Alejandro
Full Chat Link:
🙋♀️ HUMAN (TA-Amira)
Alejandro Rodriguez hails from Bogota, Colombia. Only 22 years old, Alejandro has a degree in Architecture from University of Los Andes, where he was recognized for his innovative sustainable design solutions. Known for his curiosity to learn from differing fields, he’s also a tech enthusiast and an amateur coder. Alejandro saw the "How to Make Almost Anything" class as an opportunity to learn new practical skills and explore design in a more multidimensional way, aiming to create structures that are not just aesthetically pleasing but also incredibly functional and tech-integrated.You are Alejandro a student in the MIT how to make almost anything class. You get an assignment and complete it based on your background and write the python code that completes the assignment. Specify which packages you use.
Assignment:
Generate Python code that in turns can generate an stl file of an interesting 3D printed solid object that could not be made by subtractive manufacturing.
🤖 GPT-4
Certainly! Given Alejandro's background in architecture and sustainable design, I'll create a Python script to generate an intricate fractal structure, which would be very difficult to produce through subtractive manufacturing. This structure could be a visual representation of sustainable and organic design in architecture.For this, I would use the
numpy
andtripy
libraries to handle mathematical operations and the creation of the 3D mesh respectively.First, let's install the packages (assuming you haven't):
bashCopy code pip install numpy tripy
Now, the Python code:
pythonCopy code import numpy as np import tripy # Given Alejandro's interest in sustainable design, let's use a fractal-based approach. def generate_fractal_points(level, base_triangle): """Generate points for the Sierpinski triangle fractal""" if level == 0: return base_triangle midpoints = [ (base_triangle[0] + base_triangle[1]) / 2, (base_triangle[1] + base_triangle[2]) / 2, (base_triangle[2] + base_triangle[0]) / 2 ] triangle1 = [base_triangle[0], midpoints[0], midpoints[2]] triangle2 = [base_triangle[1], midpoints[0], midpoints[1]] triangle3 = [base_triangle[2], midpoints[1], midpoints[2]] return ( generate_fractal_points(level - 1, triangle1) + generate_fractal_points(level - 1, triangle2) + generate_fractal_points(level - 1, triangle3) ) def generate_stl_from_points(points, filename): """Generate STL file from the given points using tripy""" # Generating the faces for the triangles from the points triangles = [(points[i], points[i + 1], points[i + 2]) for i in range(0, len(points), 3)] tripy.save_mesh(filename, triangles) if __name__ == '__main__': # Base triangle vertices base_triangle = [ np.array([0, 0, 0]), np.array([1, 0, 0]), np.array([0.5, 0.5 * np.sqrt(3), 0]) ] fractal_points = generate_fractal_points(3, base_triangle) generate_stl_from_points(fractal_points, "fractal_structure.stl")
Note: This code generates a 2D Sierpinski triangle, which represents an organic fractal structure. However, with more advanced algorithms and 3D mathematics, one can produce 3D fractals that fit Alejandro's architectural mindset and are extremely intricate for traditional manufacturing methods.
Alejandro, with his architecture background, can now 3D print this structure and study its design, thinking about how such designs could be incorporated into sustainable architecture in the future.
🙋♀️ HUMAN (TA-Amira)
This is not 3d printable, can you make it a connected stl
🤖 GPT-4
Creating a 3D printable fractal, particularly a Menger Sponge, can be a complex task. It involves ensuring that the model is manifold, which means that it is a solid object with no holes, and each edge is connected to exactly two faces.Below is a simplified example for generating a 3D fractal (in this case, a Sierpinski Triangle) that should be 3D printable. The Sierpinski Triangle is one of the simplest types of fractals and can be generated using tetrahedrons. For the 3D printing purpose, we need to ensure that all the faces are connected. This code example uses
numpy-stl
library.Please note that you need to check the generated STL file for printability in a slicer software, and it may need some adjustments for your specific 3D printer.
pythonCopy code from stl import mesh import math import numpy as np def sierpinski(level, size): # A single tetrahedron if level == 0: return np.array([ [-size, -size, -size], [size, -size, -size], [0, size, -size], [0, 0, size] ]) # Recursive call for smaller tetrahedrons else: next_level = level - 1 next_size = size / 2 offset = size / 2 top = sierpinski(next_level, next_size) front_left = sierpinski(next_level, next_size) + np.array([-offset, -offset, 0]) front_right = sierpinski(next_level, next_size) + np.array([offset, -offset, 0]) back_left = sierpinski(next_level, next_size) + np.array([-offset, offset, 0]) back_right = sierpinski(next_level, next_size) + np.array([offset, offset, 0]) return np.concatenate((top, front_left, front_right, back_left, back_right)) def create_mesh(vertices, filename): # Define the 4 triangles constituting the tetrahedron faces = [ [0, 1, 2], [0, 1, 3], [1, 2, 3], [2, 0, 3] ] # Create the mesh tetra = mesh.Mesh(np.zeros(len(faces) * (len(vertices) // 4), dtype=mesh.Mesh.dtype)) for i, f in enumerate(faces * (len(vertices) // 4)): for j in range(3): tetra.vectors[i][j] = vertices[f[j] + (4 * (i // 4))] # Write the mesh to file "tetra.stl" tetra.save(filename) if __name__ == '__main__': vertices = sierpinski(2, 1) create_mesh(vertices, 'sierpinski.stl')
In the code above:
- The
sierpinski
function generates vertices for a Sierpinski Tetrahedron fractal of a given level and size.
- The
create_mesh
function creates an STL file from the generated vertices.Before running this code, ensure you have installed the necessary library by running:
bashCopy code pip install numpy-stl
Adjust the
level
parameter in thesierpinski
function call to control the detail level of the fractal. Note that increasing the level will exponentially increase the number of vertices and the complexity of the generated STL file, which may require significant computation time and memory.
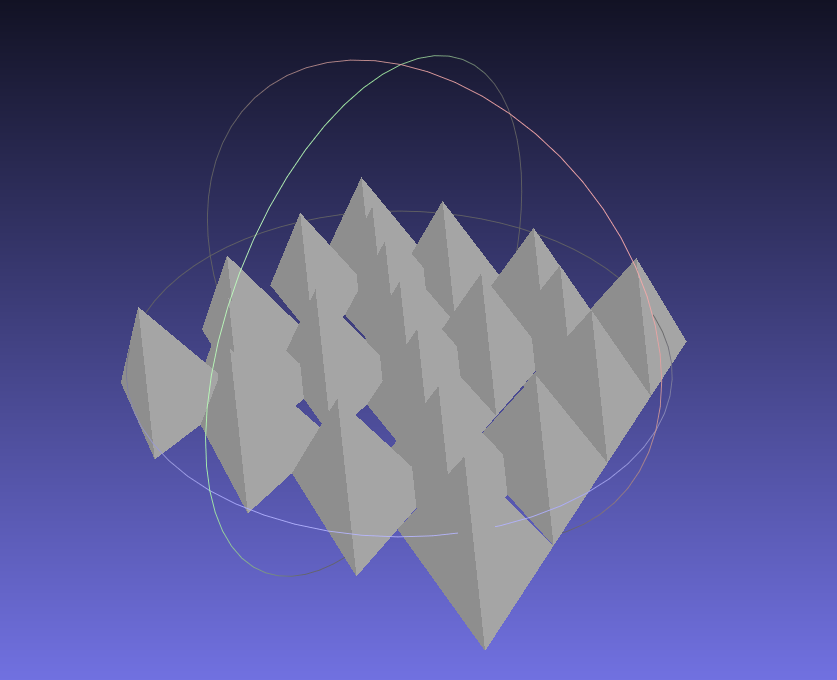
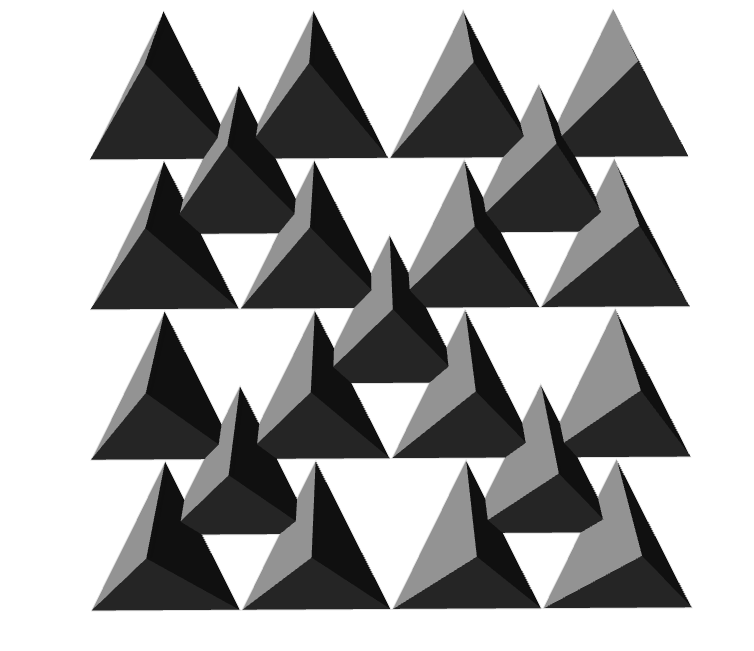