No personality
🙋♀️ HUMAN (TA-Amira)
Can you generate G-code to 3d print a gyroid that is 5cm by 5cm by 5cm
🤖 GPT-4
G-code generation is complex and it is heavily dependent on the specifics of the 3D printer being used, the material properties, the specific layer thickness, the nozzle size, print speed, and more. Hence, it’s a process that should be done using a slicing software.
🙋♀️ HUMAN (TA-Amira)
Can you generate an .stl mesh file of a gyroid that is 5cm by 5cm by 5cm using python
🤖 GPT-4
Certainly! One way to generate a gyroid mesh in the form of an STL file using Python is by utilizing thenumpy
andtripy
libraries. Here's how you can do it:
- First, you'll need to install the necessary libraries:
Copy code pip install numpy tripy
- Then, use the following Python code to generate an STL of a gyroid with the specified dimensions:
pythonCopy code import numpy as np import tripy def gyroid_function(x, y, z, wavelength=1.0, intensity=1.0): s1 = np.sin(2 * np.pi * x / wavelength) * np.cos(2 * np.pi * y / wavelength) s2 = np.sin(2 * np.pi * y / wavelength) * np.cos(2 * np.pi * z / wavelength) s3 = np.sin(2 * np.pi * z / wavelength) * np.cos(2 * np.pi * x / wavelength) return intensity * (s1 + s2 + s3) def generate_gyroid_stl(filename, size=5.0, resolution=0.1, threshold=0.0, wavelength=2.0): # Create a grid of points x = np.arange(0, size, resolution) y = np.arange(0, size, resolution) z = np.arange(0, size, resolution) X, Y, Z = np.meshgrid(x, y, z) # Evaluate the gyroid function at each point V = gyroid_function(X, Y, Z, wavelength) # Extract the isosurface using the marching cubes algorithm verts, faces, _, _ = tripy.marching_cubes(V, threshold, spacing=(resolution, resolution, resolution)) # Write the result to an STL file tripy.save_mesh(filename, verts, faces) generate_gyroid_stl("gyroid.stl", size=5.0, resolution=0.1, threshold=0.0, wavelength=2.0)
The above code defines the gyroid function and then generates a mesh representation using the marching cubes algorithm. The mesh is then saved as an STL file. Adjust the
resolution
andwavelength
parameters to change the quality and appearance of the gyroid, respectively.Do note that generating the gyroid at high resolutions may require significant memory and computational resources.
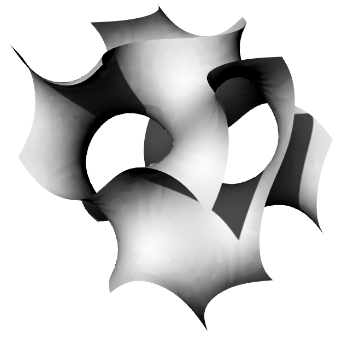
🙋♀️ HUMAN (TA-Amira)
can the gyorid be a solid closed mesh
🤖 GPT-4
Creating a closed mesh for a gyroid pattern can be complex due to its intricate, continuous structure. A simple thresholding technique such as marching cubes may not create a closed mesh right away. Additional mesh operations may be needed to ensure that the resulting structure is manifold (i.e., a "solid" 3D object without holes).You might want to approach this by first generating the gyroid pattern in a volume and then removing a margin around the edges to make the structure "solid." This way, the generated pattern will not touch the borders of the bounding box, effectively creating a closed mesh.
Here's how you could modify the Python code to create a more "closed" gyroid mesh:
pythonCopy code import numpy as np from skimage import measure from stl import mesh def gyroid(x, y, z, scale=1.0): return np.sin(x*scale) * np.cos(y*scale) + np.sin(y*scale) * np.cos(z*scale) + np.sin(z*scale) * np.cos(x*scale) # Create a 3D grid n = 50 # Resolution, increase for more details, but will be slower margin = 5 # Margin percentage to make the structure closed x = np.linspace(0, 5, n) y = np.linspace(0, 5, n) z = np.linspace(0, 5, n) X, Y, Z = np.meshgrid(x, y, z) # Calculate gyroid values at each point in the 3D grid vol = gyroid(X, Y, Z, scale=np.pi/2.5) # Mask the volume to make sure the gyroid structure is "closed" mask_margin = int(n * margin / 100) vol[:mask_margin, :, :] = -1 vol[-mask_margin:, :, :] = -1 vol[:, :mask_margin, :] = -1 vol[:, -mask_margin:, :] = -1 vol[:, :, :mask_margin] = -1 vol[:, :, -mask_margin:] = -1 # Extract the 0-level isosurface from the 3D volume verts, faces, _, _ = measure.marching_cubes(vol, 0, spacing=(5/n, 5/n, 5/n)) # Create the mesh gyroid_mesh = mesh.Mesh(np.zeros(faces.shape[0], dtype=mesh.Mesh.dtype)) for i, f in enumerate(faces): for j in range(3): gyroid_mesh.vectors[i][j] = verts[f[j], :] # Write the mesh to file gyroid_mesh.save('closed_gyroid.stl')
This modified code includes a
margin
to make the gyroid structure "closed." You can adjust themargin
percentage according to your needs.Remember to install the required Python packages if you haven't done so:
bashCopy code pip install numpy scikit-image numpy-stl
After running this code, you should get a more "closed" gyroid structure saved as
closed_gyroid.stl
.
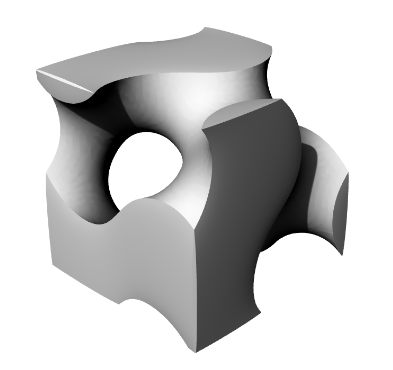
🙋♀️ HUMAN (TA-Amira)
Task: generate python code based on the following rules. Input: the mesh from the previous code block and all hardware-related parameters that
slic3r
accepts. Output: the g-code generated based on the mesh. Constraints: use the command-line toolslic3r
and usesubprocess
to call it. assumeslic3r
can be directly called by theslic3r
command. we use python3 here.
🤖 GPT-4
To accomplish this task, you can use Python's
subprocess
module to run Slic3r from the command line, passing it the STL file and any hardware-related parameters it needs. Below is an example Python code snippet that demonstrates how this might work:Firstly, ensure you have the required Python package:
bashCopy code pip install subprocess.run
Here's the Python code:
pythonCopy code import subprocess # Function to generate G-code from STL file using Slic3r def generate_gcode(stl_file, output_gcode, **slic3r_params): # Basic Slic3r command for generating G-code from an STL file cmd = ["slic3r", "--load", stl_file, "--output", output_gcode] # Add hardware-related parameters to the Slic3r command for key, value in slic3r_params.items(): cmd.append("--{} {}".format(key, value)) # Execute the command subprocess.run(cmd) # Generate the gyroid mesh here (this would be your previous code block) # ... # Define the STL and output G-code filenames stl_file = "closed_gyroid.stl" output_gcode = "gyroid.gcode" # Define hardware-related parameters (replace these with your actual parameters) slic3r_params = { 'nozzle-diameter': 0.4, 'layer-height': 0.2, 'fill-density': 20, 'print-speed': 60, # Add other Slic3r parameters here as needed } # Generate the G-code generate_gcode(stl_file, output_gcode, **slic3r_params)
Replace the values in
slic3r_params
with those that apply to your specific hardware setup. The command-line tool for Slic3r has numerous options, and you can find the complete list in Slic3r's manual.In the example,
stl_file
is the name of your input STL file (assumed to be"closed_gyroid.stl"
), andoutput_gcode
is the name of the G-code file to be generated (assumed to be"gyroid.gcode"
). The functiongenerate_gcode()
will create a G-code file based on the provided STL file and hardware parameters.Run this code after running your previous mesh generation code, and it should generate the G-code file you need.
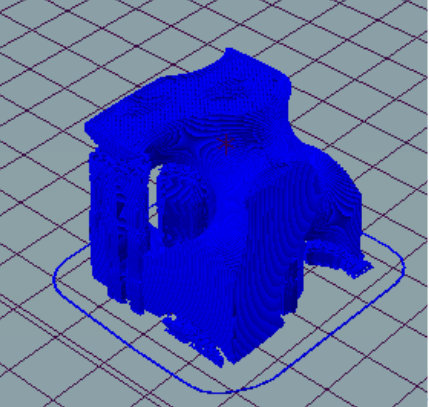