Week 9 Output devices
✅ group assignment: measure the power consumption of an output device
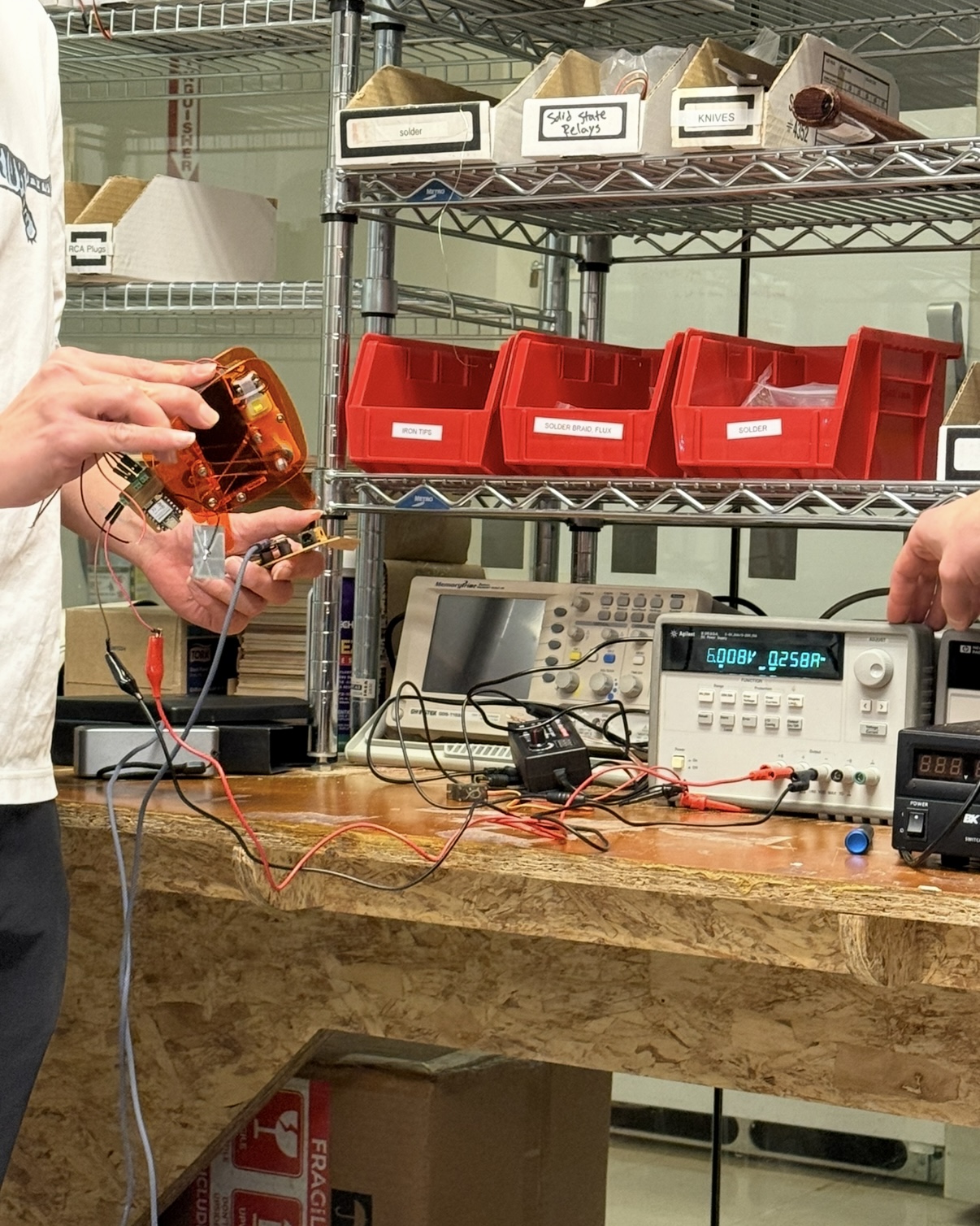
Marcello showed how to measure power consumption using the DC power supply. At 6V and 0.258A, the robot used about 1.5W when both legs moved. With one leg moving, the current was 0.15A (0.9W), and with no legs moving, it was 0.047A. This confirms that each leg requires roughly 0.1A.
✅ individual assignment: add an output device to a microcontroller board you've designed, and program it to do something
For this week I made my own LED
The Heidelberg instrument MLA maskless laser aligner
Samco Plasma Enhanced CVD System PD-220NL
Temescal FC2000 / BJD-2000 Physical Vapor Deposition System
The LED in probe station
The Lab Manual incase you want to replicate
Another Cool output device project
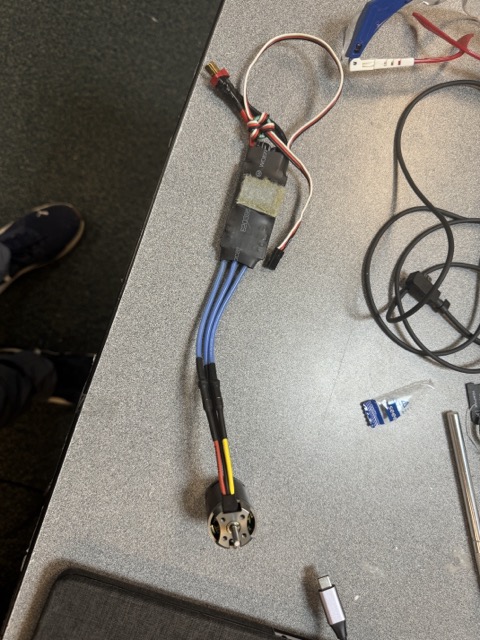
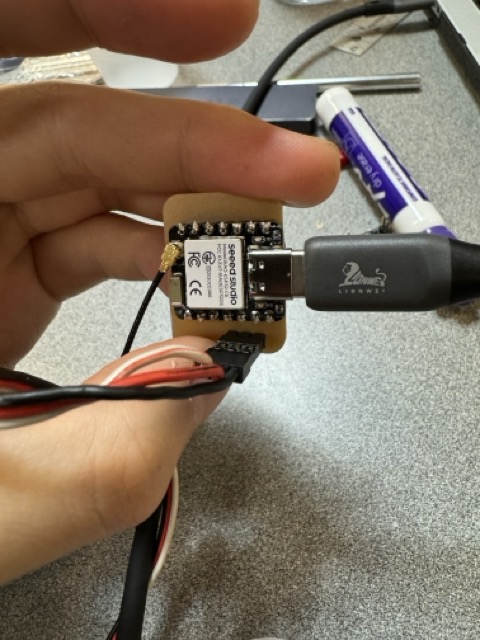
For week 9 I programed a brushless DC motor ESC, I used the Xiao rp2040 to generate a PWM signal which controls the ESC to give the motor to move, could be used for the machine linear axis in the future
// Define the pin by name
const int d1 = D1; // Using D1 as the ESC signal pin
// Timing constants
const int MIN_THROTTLE_US = 1000; // 1 ms pulse for minimum throttle
const int NEUTRAL_PULSE_US = 1500; // 1.5 ms pulse for neutral/idle
const int FULL_THROTTLE_US = 2000; // 2 ms pulse for full throttle
const int FRAME_PERIOD_US = 20000; // 20 ms frame period (typical servo signal)
void setup() {
pinMode(d1, OUTPUT);
// Arm the ESC by sending minimum throttle for a few seconds
for (int i = 0; i < 100; i++) {
sendESCPulse(MIN_THROTTLE_US);
delayMicroseconds(FRAME_PERIOD_US - MIN_THROTTLE_US);
}
// Send neutral throttle for a short time
for (int i = 0; i < 50; i++) {
sendESCPulse(NEUTRAL_PULSE_US);
delayMicroseconds(FRAME_PERIOD_US - NEUTRAL_PULSE_US);
}
}
void loop() {
// Increase throttle from neutral to full
for (int pulse = NEUTRAL_PULSE_US; pulse <= FULL_THROTTLE_US; pulse += 10) {
sendESCPulse(pulse);
delayMicroseconds(FRAME_PERIOD_US - pulse);
}
// Decrease throttle from full back down to neutral
for (int pulse = FULL_THROTTLE_US; pulse >= NEUTRAL_PULSE_US; pulse -= 10) {
sendESCPulse(pulse);
delayMicroseconds(FRAME_PERIOD_US - pulse);
}
}
void sendESCPulse(int pulseWidthUs) {
digitalWrite(d1, HIGH);
delayMicroseconds(pulseWidthUs);
digitalWrite(d1, LOW);
}