User Interfaces with Processing and ESP32
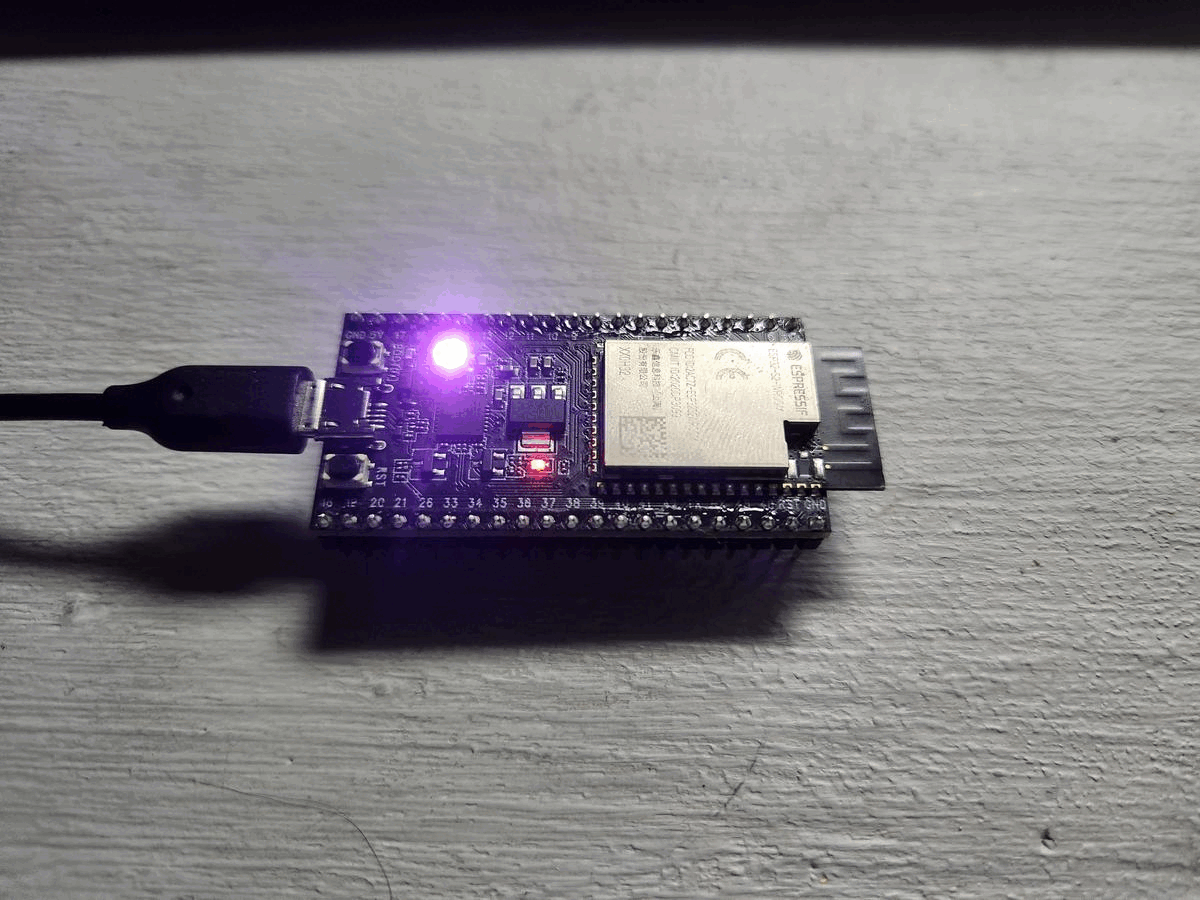
My goal this week was to produce an application that interfaces a user with an input and/or output device that you made. I decided to write an interface controlling a NeoPixel/WS2812 LED.
Follower-Side Code on ESP32
For the device under control, I used the ESP32 development board, which has a single NeoPixel LED onboard. This is indicative of how my NeoPixel bike lights would work, except without the need for an external power supply to drive so many LED’s. I wrote an Arduino program that would listen on the serial port for comma-separated values of the red, green, and blue colors for the lights. An example data packet would be: “128,64,48” corresponding to a red value of 128, green value of 64, and blue value of 48.
The Arduino code for the ESP32 interface is below:
#define LED_PIN 18
#define LED_COUNT 1
Adafruit_NeoPixel strip(LED_COUNT, LED_PIN, NEO_GRB + NEO_KHZ800);
void setup() {
strip.begin(); // INITIALIZE NeoPixel strip object (REQUIRED)
strip.show(); // Turn OFF all pixels ASAP
strip.setBrightness(50); // Set BRIGHTNESS to about 1/5 (max = 255)
Serial.begin(9600);
}
String input = "";
int red, grn, blu;
void loop() {
while (Serial.available() > 0) {
red = Serial.parseInt();
grn = Serial.parseInt();
blu = Serial.parseInt();
char r = Serial.read();
if (r == '\n'){}
if (red > 255) { red = 255; }
if (red < 0) { red = 0; }
if (grn > 255) { grn = 255; }
if (grn < 0) { grn = 0; }
if (blu > 255) { blu = 255; }
if (blu < 0) { blu = 0; }
Serial.print("(");
Serial.print(red);
Serial.print(",");
Serial.print(grn);
Serial.print(",");
Serial.print(blu);
Serial.println(")");
}
strip.setPixelColor(0, strip.Color(red, grn, blu));
strip.show();
delay(250);
}
Leader-side GUI interface in Processing
For the GUI interface that controls the lights, I used Processing, a programming language for making visual interfaces. I used a library, UIBooster, to design a color picker wheel that would then set the desired color. The desired color would be sent over serial in comma-separated value (CSV) format to the ESP32.
The code for the Processing interface is below:
import processing.serial.*;
Serial myPort;
color favoriteColor;
void setup() {
size(0, 0, P3D);
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600);
background(10);
favoriteColor = new UiBooster().showColorPickerAndGetRGB("Choose your favorite color", "Color picking");
}
void draw() {
int redVal = int(red(favoriteColor));
int greenVal = int(green(favoriteColor));
int blueVal = int(blue(favoriteColor));
myPort.write(str(redVal) + "," + str(greenVal) + "," + str(blueVal) + ",");
}