Component: Syringe Pump
The purpose of this component is to provide precise ~1µL metering of a liquid using a standard luer
lock 5mL syringe.
The syringe pump includes a stepper motor and a limit switch to home the device between uses. The
intention is that the user can include a syringe pump in a larger system and use it to dispense
liquid in a precise manner.
Ideally this tool is used with a fluid source, and a one way valve. On retraction [positive values]
in the source code, the syringe aspirates fluid from the source via the one way valve, and on
dispense [negative values] the syringe pumps fluid into your target system.
The pump can easily be integrated with 3D printers or other liquid handling systems.
File available at OnShape Link
Source Code
// warning: without a powered usb-hub, currentScale > 0.5 are likely to fail
await syringeStepper.setCurrent(0.8);
await syringeStepper.setStepsPerUnit(200);
// function that defines a homing command using homing workflow
async function home(vel){
// retract slide to limit switch
while (await syringeStepper.getLimitState()) {
await syringeStepper.velocity(vel);
}
// at limit switch, stop moving and set home position to 0
await syringeStepper.velocity(0);
await syringeStepper.setPosition(0);
// pause and move off the limit switch
await delay(500);
await syringeStepper.relative(2,5);
// pause and set home position
await delay(500);
await syringeStepper.setPosition(0);
}
// function that defines the homing workflow
async function homeSeq(){
// fast home to limit switch
await home(-10);
// slow home to limit switch for increased precision
await home(-1);
// dispense to prime
await syringeStepper.relative(2,5);
}
// run the homing sequence
await homeSeq();
// example code: aspirate 45mm, pause, and dispense all 45mm
await syringeStepper.absolute(45,5);
await delay(1000);
await syringeStepper.absolute(0,5);
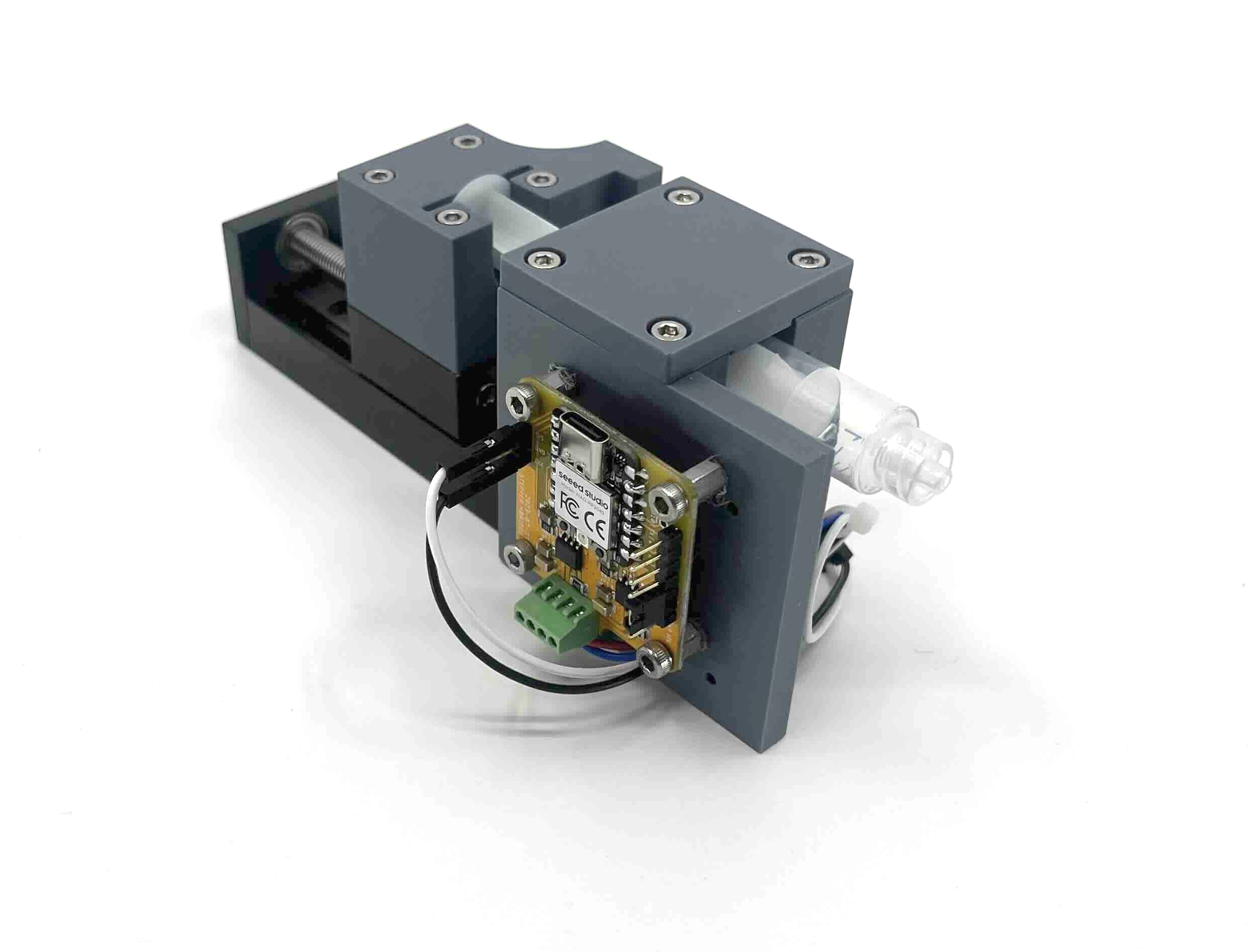
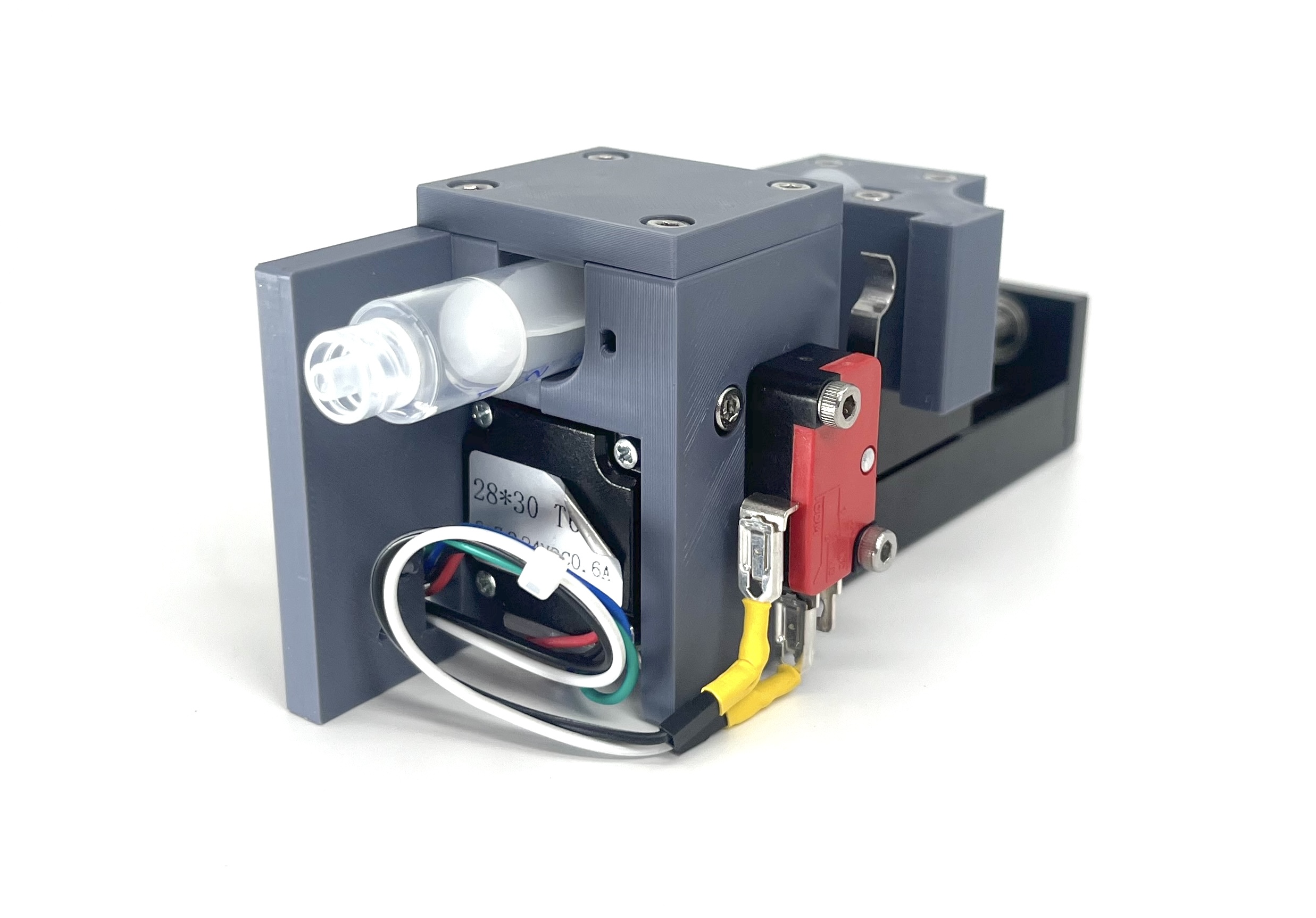
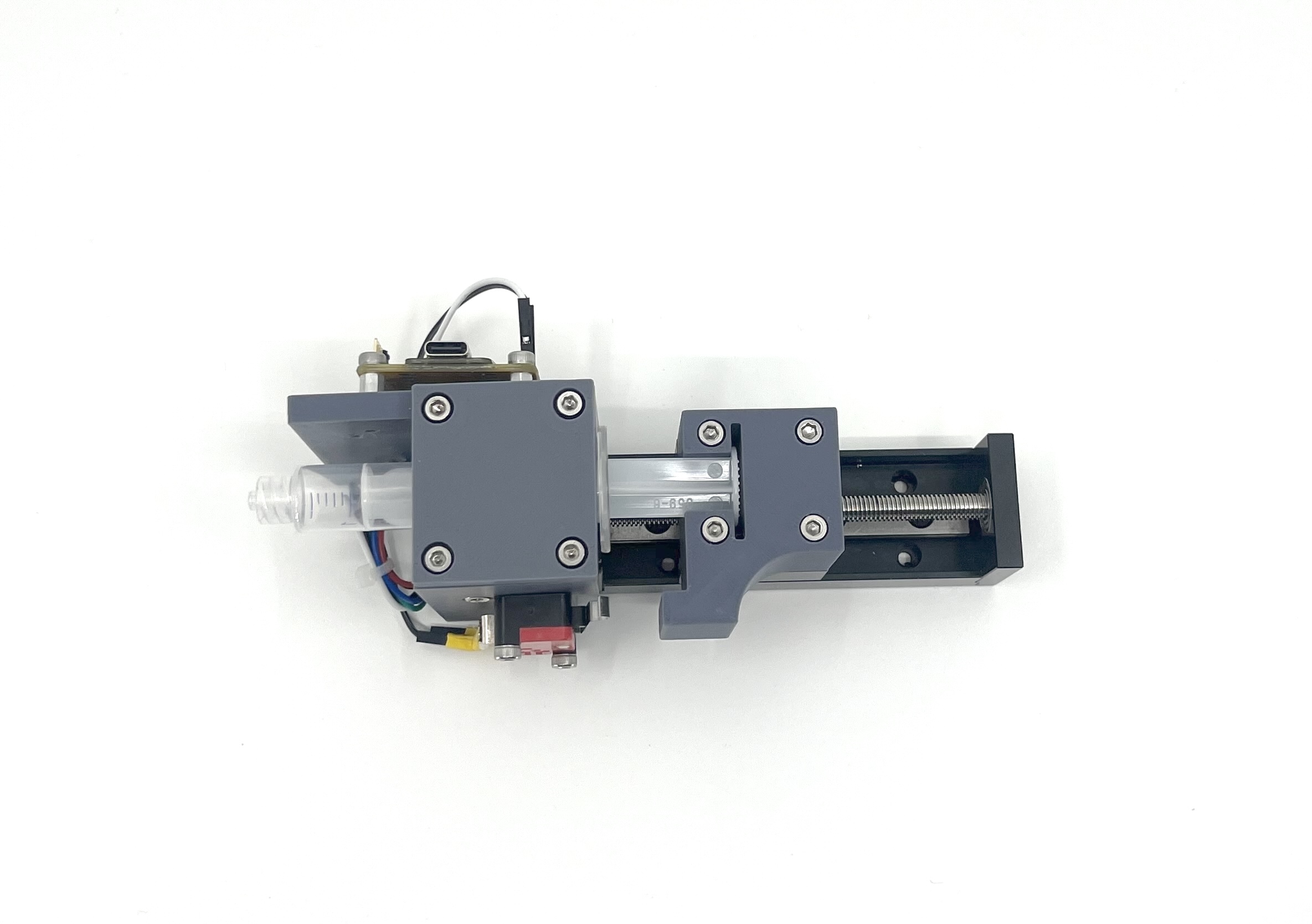