Embedded Programming
Reprogramming the Recreated echo hello-world Board
Basic Programming
For this week I attempted to program something based off of the inital C code that Neil provided but avrc and pin changes had a high learning curve, so I ended up programming my board using the Arduino IDE to take away some of the complexity. First I needed to import my board into my IDE, I used the example from highlowtech to import this and understand which pins I needed to write and how they differed from my schematic. I initially started off very simply and just programmed my board to shine when the button was pressed with the following code:
const int BUTTON = 3; //button connected to attiny44 pin 3
const int LED = 2; //led connected to attiny44 pin 2
void setup() {
pinMode(BUTTON, INPUT);
pinMode(LED, OUTPUT);
}
void loop() {
int buttonState = digitalRead(BUTTON);
if(buttonState==HIGH){
digitalWrite(LED, LOW); // turn the LED off by making the voltage LOW
}
else{
digitalWrite(LED, HIGH); // turn the LED on (HIGH is the voltage level)
}
}
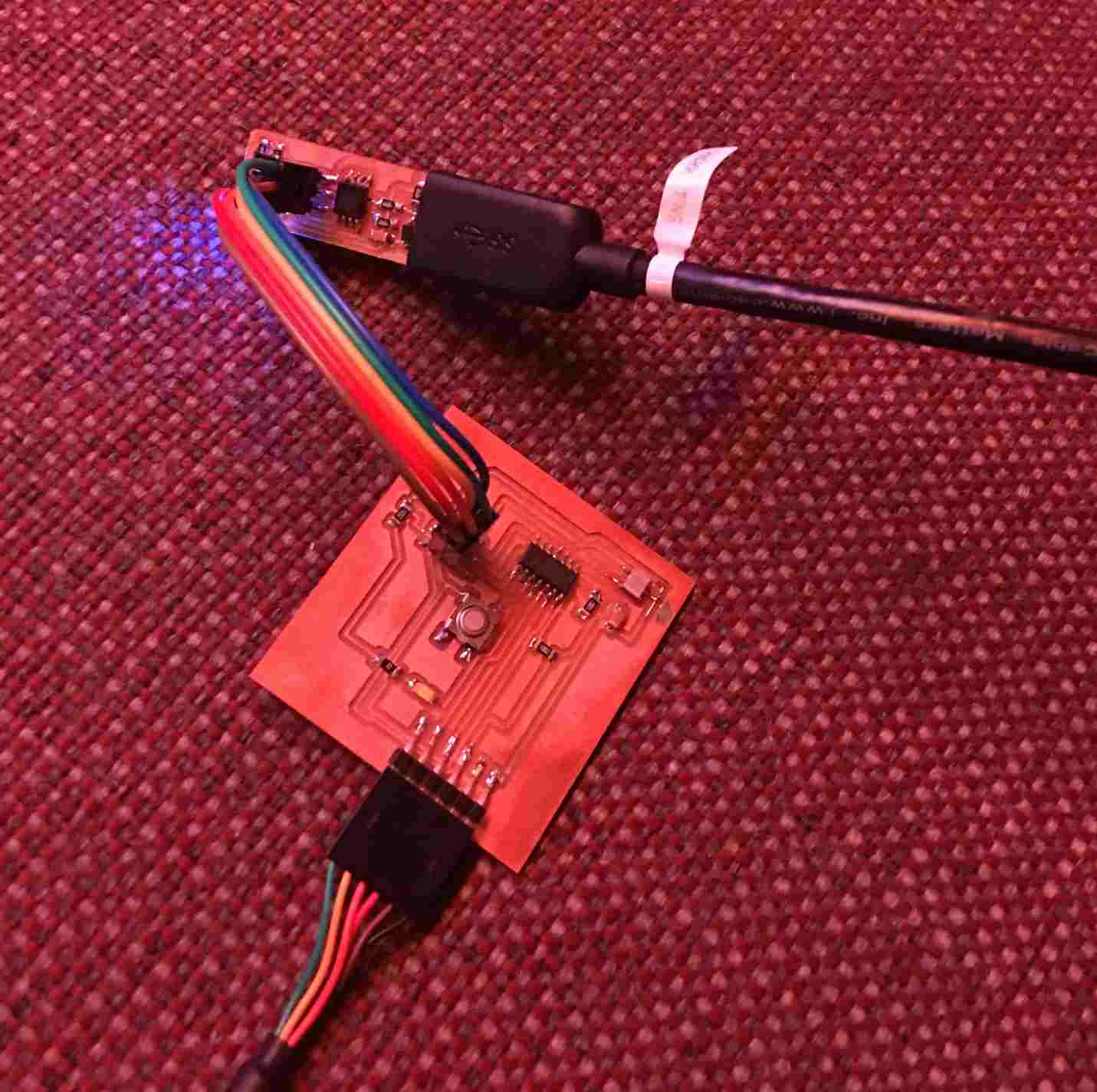
and successfully programmed the board by selecting the board as the attiny44 and the programmer as the USBtiny ISP!
Higher Level Attempt
Building off of this initial code, I wanted to try doing something more advanced to utilize the button on the board. I thought it would be cool to program something that could interpret morse code and translate it it to a string of text it could output in a text file or even email to a designated email address using a mail client. Needless to say I did not get all of this done but would like to circle back to it given more time. I definitely struggled the most with the logic for the loop function and differentiating between when the pauses between button pushes corresponded to the end of a character vs the end of a word vs the end of the message (I did say being course 6-3 was subject to change). You can find my partially written very incorrect code below.
const int BUTTON = 10; //button connected to attiny44 pin 10
const int LED = 11; //led connected to attiny44 pin 11
int morse[5]; //morse code has max 5 dots or dashes
char message[200]; //max 200 char message
unsigned long messageEnd;
unsigned long dot;
unsigned long eom;
unsigned long cTime;
//morse dictionary maybe and just parse the array and look it up...
//need to figure out wtf you do for spaces just a pause I guess... or no spaces
//yeah maybe no spaces this is way harder than I originally thought it would be.
int index;
//need to redo the logic for all of this
void setup() {
pinMode(BUTTON, INPUT);
pinMode(LED, OUTPUT);
cTime = millis();
index=0;
}
void translateMorse(){
//TODO
}
void loop() {
int buttonState = digitalRead(BUTTON);
unsigned long durationStill;
unsigned long durationPressed;
if(buttonState==HIGH){
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
durationStill=millis()-cTime;
cTime=millis();
}
else{
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
durationPressed=millis()-cTime;
cTime=millis();
//logic wrong some things should be when the button is pressed
if(durationStill>=eom&&message.size()>0){
//basically complete the message translate the message and
//email it?
message=new char[200];
index=0;
}
else if(durationStill<=eom&&durationStill<=charPause){
//determine whether pressed was a dot or a dash
if(durationPressed<=dot){
morse[index]=0;
}
else{
morse[index]=1;
}
index+=1;
}
else if(durationStill>=charPause){
//here would want to say the specific morse letter
//has been spelled outtranslate and insertinto char message
morse=new int[5];
}
}
}