Week 2: Laser-cutting
Link for full Prompt
🙋♀️ HUMAN (TA-Amira)
I want to build a box of size 10 by 10 by 10 cm using a press-fit assembly technique. Can you provide me code to create the DXF file putting into consideration the laser cutting kerf
🤖 GPT-4
Certainly! To create a 10cm x 10cm x 10cm box, you'll need six sides. However, some of these will be identical due to symmetry. Below is the Python code that extends the previous script to include all the needed sides. You'll end up cutting:
- Two of Side 1 (with vertical slots)
- Two of Side 2 (with horizontal slots)
- One of Side 3 (with vertical slots but flipped)
- One of Side 4 (with horizontal slots but flipped)
Here is the extended code:
pythonCopy code import ezdxf def draw_rect(msp, x, y, w, h): msp.add_lwpolyline([(x, y), (x + w, y), (x + w, y + h), (x, y + h), (x, y)]) def draw_cutout(msp, x, y, w, h): draw_rect(msp, x, y, w, h) doc = ezdxf.new() msp = doc.modelspace() box_size = 100 # 10 cm in mm thickness = 3 # 3 mm thickness kerf = 0.2 # 0.2 mm kerf width slot_length = box_size / 4 # Length of the slot slot_width = thickness + kerf # Width of the slot, corrected for kerf # Side 1 with vertical slots draw_rect(msp, 0, 0, box_size, box_size) draw_cutout(msp, 0, box_size / 4, slot_width, slot_length) draw_cutout(msp, 0, 3 * box_size / 4, slot_width, slot_length) draw_cutout(msp, box_size - slot_width, box_size / 4, slot_width, slot_length) draw_cutout(msp, box_size - slot_width, 3 * box_size / 4, slot_width, slot_length) # Side 2 with horizontal slots, offset by 110 in x-direction draw_rect(msp, 110, 0, box_size, box_size) draw_cutout(msp, 110 + box_size / 4, 0, slot_length, slot_width) draw_cutout(msp, 110 + 3 * box_size / 4, 0, slot_length, slot_width) draw_cutout(msp, 110 + box_size / 4, box_size - slot_width, slot_length, slot_width) draw_cutout(msp, 110 + 3 * box_size / 4, box_size - slot_width, slot_length, slot_width) # Side 3 (flipped vertical slots), offset by 220 in x-direction draw_rect(msp, 220, 0, box_size, box_size) draw_cutout(msp, 220, box_size / 4, slot_width, slot_length) draw_cutout(msp, 220, 3 * box_size / 4, slot_width, slot_length) draw_cutout(msp, 220 + box_size - slot_width, box_size / 4, slot_width, slot_length) draw_cutout(msp, 220 + box_size - slot_width, 3 * box_size / 4, slot_width, slot_length) # Side 4 (flipped horizontal slots), offset by 330 in x-direction draw_rect(msp, 330, 0, box_size, box_size) draw_cutout(msp, 330 + box_size / 4, 0, slot_length, slot_width) draw_cutout(msp, 330 + 3 * box_size / 4, 0, slot_length, slot_width) draw_cutout(msp, 330 + box_size / 4, box_size - slot_width, slot_length, slot_width) draw_cutout(msp, 330 + 3 * box_size / 4, box_size - slot_width, slot_length, slot_width) # Save DXF drawing doc.saveas("complete_box_design.dxf")
In this code, each "Side" consists of a square face and slots that are "cut out." The slots are drawn as separate closed paths. Depending on how your laser cutter interprets these, they should appear as areas that are not cut.
You'll need to cut two pieces each of "Side 1" and "Side 2" and one piece each of "Side 3" and "Side 4" to assemble a complete box. The code uses an offset in the x-direction to position each side next to the previous one. After cutting, you can assemble these pieces using the slots and tabs.
Feel free to adjust the kerf, thickness, or other parameters as needed for your specific material and laser cutter.
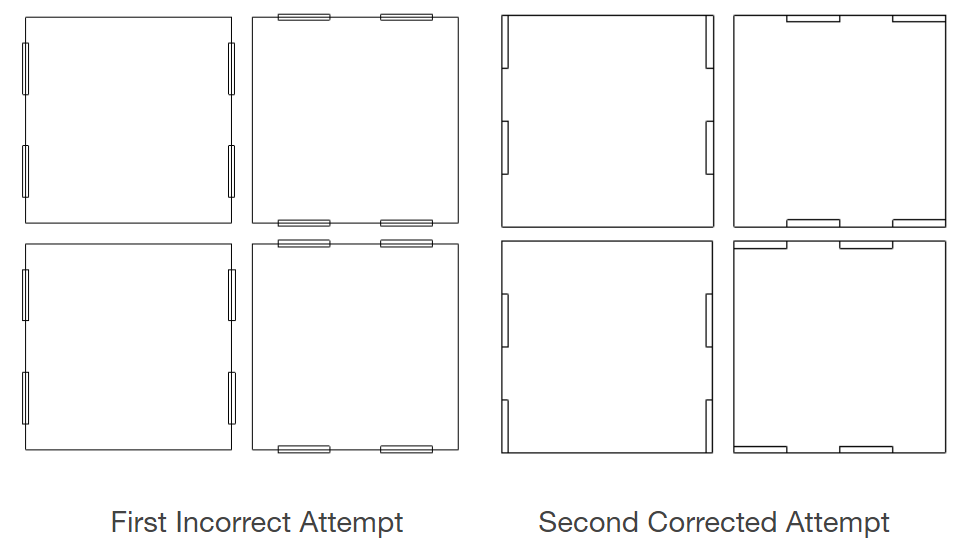