This week I wanted to add a temperature sensor to my mouse behavior rig. I expected that we would have a traditional thermistor in lab, but instead we just have a one-wire thermistor thing (DS18B20 TO-92). Datasheet here. The component seems to have been designed in Dallas (by dallas semiconductor) which is where I am from!
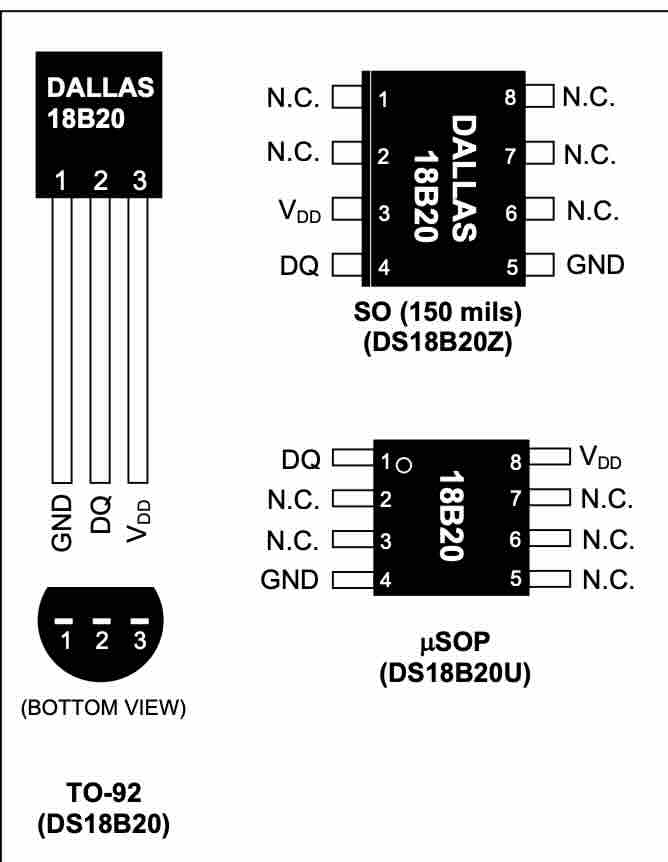
I connected the correct pins to ground, power and digital for data. I added a 4.7kOhm resistor between data and power pins. I uploaded this code to my arduino:
arduino script: thermometer example
//sketch to use temp sensor
#include <OneWire.h>
#include <DallasTemperature.h>
float temp = 0.0;
int oneWireBus = 2; //one wire temp pin
OneWire oneWire(oneWireBus);
DallasTemperature sensors(&oneWire);
void setup() {
pinMode(LED_BUILTIN, OUTPUT);
digitalWrite(LED_BUILTIN, LOW);
Serial.begin(9600);
Serial.println("One wire temp sensor");
sensors.begin();
}
void loop() {
sensors.requestTemperatures();
temp = sensors.getTempFByIndex(0);
Serial.print("Temperature: ");
Serial.println(temp);
delay(500);
}
In testing it, I found that this thermistor senses environment temperature (stably reads ~75F, room temp) but does not seem to have a way to measure the temperature of a specific wire/location. The temperature sensor is housed inside plastic. I think that thermal conductivity of the casing is the limiting factor. I was dissapointed by this, so I chipped off some of the plastic to get potentially quicker, more localized readings.
It does not measure body temperature well. I held the component body in my hand and it continued to read 75 F. So unless im severely hypothermic, it is not adjusting quickly enough or lacks sensitivity. I then held the tip of a 400C soldering iron near the component for one minute, and eventually got a reading up to 100 C with the intact component and 225 F with the chipped plastic casing. This is still way below the expected temperature but the component is not rated for sensitivty beyond 150C. The lethargy in which the temperature reading increased indicates to be that this chip is designed for measuring stable environmental temperatures, but not necessaryily localized (body) temperature or rapidly changing temperatures.
####Debug: What resolution am I measuring at? The device enables 9-10-11-12 bit resolution. The temperature conversion time depends on the bit resolution. Maybe this can partially explain the problem I describe aboce. Nevermind, 750 ms is still less than 1 sec.

I’m instead going to look into DallasTemperature library to check the calculations. Navigate to DallasTemperature directory. open .h file which defines functions + variables in C.