Week 6: How to make an evil project - Tue, Oct 15, 2024
This week our assignment was to mill out the boards we designed last week. I opted to mill out the simple timing game board I designed. I first tried to download my gerber files directly from PCB-SVG, but it did not rotate each LED correctly. I had to download it as a Kicad file, open it in Kicad, and then download the gerber files from Kicad. With this small hiccup solved, I could then let the mill do the heavy lifting of milling my board.
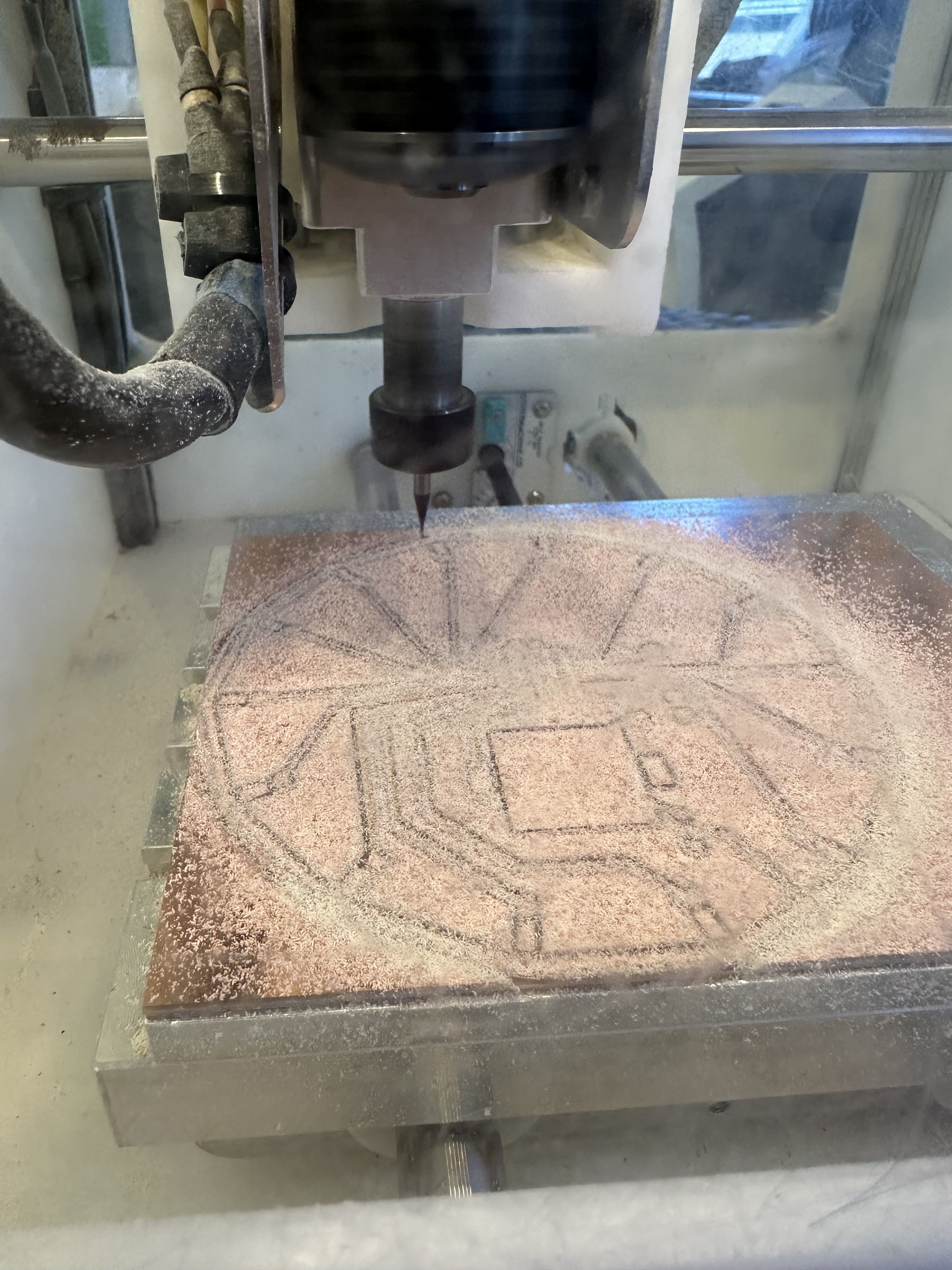
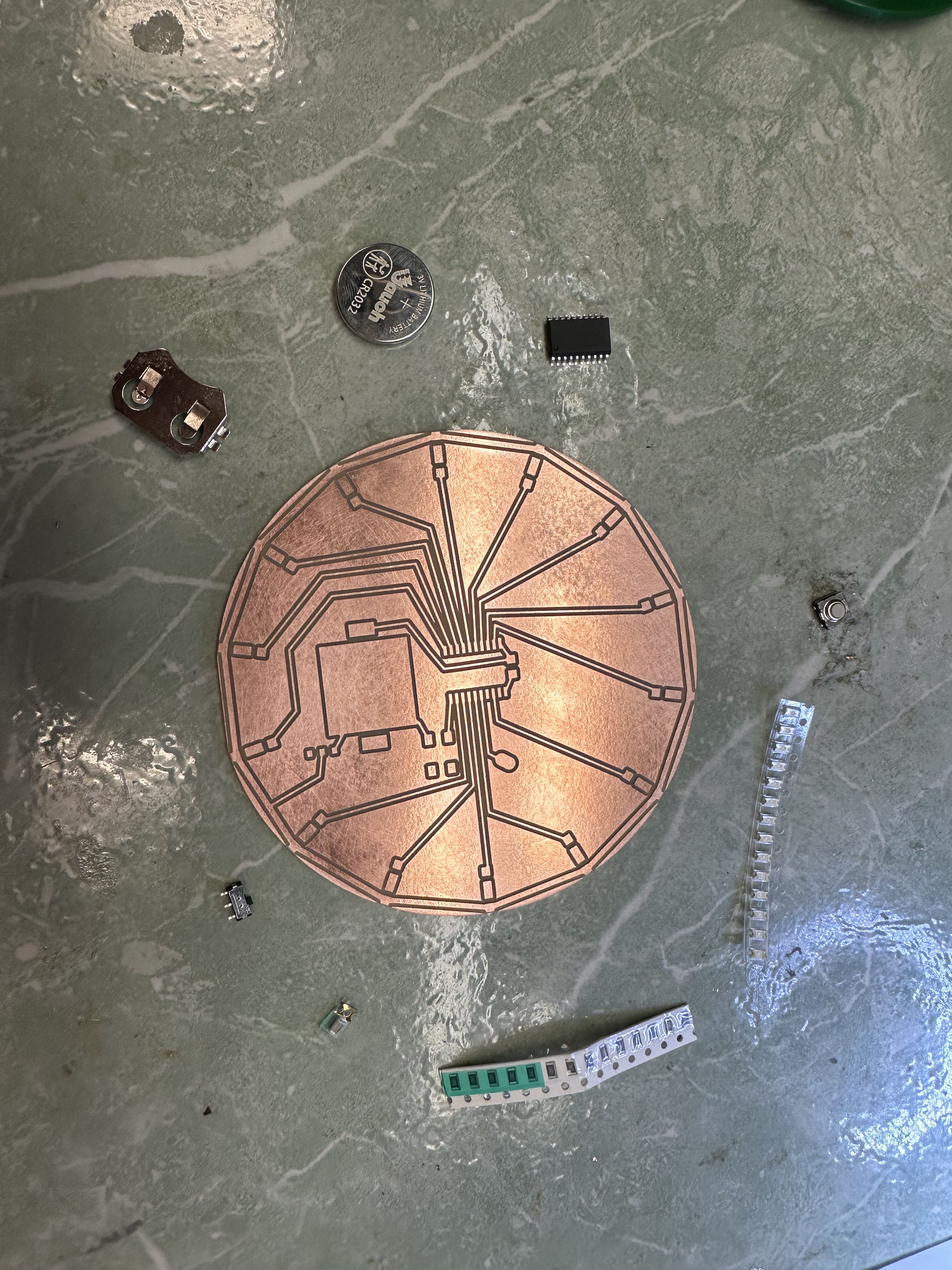
I did forget to connect the ground pin of the ATtiny3226 to ground, but this was an easy fix as I only needed to short the ground pin to one of the large unconnect copper sections and then short this copper section to the circular ground track on the edge of the board.
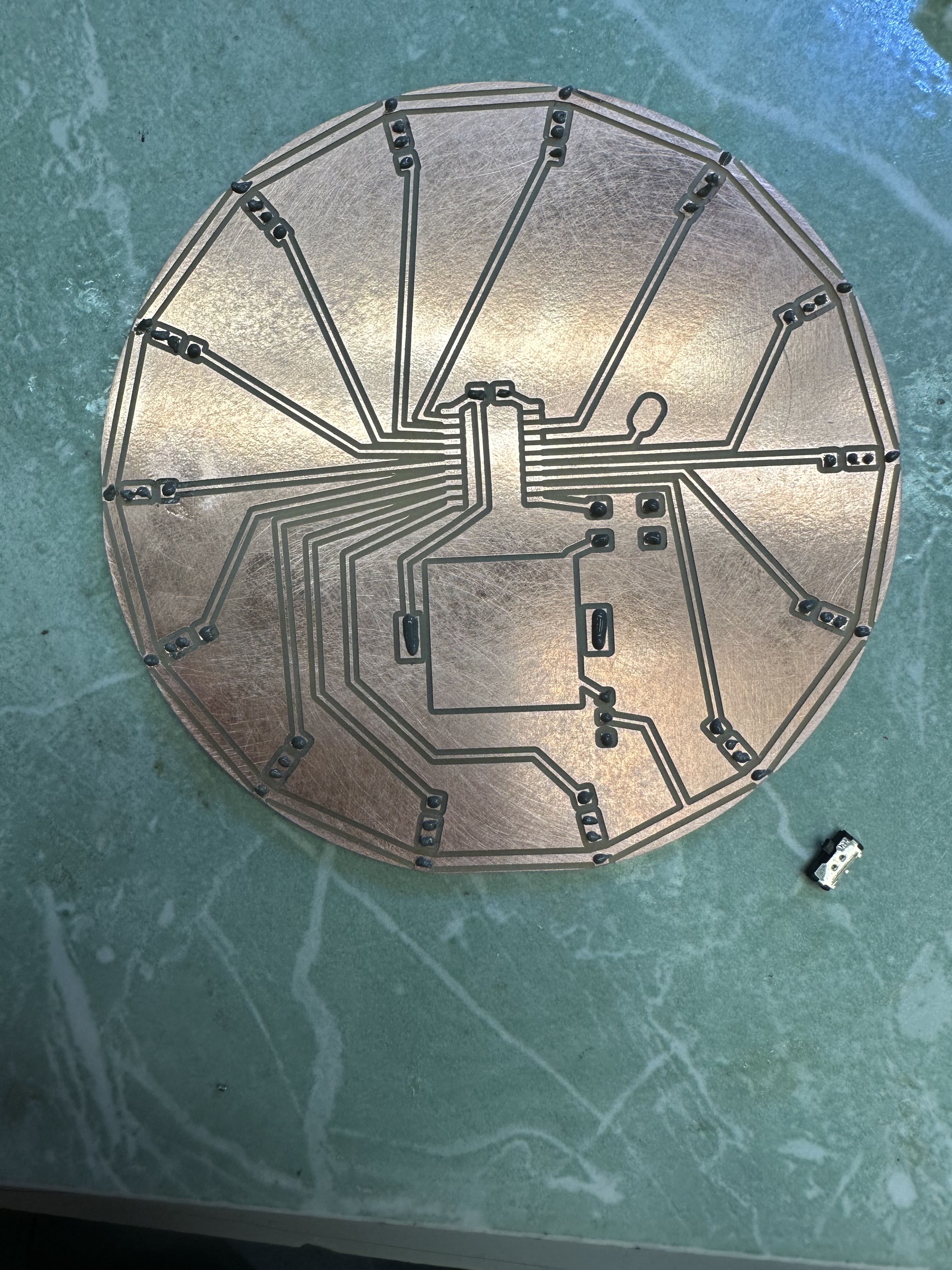
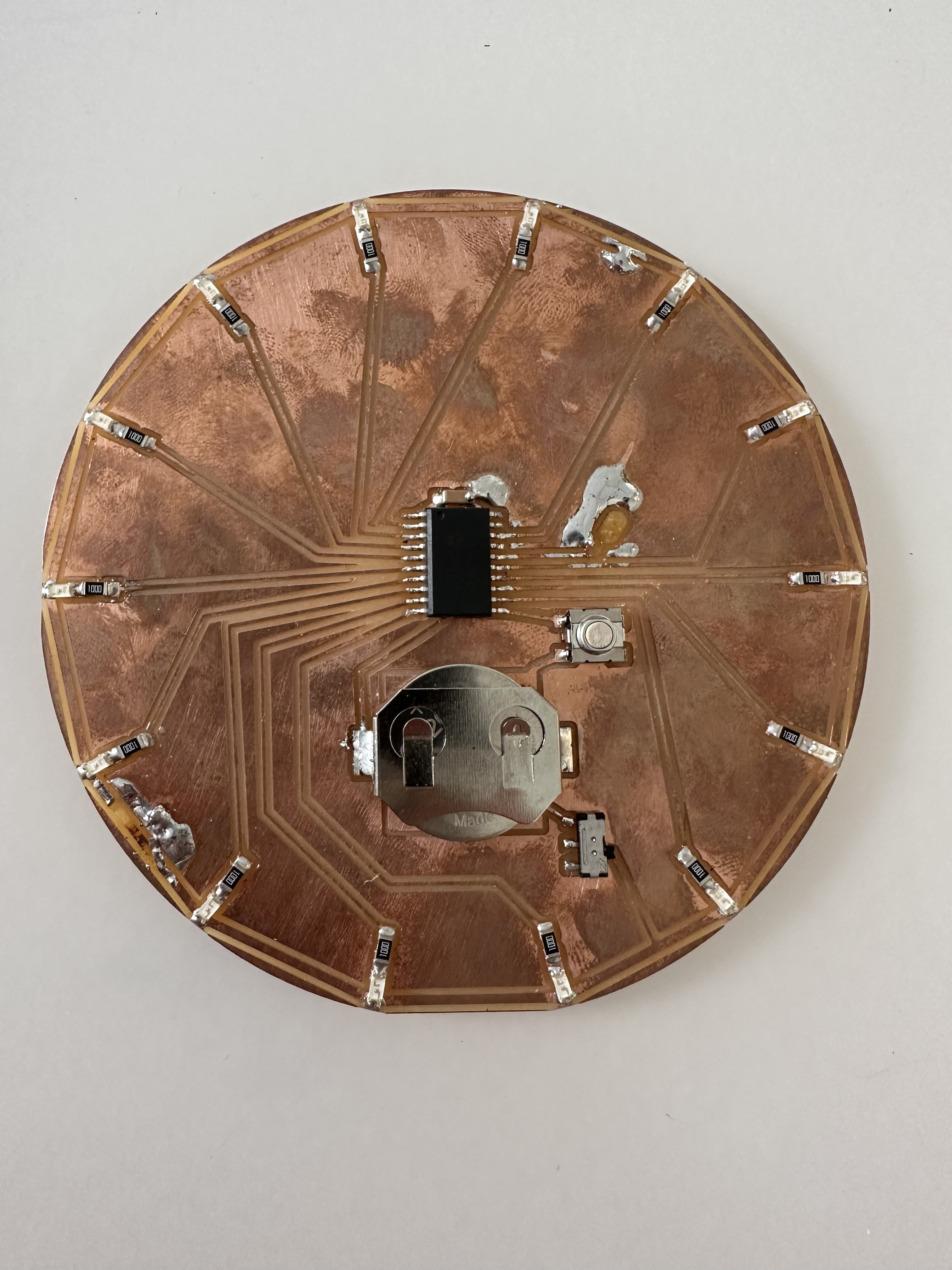
Now came the challenge of actually programming the board. I used the Arduino IDE to do this. I downloaded the megaTinyCore in my boards manager, connected an FTDI programmer to the UPDI pin of the ATtiny 3226, wrote a simple blink sketch, and pressed upload. I held my breath as it complied, but I was then hit with the error message “a programmer is needed to upload.”
I had the programmer plugged into my computer, and this sent me down a very deep rabbit hole of trying to configure the drivers on my computer and even converting an arduino uno into a jtag programmer because I thought the FTDI programmer was broken. I was about to give up when I saw the option to “Upload Using Programmer.” I clicked this and an LED started blinking on my board. This was a major facepalm moment, but at least I can now upload programs.
The sketch to make the game was not complicated. However, when I tried to run the device off the coin cell battery, the program would randomly restart. I knew that this had to be because the battery could not supply enough current. Thankfully I read in the datasheet that you could make the microcontroller consume less power if you made the internal clock slower. I had the option to reduce the clock speed all the way down to 1MHz, and after this the board worked fine off battery power.
#define LED_COUNT 14
#define BUTTON_PIN 9
#define START_DELAY 750
const byte leds[] = {
0, 1, 2, 3, 4, 5, 6, 7, 8, 10, 11, 12, 13, 15
};
unsigned long last_led_move_time = 0;
unsigned long last_button_press_time = 0;
byte stationary_led = random(0, LED_COUNT);
byte moving_led = 0;
int move_delay = START_DELAY;
bool CCW = false;
void setup() {
for(byte i = 0; i < LED_COUNT; i++) {
pinMode(leds[i], OUTPUT);
}
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
if(millis()-last_led_move_time >= move_delay) {
last_led_move_time = millis();
digitalWrite(leds[moving_led], LOW);
switch(CCW) {
case true:
moving_led = (moving_led + 1) % LED_COUNT;
break;
case false:
moving_led = (moving_led - 1 + LED_COUNT) % LED_COUNT;
break;
}
}
digitalWrite(leds[stationary_led], HIGH);
digitalWrite(leds[moving_led], HIGH);
if(digitalRead(9) == 0) {
delay(100);
if(moving_led == stationary_led) {
digitalWrite(leds[stationary_led], LOW);
stationary_led = random(0, LED_COUNT);
move_delay = 4*move_delay/5;
CCW = !CCW;
} else {
fail_animation_and_reset();
}
while(digitalRead(9) == 0) {}
}
}
void fail_animation_and_reset() {
for(byte j = 0; j < 5; j++) {
for(byte i = 0; i < LED_COUNT; i++) {
digitalWrite(leds[i], HIGH);
}
delay(300);
for(byte i = 0; i < LED_COUNT; i++) {
digitalWrite(leds[i], LOW);
}
delay(300);
}
move_delay = START_DELAY;
}
And ta-da, a working game.