Week 12
Networking and Communications
Networking
Assignment: design, build, and connect wired or wireless node(s) with network or bus addresses and local input &/or output device(s)
For this week's assignment, I set up a super simple Flask server. I wrote a quick script server.py to create the server (run with python server.py):
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/message', methods=['GET'])
def message():
return jsonify({"message": "Hello!"})
# Run server
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8080)
This server is part of the networked system, and will be the communication endpoint for a ESP32-c3 node. The server has a network address, making it addressable within the local network. I then set up the ESP32-c3 to connect to wifi, establishing the ESP32-c3 as a node on the network. This way it can communicate with other nodes. The code uploaded onto the ESP32-c3:
#include <WiFi.h>
#include <HTTPClient.h>
const char* ssid = "XXX";
const char* password = "XXX";
const char* serverURL = "XXX";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
void loop() {
if (WiFi.status() == WL_CONNECTED) {
HTTPClient http;
http.begin(serverURL);
int httpCode = http.GET();
if (httpCode > 0) {
String payload = http.getString();
Serial.println("Response:");
Serial.println(payload);
} else {
Serial.println("Error on HTTP request");
}
http.end();
}
delay(10000); // Wait 10 seconds between requests
}
With this code, the esp32-c3 connects to the wifi network, and then sends a GET request to the server every 10 seconds. The response from the server is printed to the serial monitor (a local output device). You can see below that this works:
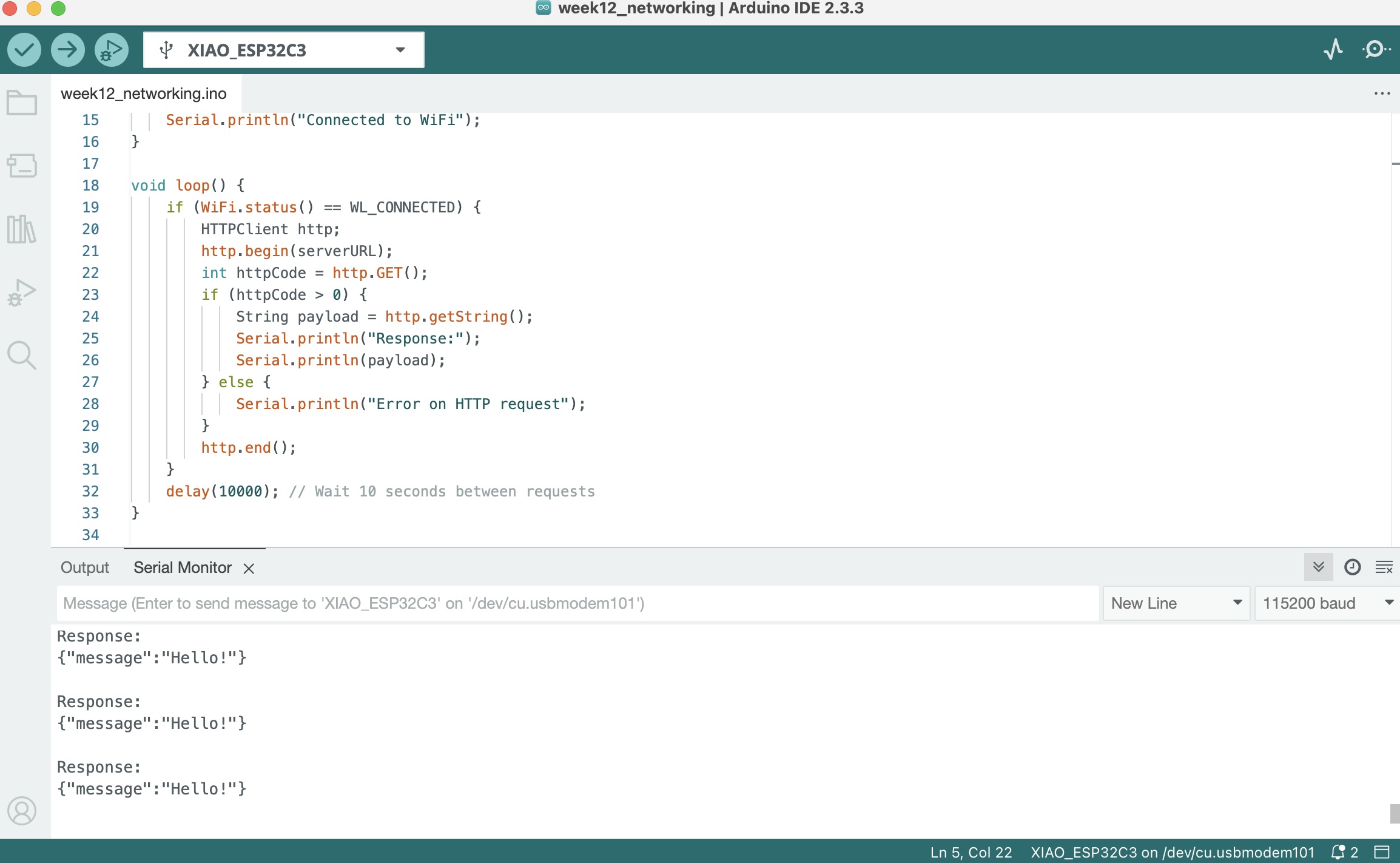
When I stop the server, I correctly get an error message: "Error on HTTP request".