Week 12: Interfaces and Application Programming
Prior Experience: 4/5
Coming from a software background, this is something I probably have the most experience in out of everything in this class.
The Idea
With the final project coming up very soon, I decided to work on something that might be useful for or related to my final project. I decided to build off of last week's project (where I already started to build a user interface for controlling the color of the RGB LED), but instead of a simple dropdown, have an online version of the sliding tile puzzle game for my final project.
Programming
A basic overview: I used the library ESPAsyncWebServer.h to build a web server to host the game. Like in networking and communications week, I connect to the wifi to be able to communicate between the micrcontroller and web server. then send GET requests to send the website info (as HTML) and get tile movements to update the board state. The board state is kept track as strings in a 2x2 array. I handle tile movements by calling the handler function anytime a tile is clicked on the webpage. Within the function, I check if the tile clicked is adjacent to the empty space, and if so, swap the clicked tile with the empty tile in the board state. I also call the function to check for color change. That function checks each row and column to see if the first two words in the row/column are "LIGHT" and "IS", and if so, sets the color to be the third word in that row/column.
Below, we see that the game page is generated at the root endpoint. In other words, HTML for the page is loaded when at the root URL (i.e. the "home page"). Moreover, tile movement is handled by a GET request with the row and column (of the tile clicked) as parameters. The game page is re-loaded to update the board state.
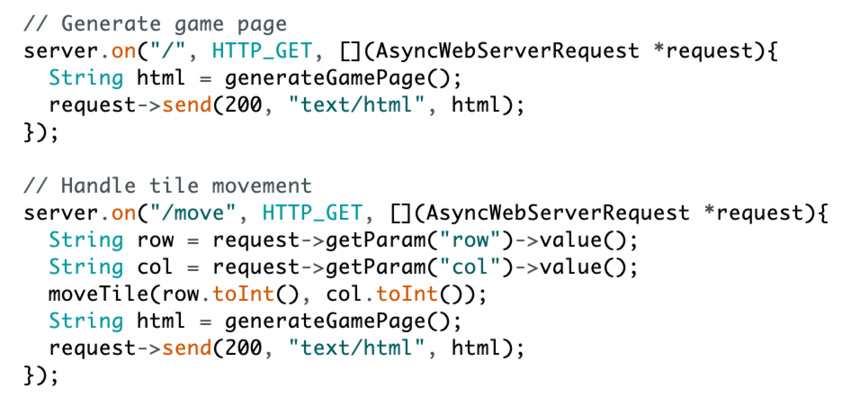
The generateGamePage function generates the HTML for the page. It consists of a grid of divs. Each div has an onClick hander; when a tile is clicked, the moveTile function is called with the row and column of the tile clicked as arguments.
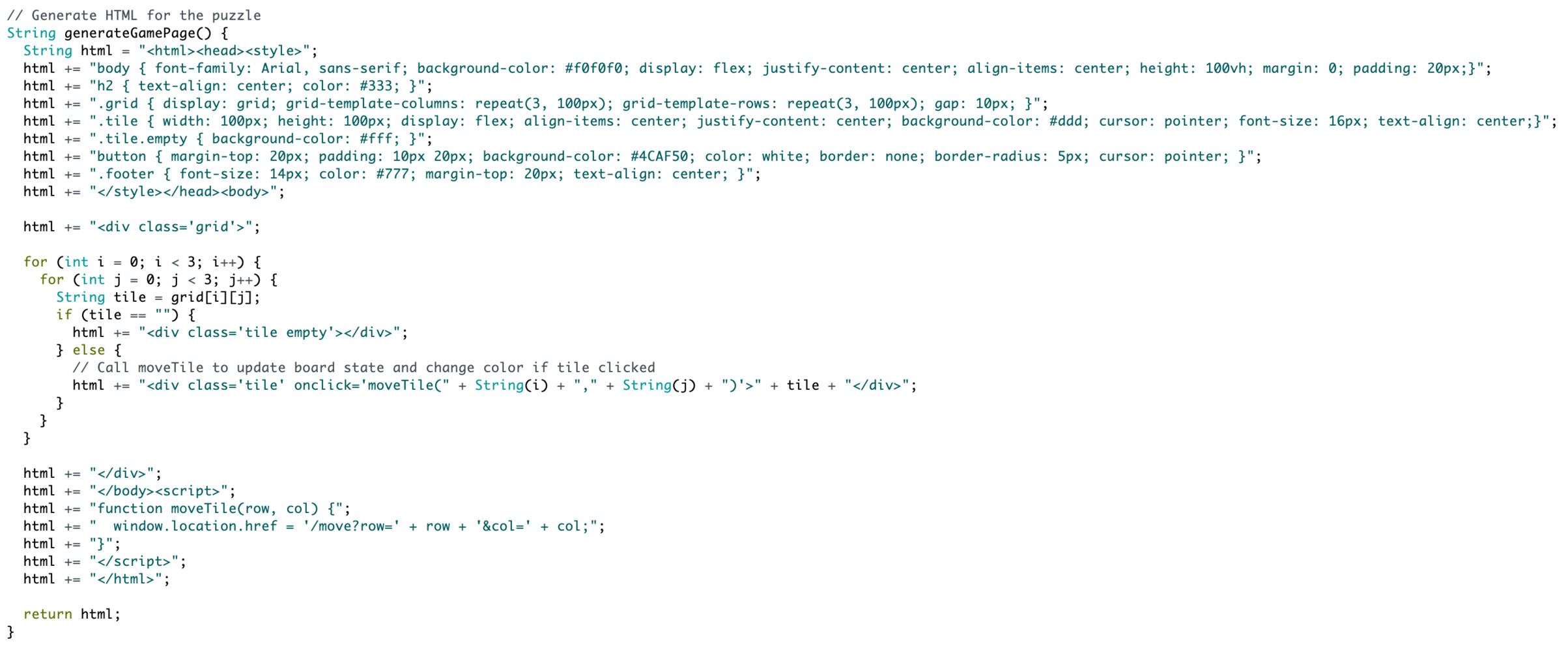
The moveTile function checks whether the tile clicked is adjacent to the empty space, and if so, swaps the tile clicked with the empty space. It also checks the new board state for color change by calling the function.
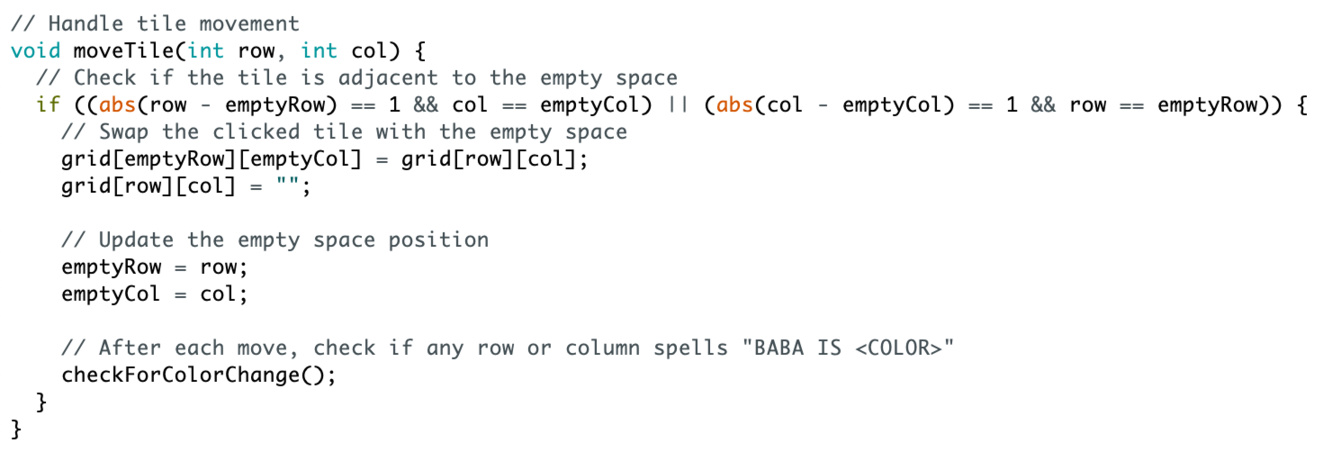
The checkForColorChange function checks whether any row or column spells out "BABA IS [COLOR]", and if so, changes the color of the RGB LED.
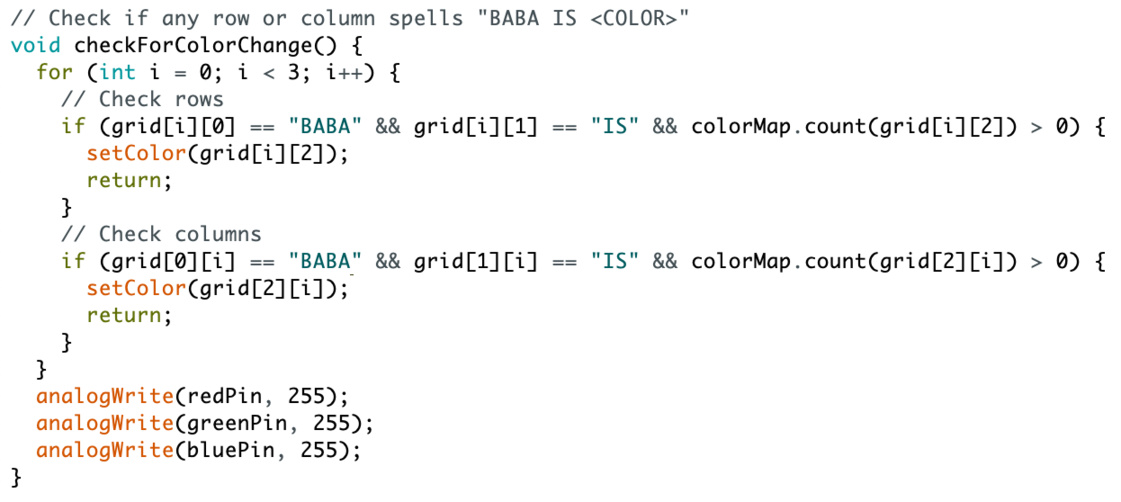
Demo
I access the web page by navigating to the IP address printed in the Serial monitor in a web browser. The code works as expected! When the rule BABA IS [COLOR] is formed on the grid, the LED light changes to that color.