Input Devices
For this week, I decided to modify my Hatsune Miku Board from week 6 by adding a capacitance sensor. I had an unused pin, so I attached the sensor to that one.
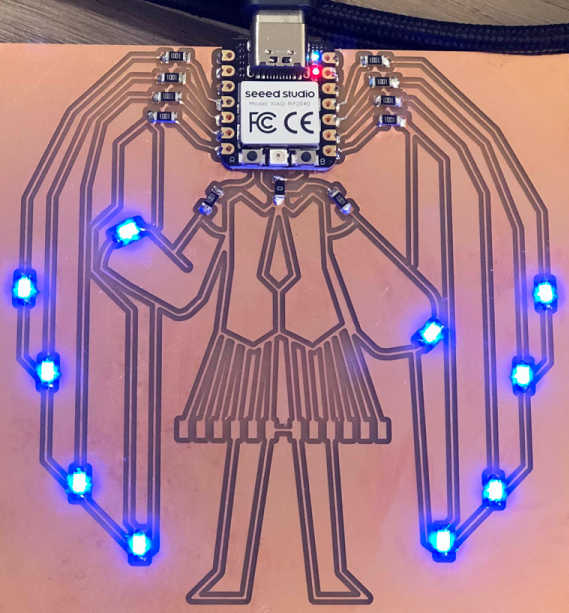
To test out the board, I wrote a program that would light up if my hand was near the copper.
I had originally wanted the board to change the brightness of the LEDs based on how close my hand was, but I found it to be more finicky and prone to fluctuations to accomplish that. Part of it could be my setup also might not have been the best, but I did observe that both the wire and the board weren't sensitive to the touch.
On my final project board, I decided to try it again. This time, I included a ground plane, as well as a 1M ohm resistor to ground. This value was then fed into an LED to change the brightness. This time, the capacitance sensor worked better than my last test, but one still needed to place their hand on it in order for it to work as expected.
I first printed out the values of the capacitance sensor to see how much it was reading

Then, I scaled down the range and capped it at 255 to write to a Neopixel.
Resources
Code
#
# hello.steptime1.RP2040.py
#
# 1 pin step-response loading measurement with PIO
#
# Neil Gershenfeld 7/24/24
#
# This work may be reproduced, modified, distributed,
# performed, and displayed for any purpose, but must
# acknowledge this project. Copyright is retained and
# must be preserved. The work is provided as is; no
# warranty is provided, and users accept all liability.
#
# install MicroPython
# https://micropython.org/download/RPI_PICO/
#
from machine import Pin,freq
import rp2
import neopixel
@rp2.asm_pio(set_init=rp2.PIO.OUT_HIGH)
def steptimer():
#
# initialize
#
pull()
mov(y,osr) # move loop to y
pull() # move settle to osr
mov(x,null) # move count to x
set(pins,1) # turn on pin
#
# main loop
#
label('main loop')
#
# charge up
#
set(pindirs,1) # set pin to output
#
# settle up
#
label('up settle')
mov(isr,x)
mov(x,osr)
label('up settle loop')
jmp(x_dec,'up settle loop')
mov(x,isr)
#
# discharge
#
set(pindirs,0) # set pin to input
#
# time down
#
label('down loop')
jmp(pin,'down jump')
jmp('down continue')
label('down jump')
jmp(x_dec,'down loop')
#
# loop
#
label('down continue')
jmp(y_dec,'main loop')
#
# push count
#
mov(isr,x)
push()
class steptime:
def __init__(self,sm_id,pin):
self._sm = rp2.StateMachine(sm_id,steptimer,
jmp_pin=pin,set_base=pin)
self._sm.active(True)
self.get = self._sm.get
self.put = self._sm.put
freq(250000000)
step0 = steptime(0,Pin(19))
#step1 = steptime(1,1)
#step2 = steptime(2,2)
#step3 = steptime(3,4) # these are flipped in pinout
#step4 = steptime(4,3) # "
loop = 200
settle = 20000
min0 = min1 = min2 = min3 = min4 = 1e6
pix = Pin(21, Pin.OUT)
sun = neopixel.NeoPixel(pix, 1)
def get_reading():
min0 = 1e6
step0.put(loop)
step0.put(settle)
result0 = 4294967296-step0.get() # +1 for init 0
if (result0 < min0): min0 = result0
val = min(result0//10000, 255)
sun[0] = (val, val, 0)
sun.write()
while True:
print('loop started')
step0.put(loop)
step0.put(settle)
result0 = 4294967296-step0.get() # +1 for init 0
if (result0 < min0): min0 = result0
val = min(result0//10000, 255)
print(val)
sun[0] = (val, val, 0)
sun.write()
Files can be found in final project