14. November 2022
Output Devices
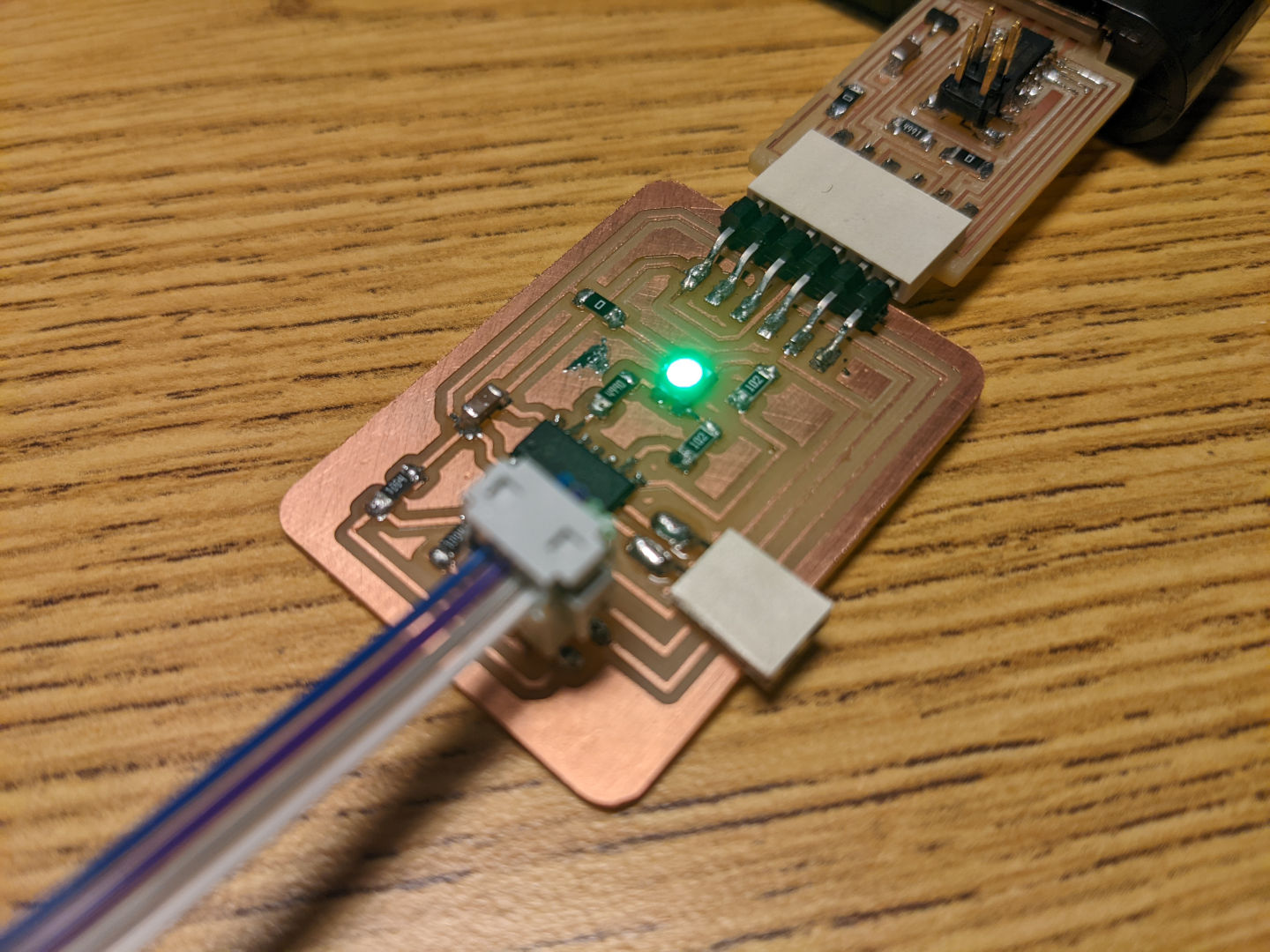
This week’s assignment was to add an output device to a microcontroller board.
Design
I decided to use the capacitive touch sensor I designed last week to control an RGB LED. As such, I redesigned my board from last week to include the RGB LED; I made sure to have the cathodes of the LED connect to PWM pins of the microcontroller.
I then routed the paths in a similar fashion to my other board.
Milling
After designing my board, I milled it.
Stuffing
I then stuffed my board; shown are my board from this week, as well as the parts I made last week.
I added a connector to the VCC of the serial-UPDI board so I could supply power and program my boards from my own computer.
Programming
Finally, I programmed my board so that the LED is
- Blue when the sensor is pressed too gently
- Green when the sensor is pressed with just the right amount of force
- Red when the sensor is pressed too hard
1#define analog_pin PIN_PA4
2#define tx_pin PIN_PA5
3#define red_pin PIN_PB1
4#define green_pin PIN_PB0
5#define blue_pin PIN_PA3
6int red_thresh = 6000;
7int green_thresh = 7500;
8int blue_thresh = 9000;
9
10long result;
11
12void setup() {
13 pinMode(tx_pin,OUTPUT);
14 Serial.begin(9600);
15
16 pinMode(red_pin,OUTPUT);
17 pinMode(green_pin,OUTPUT);
18 pinMode(blue_pin,OUTPUT);
19
20 digitalWrite(red_pin,HIGH);
21 digitalWrite(green_pin,HIGH);
22 digitalWrite(blue_pin,HIGH);
23}
24
25long tx_rx(){
26 int read_high;
27 int read_low;
28 int diff;
29 long int sum;
30 int N_samples = 100;
31
32 sum = 0;
33 for (int i = 0; i < N_samples; i++){
34 digitalWrite(tx_pin,HIGH);
35 read_high = analogRead(analog_pin);
36 delayMicroseconds(100);
37 digitalWrite(tx_pin,LOW);
38 read_low = analogRead(analog_pin);
39 diff = read_high - read_low;
40 sum += diff;
41}
42 return sum;
43}
44
45void loop() {
46 result = tx_rx();
47 if (result < red_thresh) {
48 digitalWrite(red_pin,LOW);
49 digitalWrite(green_pin,HIGH);
50 digitalWrite(blue_pin,HIGH);
51 } else if (result < green_thresh) {
52 digitalWrite(green_pin,LOW);
53 digitalWrite(blue_pin,HIGH);
54 digitalWrite(red_pin,HIGH);
55 } else if (result < blue_thresh){
56 digitalWrite(blue_pin,LOW);
57 digitalWrite(red_pin,HIGH);
58 digitalWrite(green_pin,HIGH);
59 }
60 Serial.println(result);
61 //delay(100);
62}
Overall, the input and output devices work very well!