Week 11
Interface and Application Programming
This week was fun! I built a new pcb to get more prepared for my final project which involves controlling 4 vibro-motors. Based on previous experiences I made this board more successful than the other ones I believe! Here is my KiCAD schematic. The idea was to have 4 vibro-motors and two photoresistors to control the motors with light, but actually I feel like, I would use proximity sensor kind of thing for final.

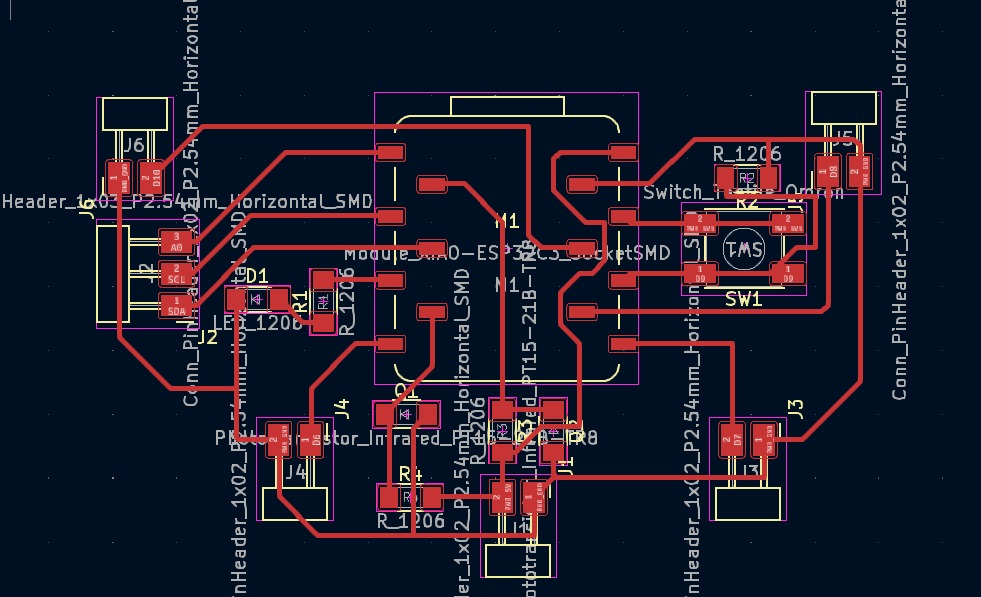
After miling and soldering my pcb looked like this:
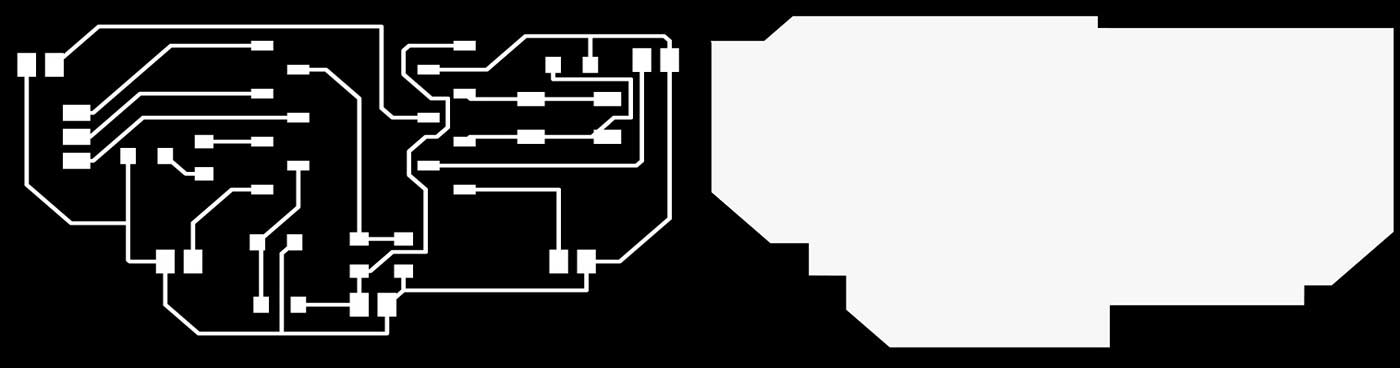
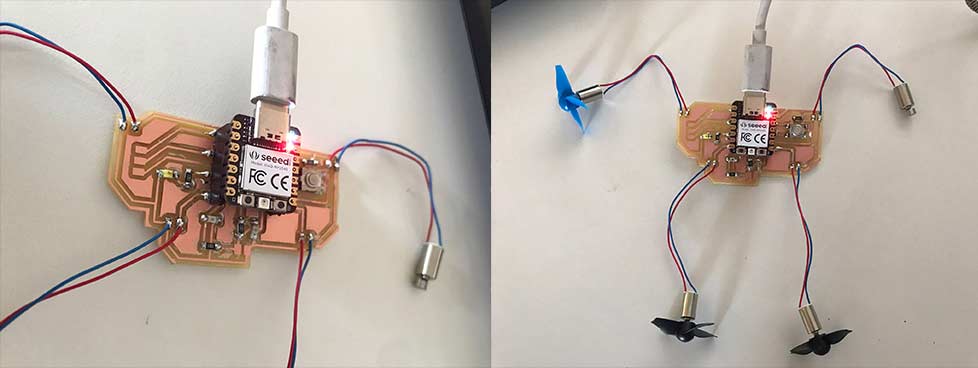
After finishing the PCB I tested it and yay!! It was working.
Now, it was time to implement an interface for my pcb, it was easier than I thought. My initial idea was to have 4 buttons on screen and one by one controlling motors individually. I will try to expand this idea and I hope to control my device remotely from computer for the final project. For now here is the demonstration:
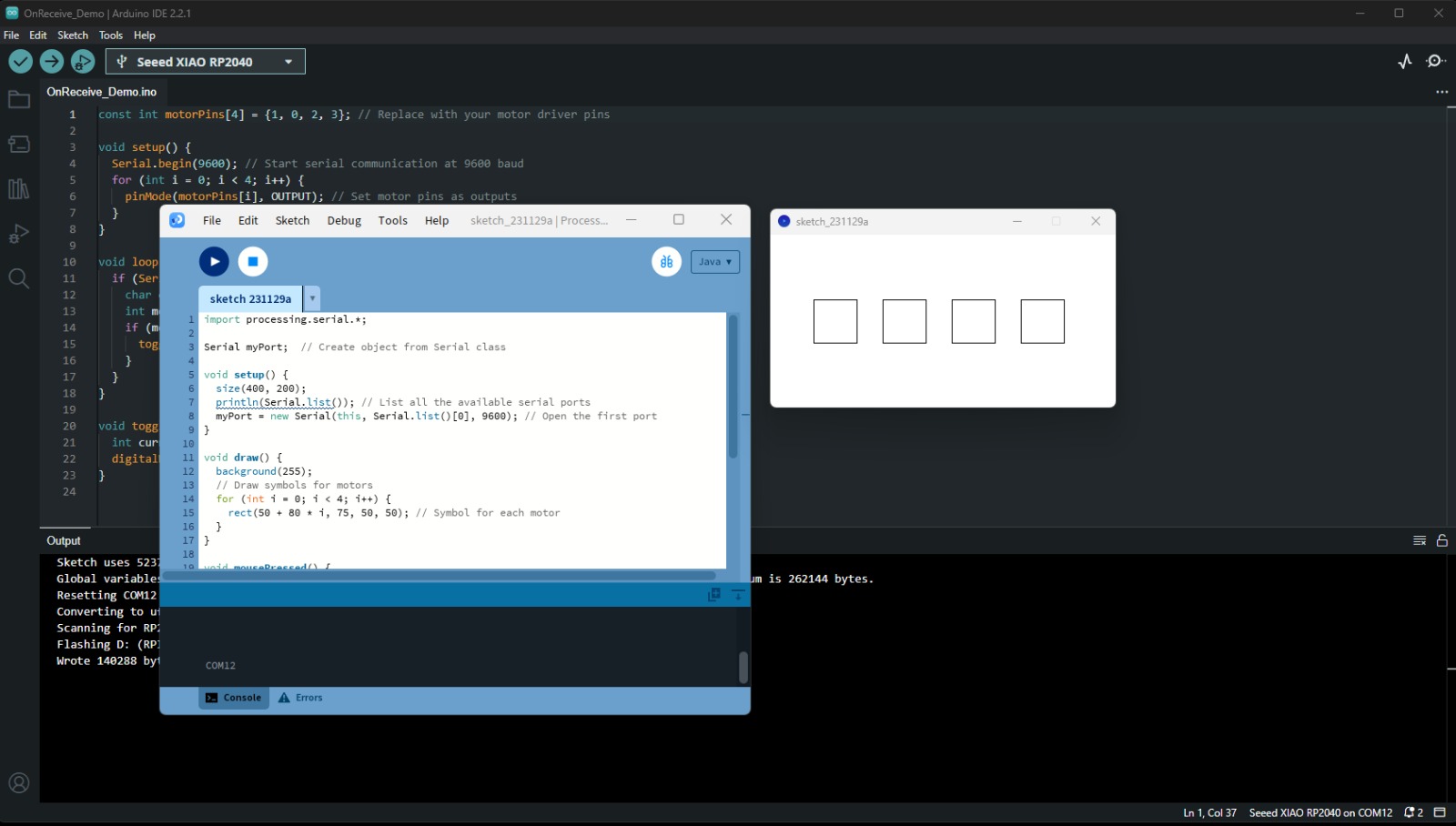
Here is the code I used for the interface:
This is arduino code:
const int motorPins[4] = {1, 0, 2, 3}; // Replace with your motor driver pins
void setup() {
Serial.begin(9600); // Start serial communication at 9600 baud
for (int i = 0; i < 4; i++) {
pinMode(motorPins[i], OUTPUT); // Set motor pins as outputs
}
}
void loop() {
if (Serial.available()) { // Check if data is available to read
char command = Serial.read(); // Read the incoming byte
int motorIndex = command - '0'; // Convert char to int (e.g., '1' becomes 1)
if (motorIndex >= 1 && motorIndex <= 4) {
toggleMotor(motorIndex - 1); // Adjust index for zero-based array
}
}
}
void toggleMotor(int index) {
int currentState = digitalRead(motorPins[index]);
digitalWrite(motorPins[index], !currentState); // Toggle the motor state
}
And this is the processing code:
import processing.serial.*;
Serial myPort; // Create object from Serial class
void setup() {
size(400, 200);
println(Serial.list()); // List all the available serial ports
myPort = new Serial(this, Serial.list()[0], 9600); // Open the first port
}
void draw() {
background(255);
// Draw symbols for motors
for (int i = 0; i < 4; i++) {
rect(50 + 80 * i, 75, 50, 50); // Symbol for each motor
}
}
void mousePressed() {
for (int i = 0; i < 4; i++) {
if (overRect(50 + 80 * i, 75, 50, 50)) {
myPort.write(str(i + 1).charAt(0)); // Send command to toggle corresponding motor
}
}
}
boolean overRect(int x, int y, int width, int height) {
return mouseX >= x && mouseX <= x+width &&
mouseY >= y && mouseY <= y+height;
}