Week 12 Networking
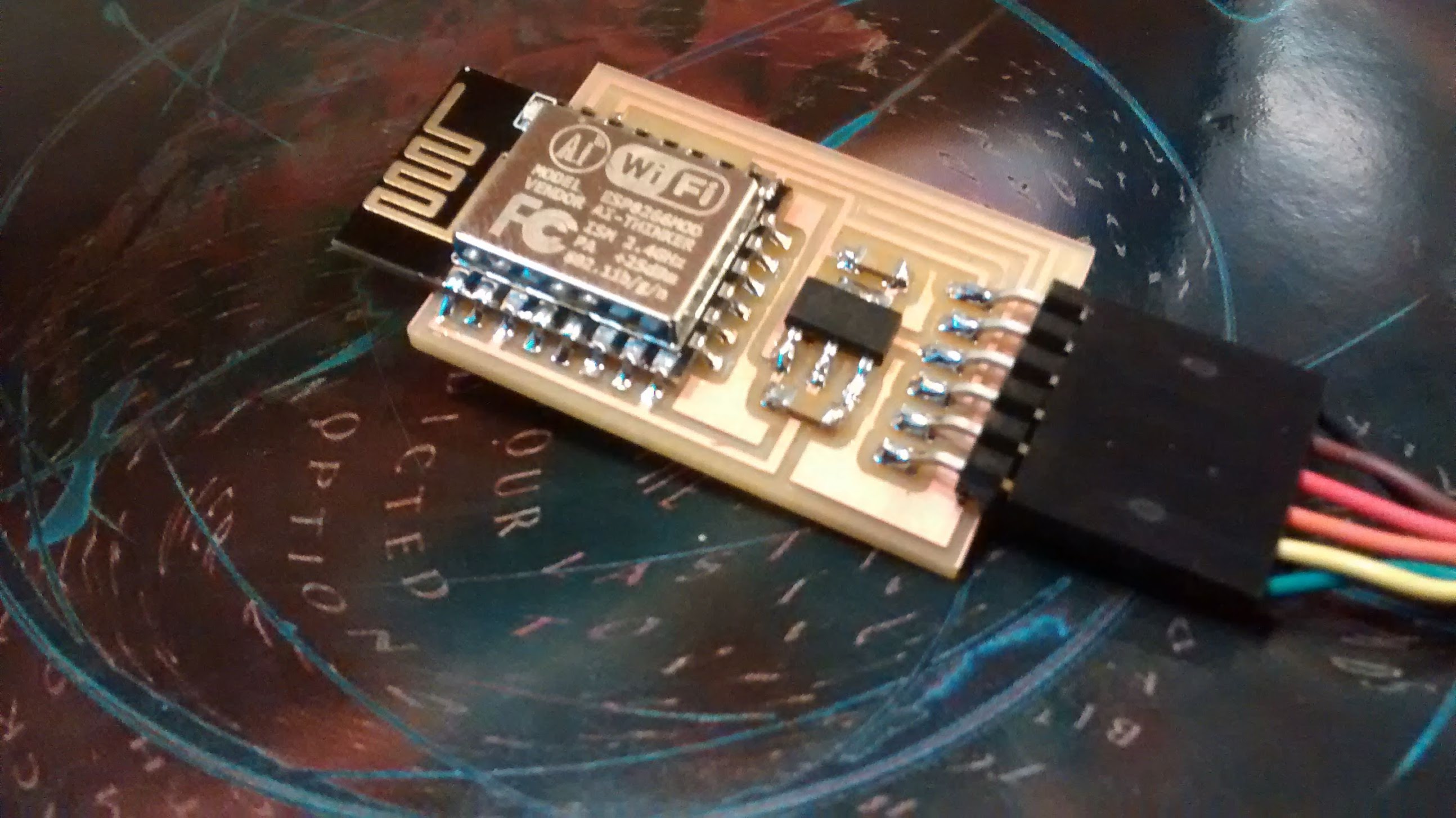
This week I chose to play with the ESP8266 module for networking. I used Neil's hello.ESP8266-12E board as a base (image below).
After milling the board and soldering components, it was the time to figure out how the connections work.
ACCESSING THE WIFI NETWORKS
I initially thought I would be able to connect to this board from Arduino IDE without changing anything on the configuration. However after installing ESP8266 on the Arduino board manager and trying to upload the code, it didn't work. I looked around the website and found the tutorial page which has step by step instructions on how to send commands via python using an ftdi cable.
I downloaded the files and took a look inside. The tutorial asks you to use the following line on the terminal, after you cd to the directory where the files are:
# for a simple connection test
python esp8266test.py [serial_port] [ssid] [password]
# to set the board as a server
python esp8266server.py [serial_port] [baud_rate] [ssid] [password]
For my module, [baud_rate] was 115200. [ssid] and [password] are for the wifi network you want it to connect (I just used 'MIT', which is public). To find the right serial port on the Mac, you can type this into the terminal:
ls /dev/tty*
So your command will be something like this:
python esp8266server.py /dev/tty.usbserial-XXXXXXXX 115200 'MIT' ''
The file esp8266server.py is prepared to send messages to the module and set it up to connect to the networks. The part below is the crucial part:
send_cmd( "AT" )
send_cmd( "AT+CWMODE=1" ) # set device mode (1=client, 2=AP, 3=both)
send_cmd( "AT+CWLAP", 30) # scan for WiFi hotspots
send_cmd( "AT+CWJAP=\""+ssid+"\",\""+pwd+"\"", 5 ) # connect
send_cmd( "AT+CIFSR", 5) # check IP address
send_cmd( "AT+CIPMUX=1" ) # multiple connection mode
send_cmd( "AT+CIPSERVER=1," + str(p))
SHINY AND SMOOTH
Once I got it working, I decided to make a modification on the esp8266server.py file. This would allow anyone who accesses the board IP address through a browser to load a web page, in which GIF files would be shown randomly.
# process requests
while (1):
html = '<html>head>'
html += '<body><div id="main">'
html += '<div id="shiny"><h1 id="title1">shiny!</h1></div><div id="smooth"><h1 id="title2">smooth!</h1></div>'
html += '</div></body>'
html += '<script src="http://carolx.me/fab-class/js/giphyapp.js"></script></body>'
html += '</html>'
process_request(html)
#process_request("GOT IT! (" + str(datetime.datetime.now()) + ")")
print "..."
sleep(0.05)
On the giphyapp.js that I linked, I made a quick GIPHY application so you when you access the board's address, it displays two "almost" random GIFs on a page. If you want to try, first create an app at GIPHY. You will need to create an account if you don't have one. If you don't want to create an app you can try the public key, but that might limit the access.
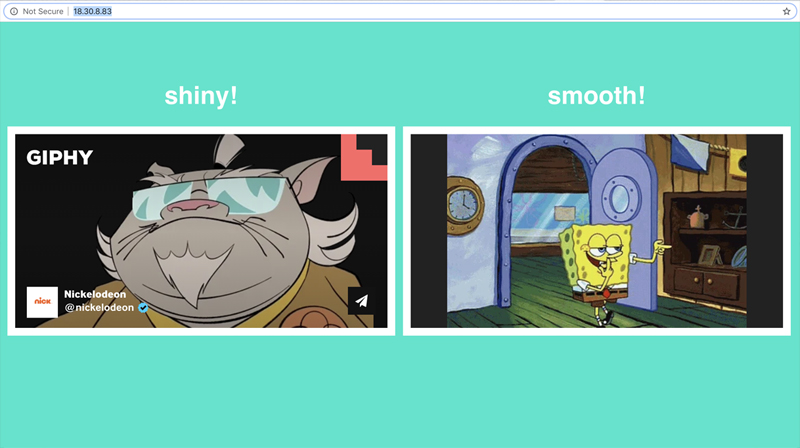
And here's a quick and dirty code for the app that loads 'shiny' & 'smooth' random GIFs:
/* load random "shiny" and "smooth" images from GIPHY */
var body, main, img1, img2, div1, div2;
const httpReq1 = new XMLHttpRequest();
const httpReq2 = new XMLHttpRequest();
const apiKey = 'dc6zaTOxFJmzC'; // get yours from GIPHY after creating your app
const urlShiny='http://api.giphy.com/v1/gifs/search?q=shiny&api_key='+ apiKey +'&limit=1&rating=pg';
const urlSmooth='http://api.giphy.com/v1/gifs/search?q=smooth&api_key='+ apiKey +'&limit=1&rating=pg';
httpReq1.onreadystatechange = function(e){
if (httpReq1.readyState==4 && httpReq1.status==200) {
var json = JSON.parse(httpReq1.response)
console.log(json.data[0])
var gif = json.data[0].embed_url;
img1.setAttribute("src", gif);
}
}
httpReq2.onreadystatechange = function(e){
if (httpReq2.readyState==4 && httpReq2.status==200) {
var json = JSON.parse(httpReq2.response)
console.log(json.data[0])
var gif = json.data[0].embed_url;
img2.setAttribute("src", gif);
}
}
function init(){
main = document.querySelector("#main");
div1 = document.querySelector("#shiny");
div2 = document.querySelector("#smooth");
main.setAttribute("style", "overflow:hidden;");
div1.setAttribute("style", "text-align:center;display:inline-block;color:#fff;width:500px;overflow:hidden;margin:5px;");
div2.setAttribute("style", "text-align:center;display:inline-block;color:#fff;width:500px;overflow:hidden;margin:5px;");
document.querySelector("#title1").setAttribute("style", "font-family:sans-serif")
document.querySelector("#title2").setAttribute("style", "font-family:sans-serif")
document.querySelector("body").setAttribute("style", "background:#00e6cc;text-align:center")
// load and style GIPHY in iframe 1
img1 = document.createElement("iframe");
img1.setAttribute("id", "img1");
img1.setAttribute("width", "480px");
img1.setAttribute("height", "250px");
img1.setAttribute("scrolling", "no");
img1.setAttribute("style", "border: 10px solid #fff; overflow:hidden; background:#222;");
// load and style GIPHY in iframe 2
img2 = document.createElement("iframe");
img2.setAttribute("id", "img2");
img2.setAttribute("width", "480px");
img2.setAttribute("height", "250px");
img2.setAttribute("scrolling", "no");
img2.setAttribute("style", "border: 10px solid #fff; overflow:hidden; background:#222;");
div1.appendChild(img1);
div2.appendChild(img2);
// request shiny GIF
httpReq1.open("GET", urlShiny+"&offset="+Math.floor(Math.random()*100));
httpReq1.send();
// request smooth GIF
httpReq2.open("GET", urlSmooth+"&offset="+Math.floor(Math.random()*100));
httpReq2.send();
}
init();