Output Devices
For this week, I decided to do part of my final project by being able to control a motor. I was looking at a couple of different motors, and decided on a stepper motor. I wanted to use an RP2040, so I designed a board with a Xiao (based on the example board in class) that would use the DRV8428 to control the motor.
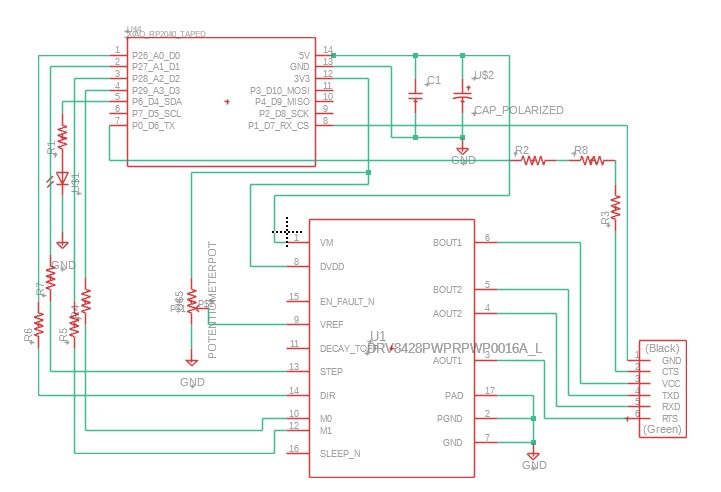
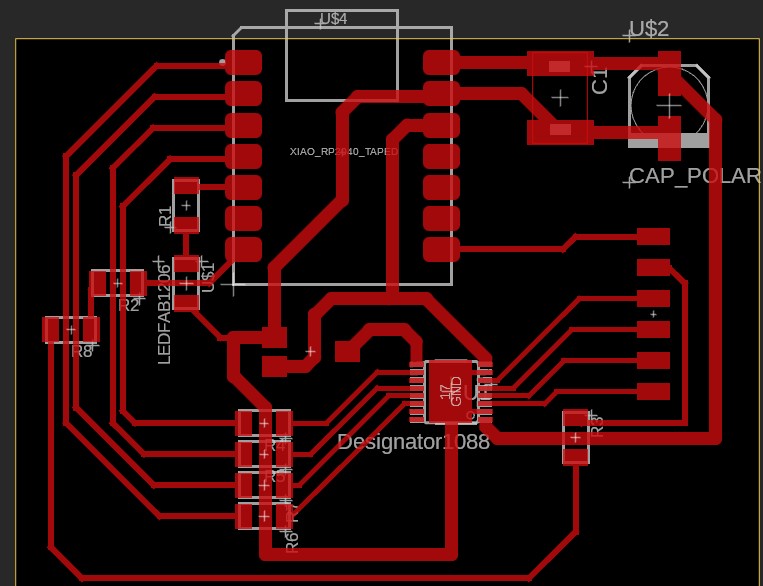
I then milled it out (the small traces needed an engraving bit). The first board turned out not great, as some of the pads were connected that were definitely not supposed to be, and the 1/32" end mill was dull. It is possible that the wires have too wide of a clearance which made the program think it was all one piece.
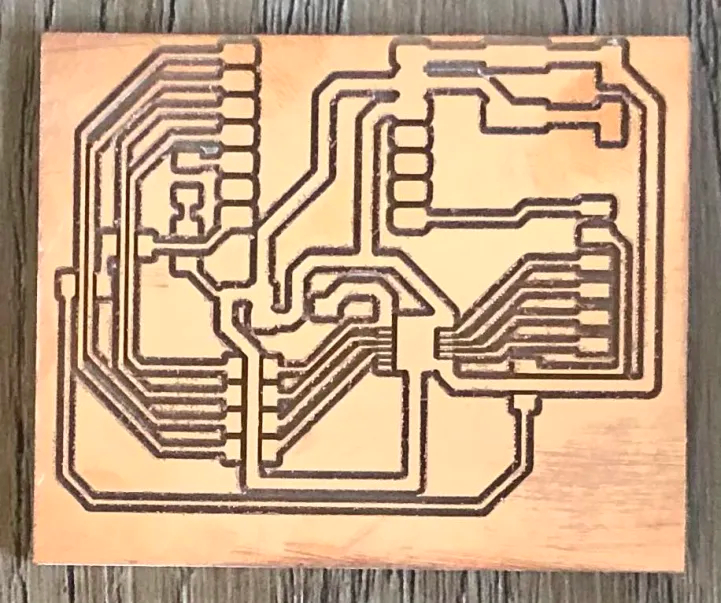
After switching to gerber files on the bamtam, I was able to get the pads separated, but the other 1/32" endmill was also dull, so I sanded the board. I then soldered the components, and adapted the motor test code to work with the RP2040. It... didn't work.
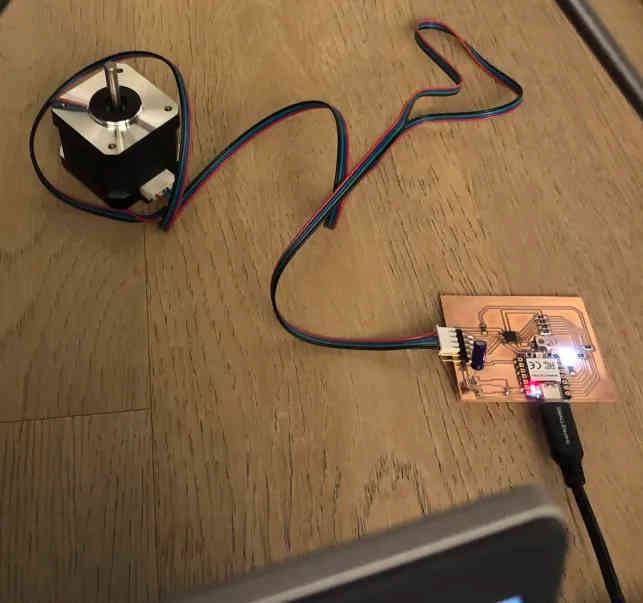
I had not connected the enable and sleep pins to any power, so the DRV chip wouldn't have turned on. After connecting them to a pin on the Xiao, it worked.
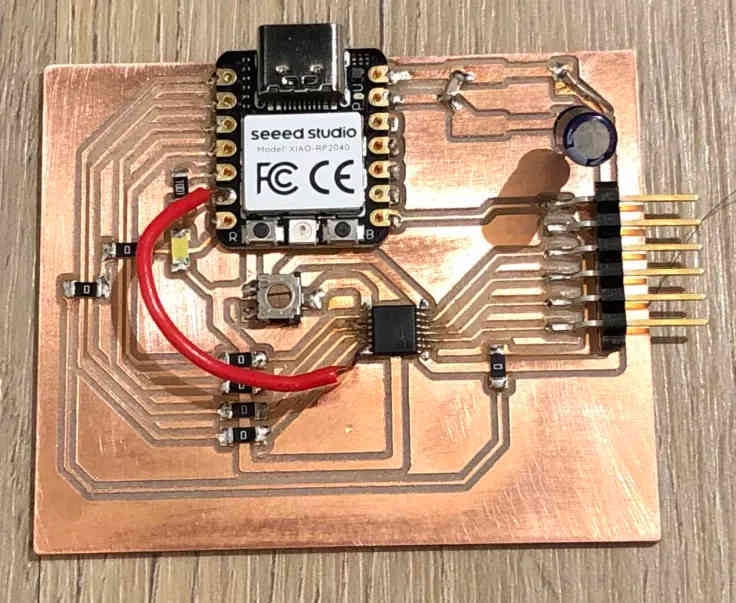
Resources
Code
//
// hello.DRV8428-D11C.ino
//
// DRV8428-D11C stepper hello-world
//
// Neil Gershenfeld 5/30/21
//
// This work may be reproduced, modified, distributed,
// performed, and displayed for any purpose, but must
// acknowledge this project. Copyright is retained and
// must be preserved. The work is provided as is; no
// warranty is provided, and users accept all liability.
//
#define LEDA D4
// #define LEDC 2
#define EN D5
#define DIR D0
#define STEP D1
#define M1 D3
#define M0 D2
#define NSTEPS 1000
#define DELAYHIGH 1000
#define DELAYLOW 1000
#define BLINK 100
void setup() {
pinMode(LEDA,OUTPUT);
pinMode(EN,OUTPUT);
pinMode(STEP,OUTPUT);
pinMode(DIR,OUTPUT);
digitalWrite(LEDA,HIGH);
// digitalWrite(LEDC,LOW);
// pinMode(LEDC,OUTPUT);
digitalWrite(EN,HIGH);
digitalWrite(STEP,LOW);
digitalWrite(DIR,LOW);
}
void blink_off() {
digitalWrite(LEDA,LOW);
delay(BLINK);
digitalWrite(LEDA,HIGH);
}
void do_steps() {
digitalWrite(DIR,HIGH);
for (int i = 0; i < NSTEPS; ++i) {
digitalWrite(STEP,HIGH);
delayMicroseconds(DELAYHIGH);
digitalWrite(STEP,LOW);
delayMicroseconds(DELAYLOW);
}
digitalWrite(DIR,LOW);
for (int i = 0; i < NSTEPS; ++i) {
digitalWrite(STEP,HIGH);
delayMicroseconds(DELAYHIGH);
digitalWrite(STEP,LOW);
delayMicroseconds(DELAYLOW);
}
}
void step_2() {
digitalWrite(M0,HIGH);
digitalWrite(M1,LOW);
pinMode(M0,OUTPUT);
pinMode(M1,OUTPUT);
}
void step_4() {
digitalWrite(M0,LOW);
digitalWrite(M1,HIGH);
pinMode(M0,OUTPUT);
pinMode(M1,OUTPUT);
}
void step_8() {
digitalWrite(M0,HIGH);
digitalWrite(M1,HIGH);
pinMode(M0,OUTPUT);
pinMode(M1,OUTPUT);
}
void step_16() {
digitalWrite(M1,HIGH);
pinMode(M0,INPUT);
pinMode(M1,OUTPUT);
}
void step_32() {
digitalWrite(M0,LOW);
pinMode(M0,OUTPUT);
pinMode(M1,INPUT);
}
void step_128() {
pinMode(M0,INPUT);
pinMode(M1,INPUT);
}
void step_256() {
digitalWrite(M0,HIGH);
pinMode(M0,OUTPUT);
pinMode(M1,INPUT);
}
void loop() {
blink_off();
step_2();
do_steps();
blink_off();
step_4();
do_steps();
blink_off();
step_8();
do_steps();
blink_off();
step_16();
do_steps();
blink_off();
step_32();
do_steps();
blink_off();
step_128();
do_steps();
blink_off();
step_256();
do_steps();
}