Embedded Programming
I decided to code a program for my final project, which would be moving towards the right amount of light (phototaxis). Looking at the options given, I felt that a Raspberry Pi 2040 board would be the best option, as I would have a lot of peripherals to add to my system. Once I finished reading the documentation, I went to Wokwi to simulate a program. I also approximated a light sensor as a potentiometer as I felt they would be similar in terms of reading voltage. The potentiometer reading is between 0 and 1023. The LEDS are to easily show which mode it is in.
When there is the right amount of light (when the potentiometer reading is between 488 and 536), the isopod will not move. The stepper motor does not spin, and the green light is on.
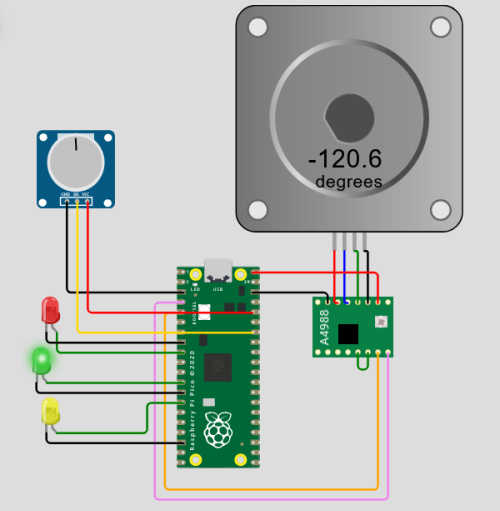
If there is not enough light (the potentiometer is less than 488), the isopod will move towards it. The stepper motor spins as to move towards light, and the yellow light is on.
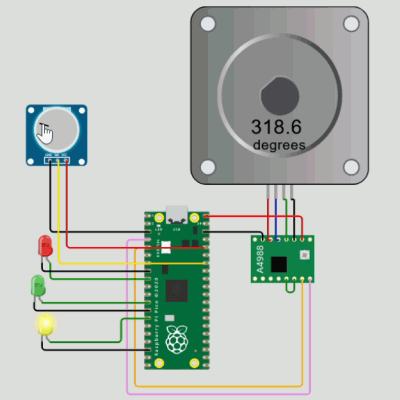
If there is too much light (the potentiometer is more than 536), the isopod will move away from it by spinning the stepper motor in the other direction and the red light turns on.
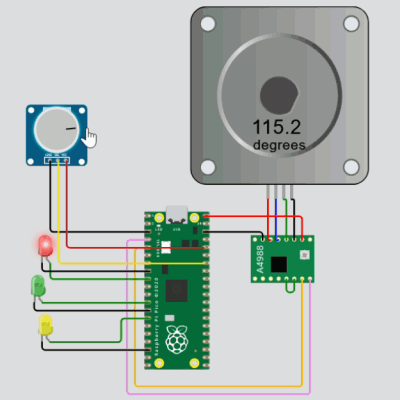
Pico Code
// Raspberry Pi Pico + Stepper Motor Example
#define DIR_PIN 2
#define STEP_PIN 3
#define LIGHT 28
#define GREEN 9
#define RED 6
#define YELLOW 10
void setup() {
pinMode(STEP_PIN, OUTPUT);
pinMode(DIR_PIN, OUTPUT);
digitalWrite(STEP_PIN, LOW);
pinMode(LIGHT, INPUT);
pinMode(GREEN, OUTPUT);
pinMode(RED, OUTPUT);
pinMode(YELLOW, OUTPUT);
}
void loop() {
// Move 200 steps (one rotation) CW over one second
int val = analogRead(LIGHT); // reads the value of the potentiometer (value between 0 and 1023)
Serial.println(val);
if(val > 536){
digitalWrite(GREEN, LOW);
digitalWrite(YELLOW, LOW);
digitalWrite(RED, HIGH);
digitalWrite(DIR_PIN, HIGH);
digitalWrite(STEP_PIN, HIGH);
digitalWrite(STEP_PIN, LOW);
delay(5); // 5 ms * 200 = 1 second
}
else if(val < 488){
digitalWrite(GREEN, LOW);
digitalWrite(YELLOW, HIGH);
digitalWrite(RED, LOW);
digitalWrite(DIR_PIN, LOW);
}
else{
digitalWrite(GREEN, HIGH);
digitalWrite(YELLOW, LOW);
digitalWrite(RED, LOW);
}
}