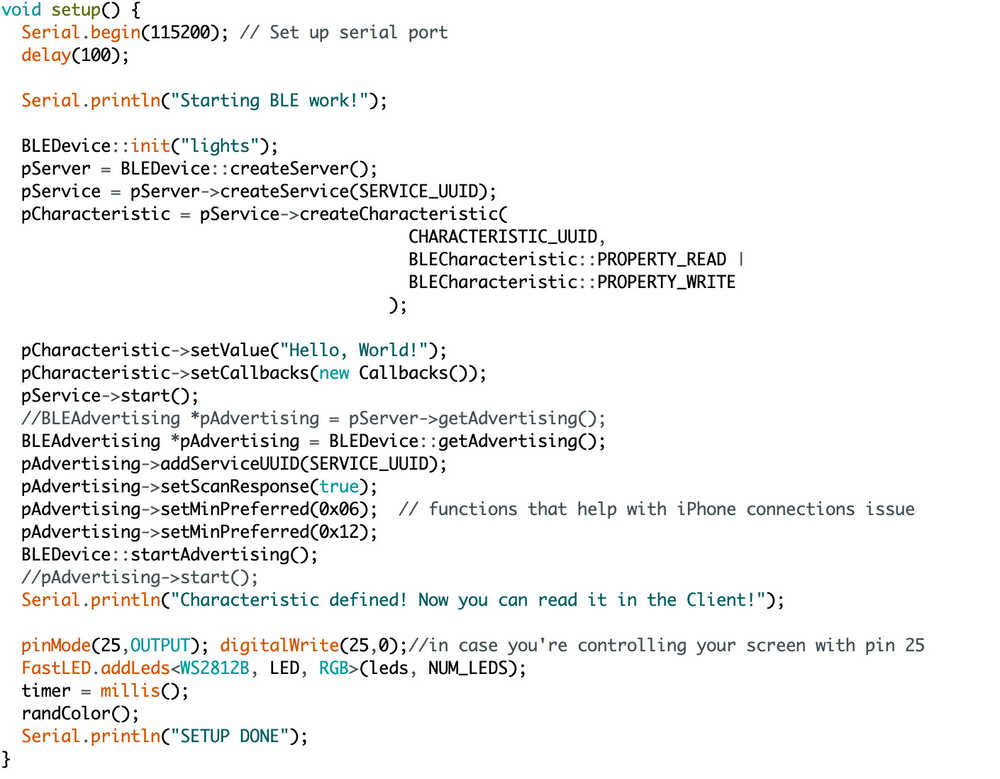
Computer
Once again I won't go through all the details, but rather just focus on the bluetooth side of things. The following pictures show how the code was setup to use the Web Bluetooth Api on the computer side. The first photo shows the code to request bluetooth devices. I filtered based off the specific service and name. The second photo is the code for connecting and disconnecting to the characteristic. The third photo shows how I handled sending data to the characteristic over bluetooth in packets.
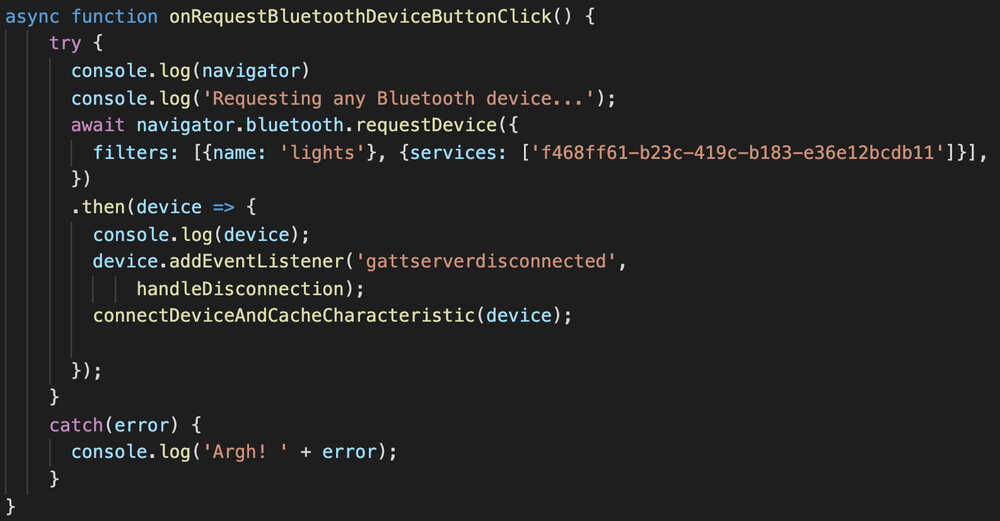
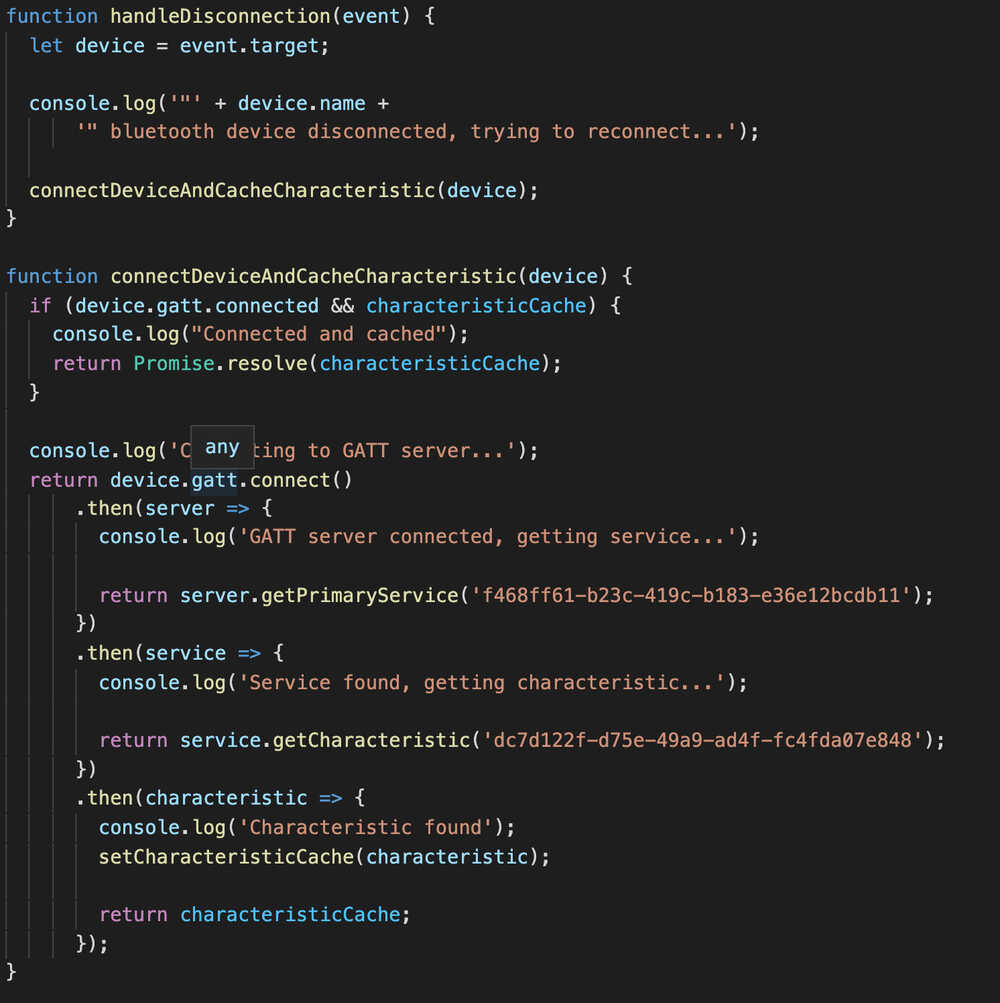
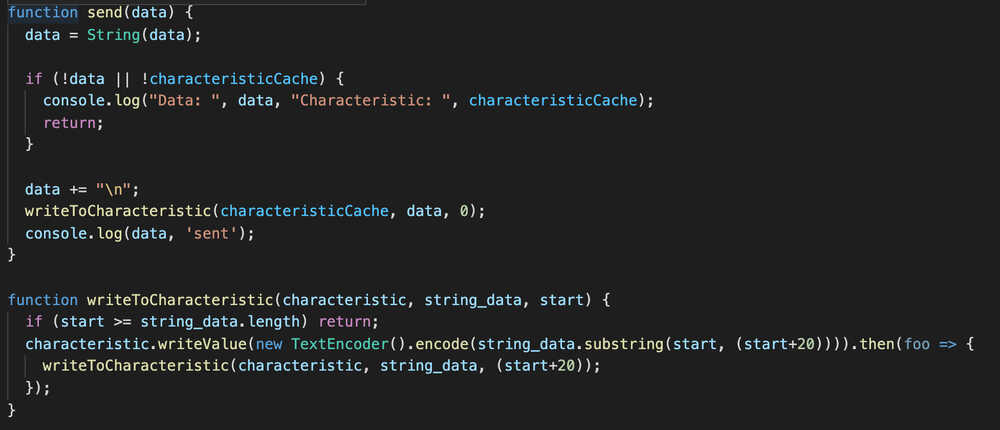
Results
After successfully communicating with bluetooth I played around with the spotify API and got everything behaving nicely!