Week 8
Input Devices
Part 1: Designing the PCB
Weekly assignment - measure something: add a sensor to a microcontroller board that you have designed and read it. For this week, I planned to design a board with a light sensor, then program it to measure the light intensity.
I used KiCad to design my PCB, adding a couple light sensors so that I could cover some of them and measure different light intensities as I covered/uncovered them. That left me with this schematic:
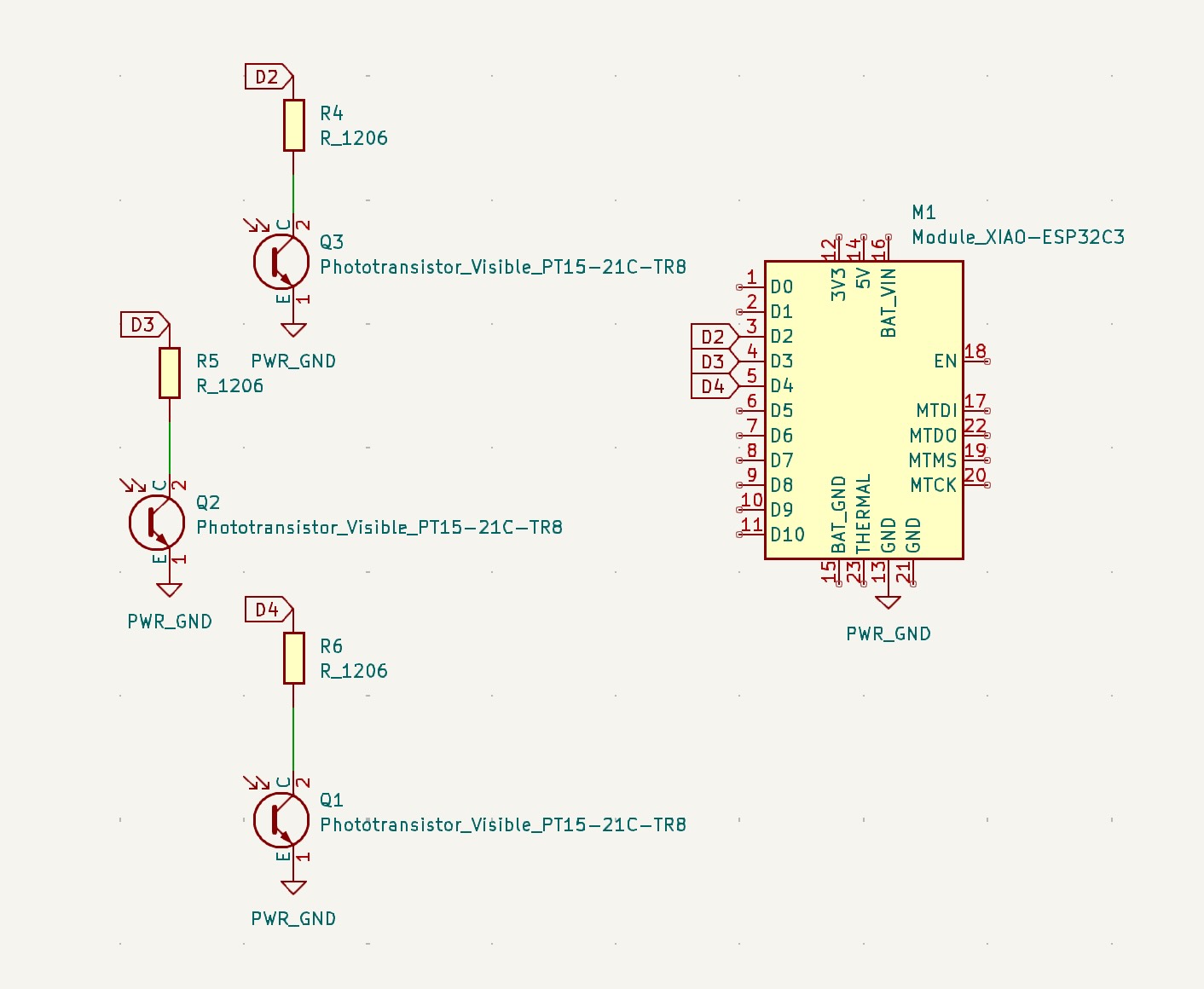
In the PCB editor, I added the traces (and a little light bulb shape for decoration):
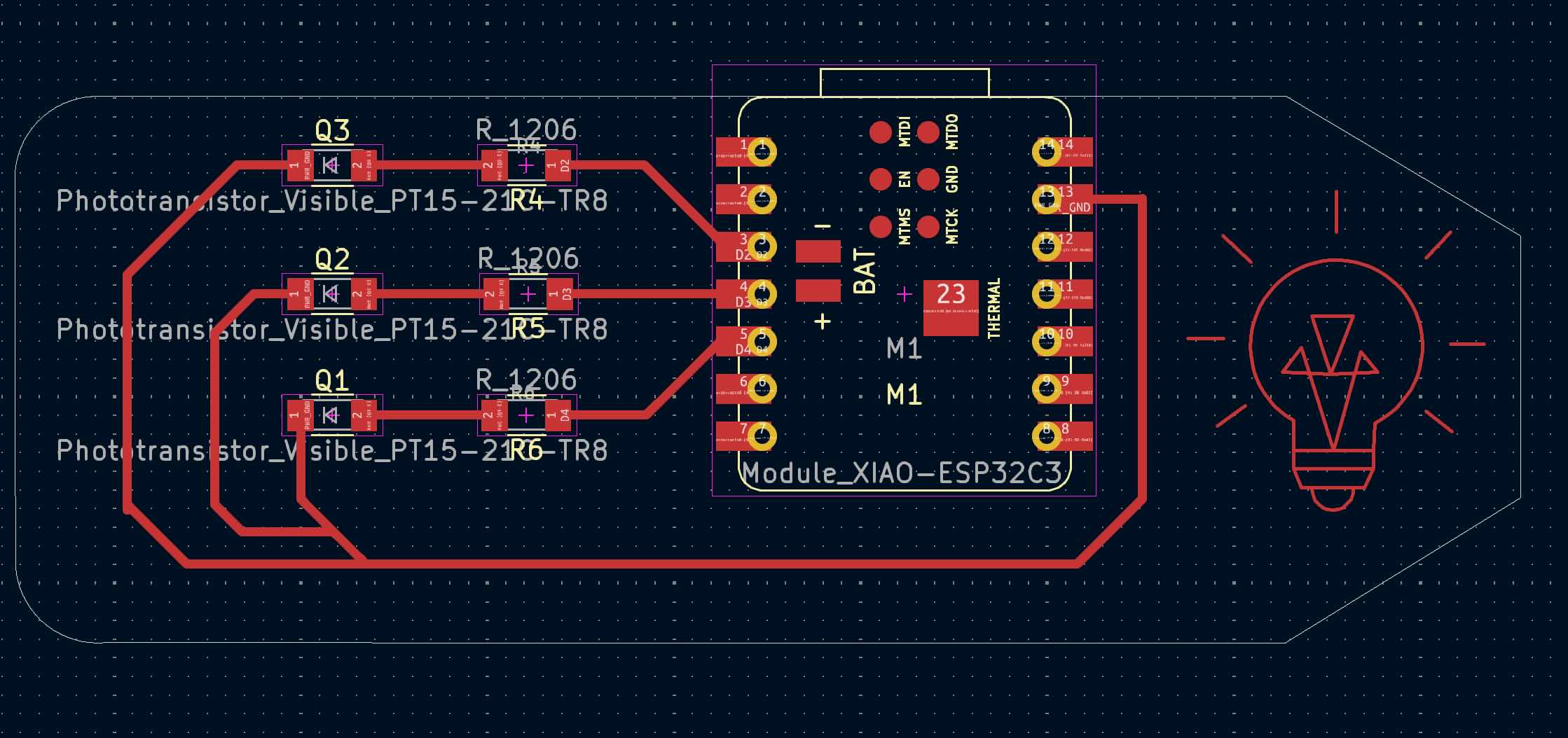
Part 2: Milling
After exporting to an SVG and inverting the colors, I have the following edge and trace PNG files:
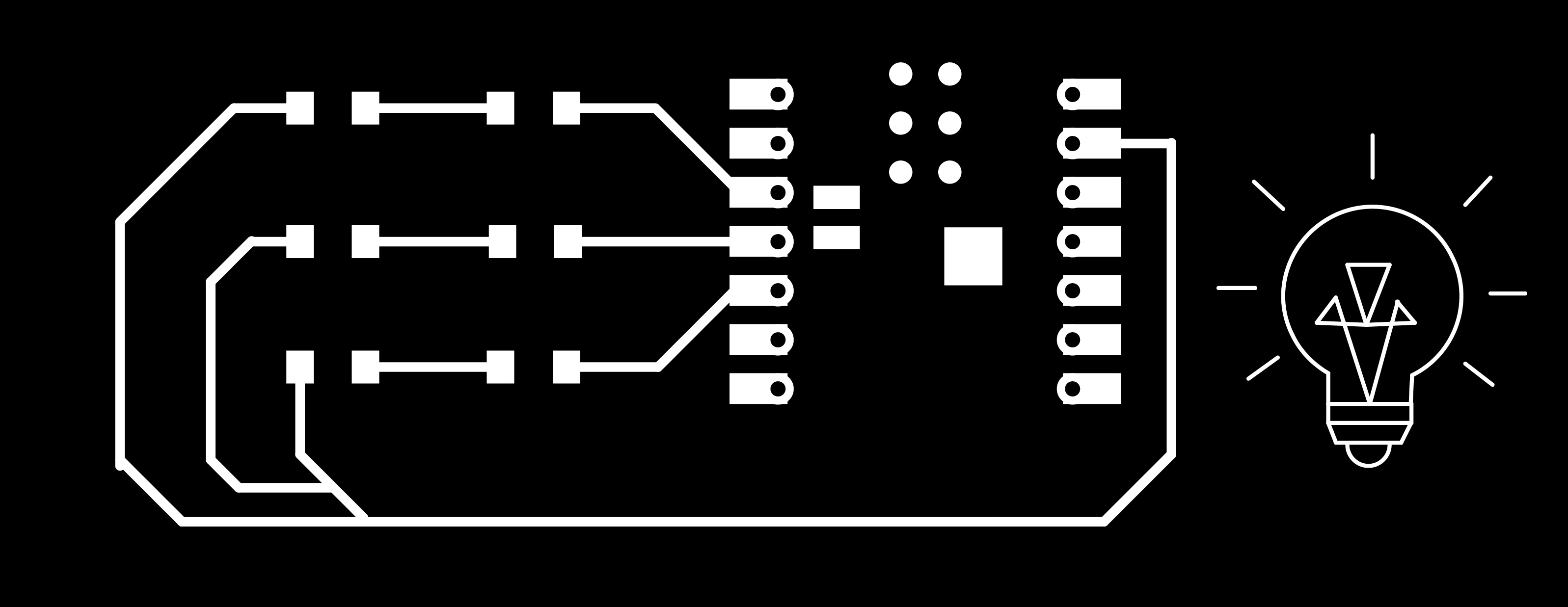
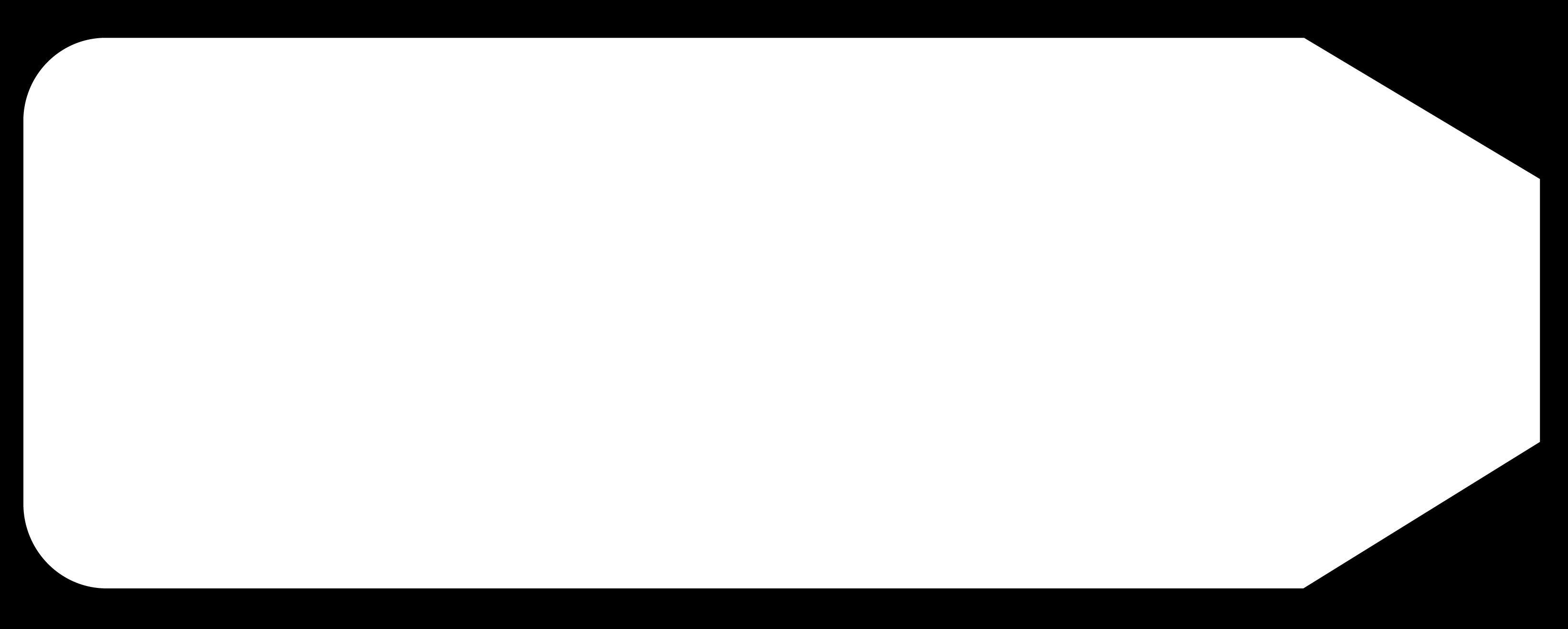
I used (this) software to mill.
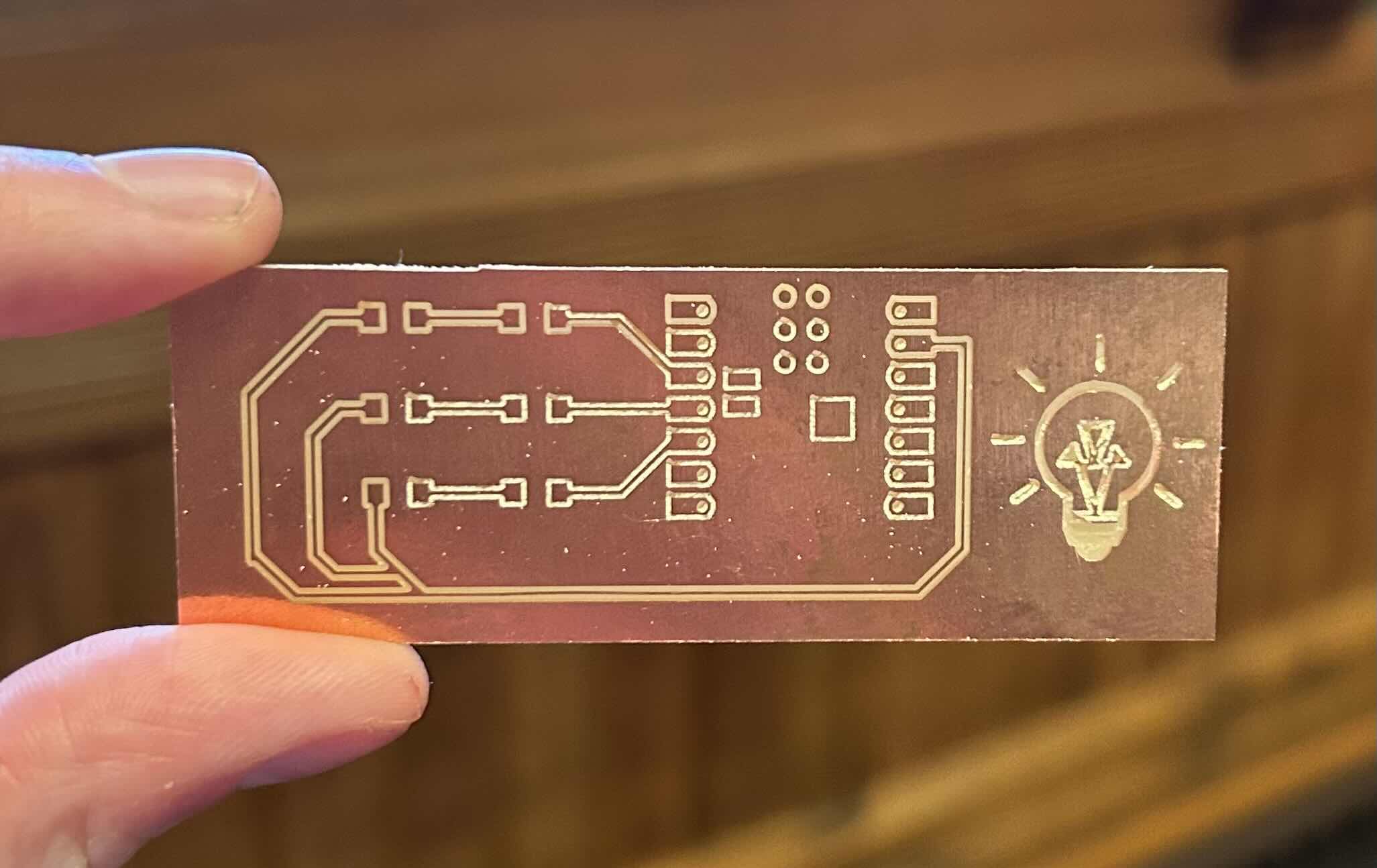
Part 3: Pivot
Unfortunately, when I went back to the shop to solder, I realized that in the time since I'd designed the board, we had run out of all inventory in the light sensors I needed :'( So while I wasn't able to finish working with this input device, below is documentation for an input device I ultimately integrated into my final project.
You can find the expanded documentation for the PCB design in the final project section of my site (here).
For this input device, I read measurements from a Force-Sensitive Resistor, to detect the live force exerted on the sensor. I designed and milled the following PCB (which also includes other components-- the pins going to the FSR lead to the header sticking out horitzonally on the left in the images below):
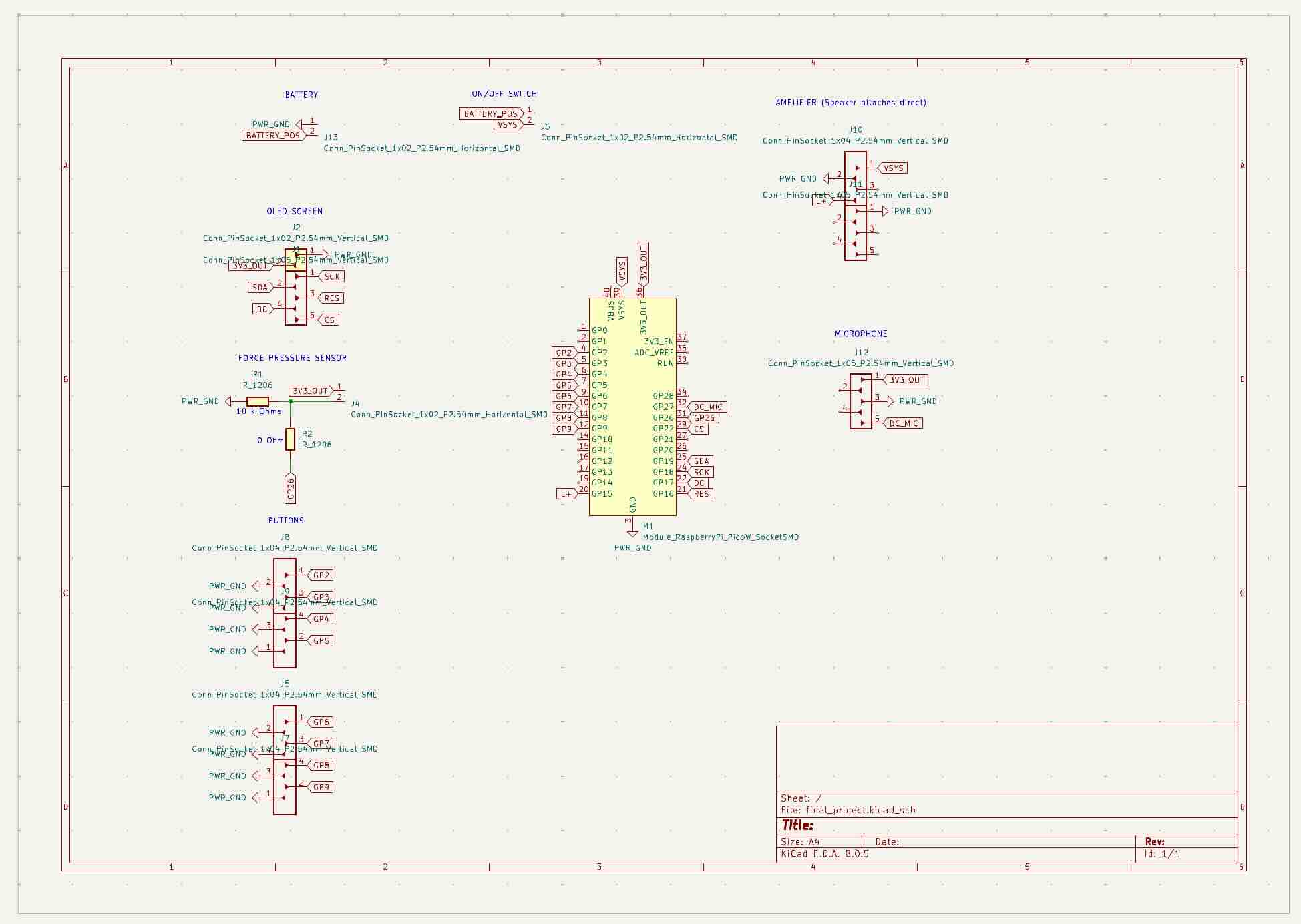
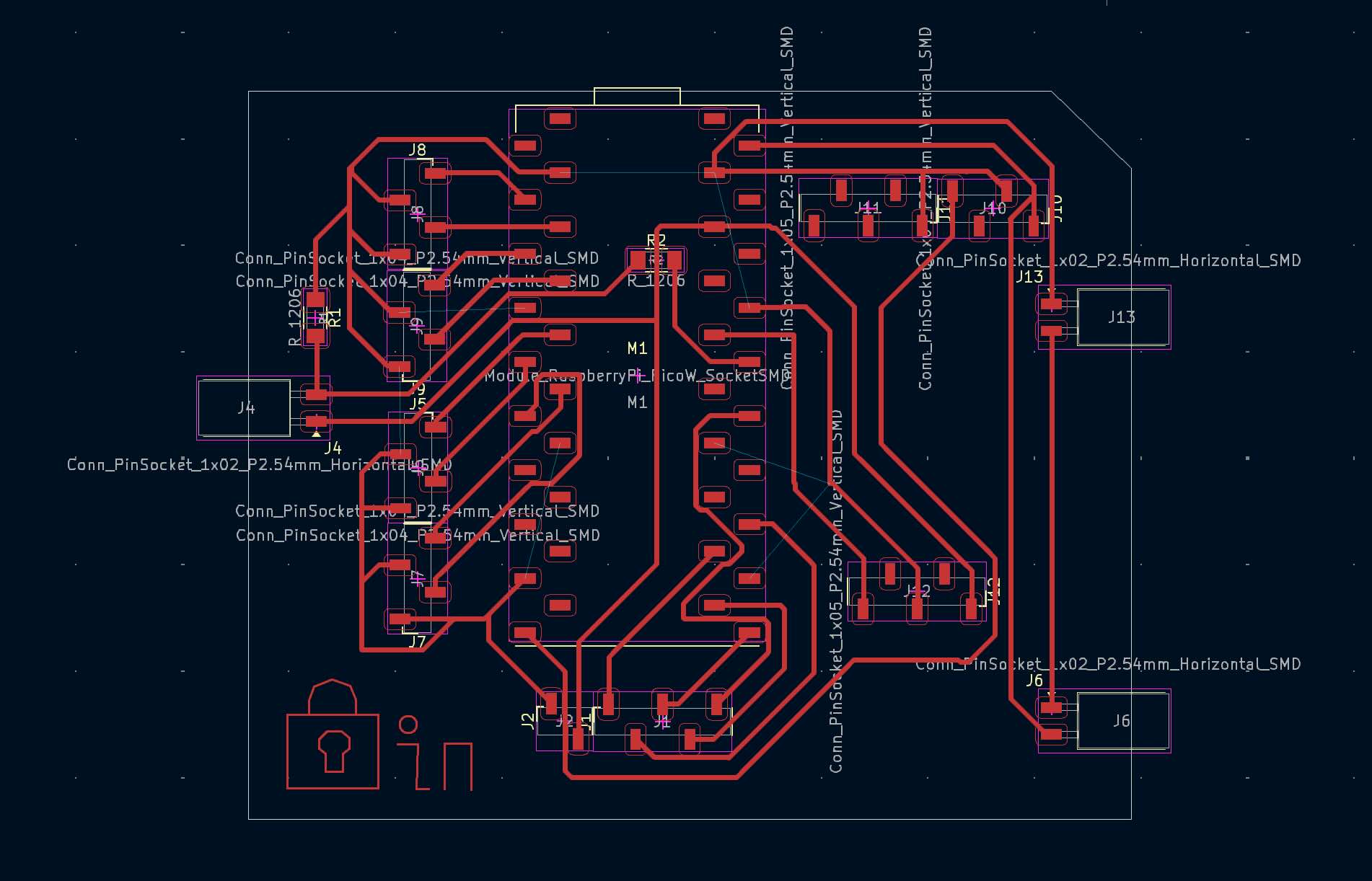
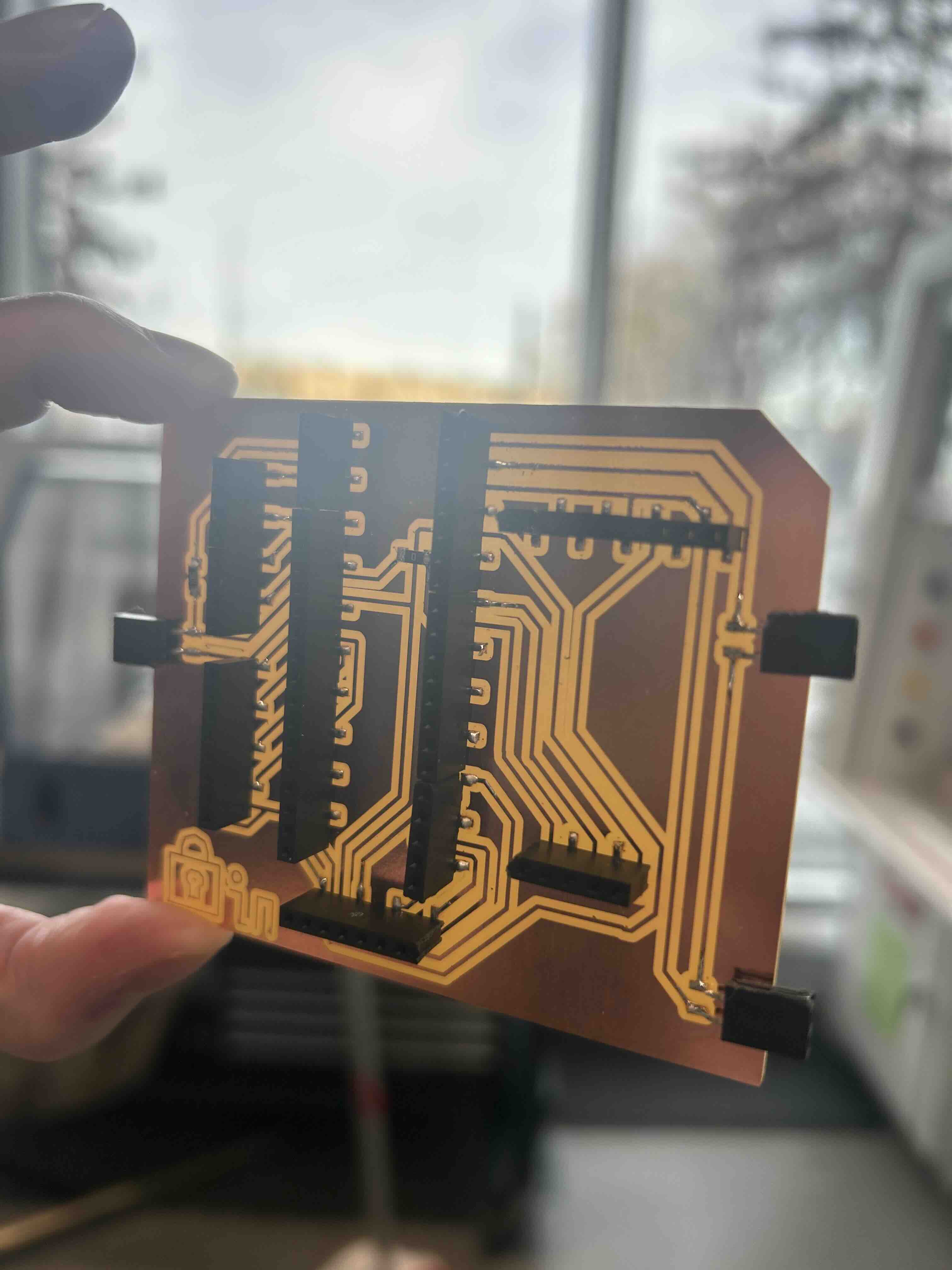
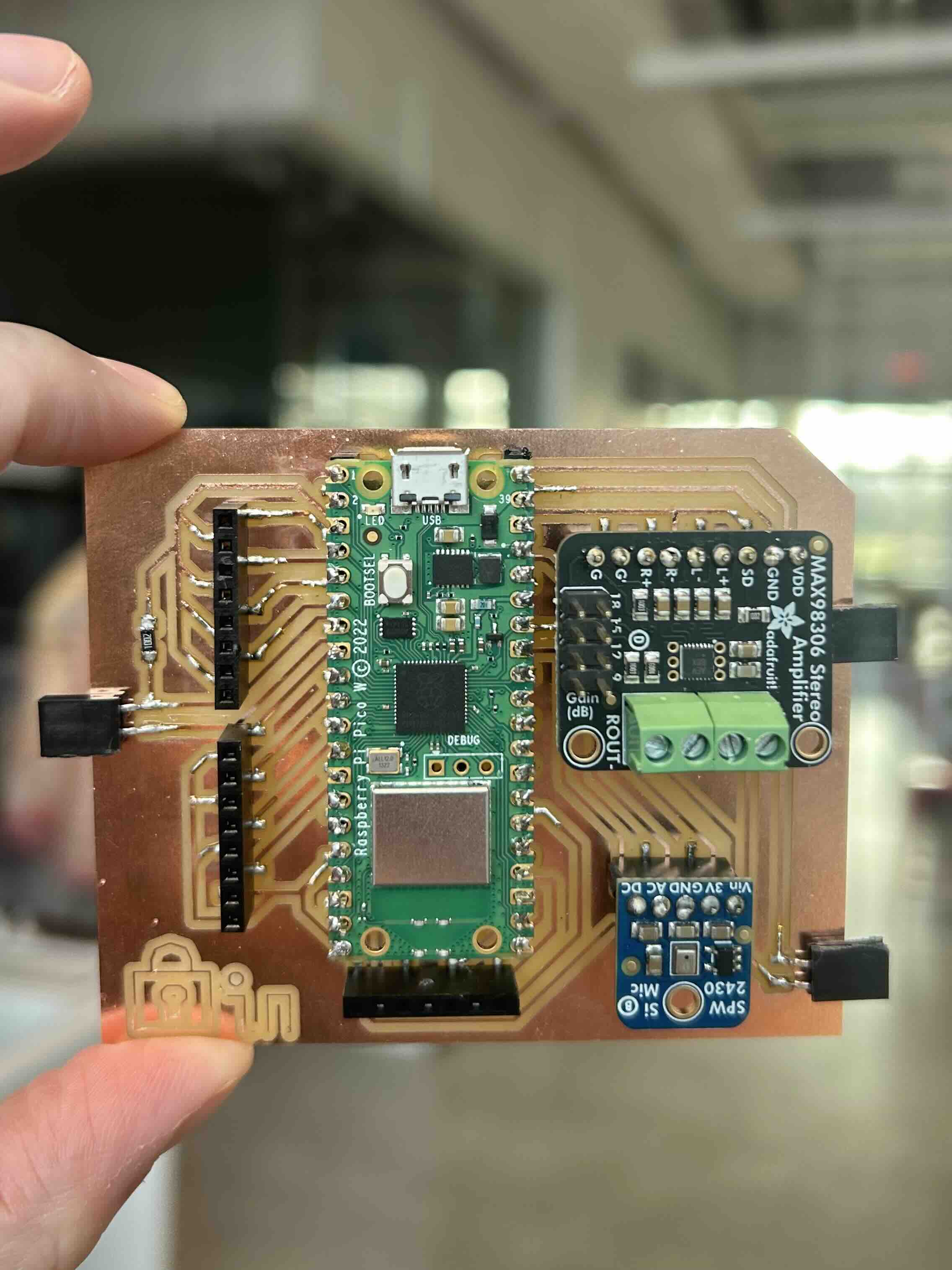
I programmed this to read the force exerted on the sensor and then display the output on the OLED:
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
#define OLED_MOSI 19 // SPI Data (SDA)
#define OLED_CLK 18 // SPI Clock (SCK)
#define OLED_DC 17 // Data/Command
#define OLED_CS 22 // Chip Select
#define OLED_RESET 16 // Reset
// FSR pin definition
#define FSR_PIN A0
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &SPI, OLED_DC, OLED_RESET, OLED_CS);
void setup() {
Serial.begin(115200);
Serial.println("Starting OLED and FSR test...");
// Initialize the OLED display
if (!display.begin(SSD1306_SWITCHCAPVCC)) {
Serial.println("SSD1306 allocation failed");
while (true);
}
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(SSD1306_WHITE);
display.setCursor(0, 0);
display.println("FSR Test Starting...");
display.display();
delay(2000);
}
void loop() {
// Read the FSR value
int fsrValue = analogRead(FSR_PIN);
// Print the FSR value to the Serial Monitor
Serial.print("FSR Value: ");
Serial.println(fsrValue);
// Display FSR value on the OLED
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(SSD1306_WHITE);
display.setCursor(0, 0);
display.print("FSR: ");
display.println(fsrValue);
display.display();
delay(1000); // Update every second
}
This allowed me to successfully read the data from this sensor: