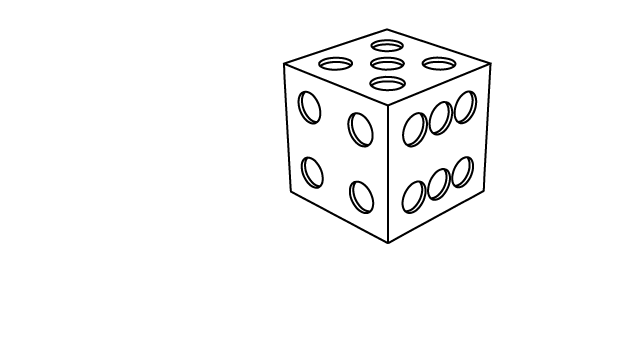
Project 12
Graphical User Interfaces (GUIs)
Embedded programming
This week I used the RFduino, to run my embedded program. The program is quite straightforward and relies on two major libraries. 1) the Arduino library and 2) the RFduino library. With these two libraries, the code necessary to read in values and publish useful information over BLE is very simple. For my first application, the embedded program simply publishes roll, pitch, an yaw (or rotation around x, y, and z) as values that are incrementing. This allows me to see values published, even though they are not based on the actual accelerometer.
Note: To upload RFduino code, the RFduino board needs to be specified, as the processor is an ARM Cortex M0, which is a different beast than the AVR chips we have been using.
Here is my RFduino Code as written in the Arduino IDE
/*
This RFduino sketch demonstrates a Bluetooth Low Energy 4 connection
between an iPhone application and an RFduino.
This sketch works with the the Dice++ iPhone application.
This sketch demonstrates publishing x, y, and z rotational values for
the dice, and the iPhone app will receive this information formatted in
a friendly constant length string to be parsed and inform the visualization.
*/
#include
int x, y, z;
String data;
bool bRotateX = false;
bool bRotateY = false;
bool bRotateZ = false;
void setup() {
// this is the data we want to appear in the advertisement
// (if the deviceName and advertisementData are too long to fix into the 31 byte
// ble advertisement packet, then the advertisementData is truncated first down to
// a single byte, then it will truncate the deviceName)
RFduinoBLE.advertisementData = "dice++";
// start the BLE stack
RFduinoBLE.begin();
Serial.begin(9600);
}
void loop() {
if(Serial.available()){
char val = Serial.read();
Serial.println(val);
if(val == 'x'){
bRotateX = !bRotateX;
}
if(val == 'y'){
bRotateY = !bRotateY;
}
if(val == 'z'){
bRotateZ = !bRotateZ;
}
}
// send some fake data, increment the
if(bRotateX)
x++;
if(bRotateY)
y++;
if(bRotateZ)
z++;
if(x > 360)
x = 0;
if(y > 360)
y = 0;
if(z > 360)
z = 0;
data = getStringWithXYZ(x,y,z);
char charBuf[data.length()];
data.toCharArray(charBuf, data.length());
int length = strlen(charBuf);
if( length <= 20){
RFduinoBLE.send(charBuf,length);
}else{
RFduinoBLE.send(charBuf,20); // BLE packet is 20 bytes max.
}
//wait .01 seconds (publishing ~100 messages per second!!)
delay(10);
}
// convert a radian into an integer degree value
int getIntDegreeValueForRadian(float val) {
return (int)(val * 180 / PI);
}
// create a string from int values for rotation
String getStringWithXYZ(int x, int y, int z)
{
String str;
str = "x";
str+=getThreeDigitString(x);
str+="y";
str+=getThreeDigitString(y);
str+="z";
str+= getThreeDigitString(z);
str+="/"; // this last character gets lost in transmission
return str;
}
// create a string from an integer to take up 3 characters of space
// this is inefficient, but keeps formatting nice
String getThreeDigitString(int val) {
String str;
if(val < 10) {
str = "00";
str += val;
}
else if(val < 100) {
str = "0";
str += val;
}
else {
str = ""; // needs to add the integer like so, otherwise it complains about the type
str += val;
}
return str;
}
void RFduinoBLE_onDisconnect()
{
}
3D Model
Nothing new here, just wanted to center my model around the origin to make it a nice model for the iPhone app to play with.
iOS Programming
Clearly this post is not going to teach all of the steps necessary to make an iOS application, but attached will be some useful tutorials, some tips and tricks, and what I used to quickly make an app that does what I want. One of my favorite sources for iOS tutorials is http://www.raywenderlich.com/ which continues to be updated with the latest, including the move to Swift programming.
Creating an App
Seeing the App on the iPhone
To load an app onto your iPhone, you do need to have an iOS developer account. Here is a relatively new and great resource directly from Apple on the starting process: https://developer.apple.com/library/ios/referencelibrary/GettingStarted/RoadMapiOS/.
Adding Libraries
There are two libraries in particular that I was dependent on this week. 1) RFduino's iOS SDK and 2) A nice OpenGLES2 library for rendering .obj models simply. With Apple's latest release of iOS 8, I could have also used SpriteKit to load in collada (.dae) files, but I noticed this too late. Back in the day, Apple actually required that the 3D files be specified in header files for OpenGL, but this library converts the coordinate so I don't need to do any pre-processing of the 3D file.
https://github.com/nicklockwood/GLView
https://github.com/RFduino/RFduino or http://www.rfdigital.com/download-rfduino-library/
Xcode
While Xcode is an amazing IDE (programming environment), there are so many features that are simply hidden to the beginner, and are not congruent with most peoples ideas of how programming works. For example, Interface Builder is a feature of Xcode that allows people to easily layout interfaces visually, a sort of WYSIWYG, but requires linking back to the code that will control and update it. To link an element that is dragged into the Interface Builder, you need to hold 'control' and drag from the component into the text file which is your code. This feels odd at first... actually it still feels odd, but it is quite powerful and most iOS apps are built this way.
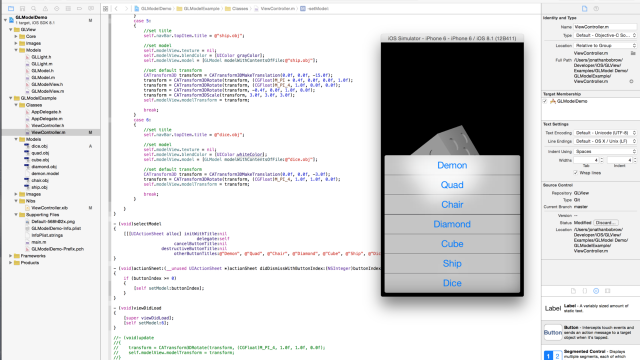
Here I am simply loading my .obj file into a demo sketch for the GLView library. After successfully seeing my model, it was time to create a new app and add in the animations that I want to display.
DicePlusPlus on GitHub
All of the iOS code is now hosted on GitHub, and will continue to be updated here: https://github.com/jbobrow/DicePlusPlus
The App + RFduino in action
Here is a lil video demo of me plugging the RFduino into the computer with USB for power as well as Serial communication. Hitting an 'x' on the keyboard starts/stops the die rotating on its x axis and the same goes for y and z. Please excuse the background noise, there just so happened to be a baby as well as a jazz pianist hanging in the space :)
Making a nice App icon
If you are gonna have an app, you better have a great App icon, and here is the quickest way to fit the Apple standard guidelines and make it quickly in all of the correct resolutions. http://appicontemplate.com/