
[07] Embedded Programming
Summary
Read a microcontroller data sheet. Program your board to do something, with as many different programming languages and programming environments as possible. For this I am using my USBtiny programmer.
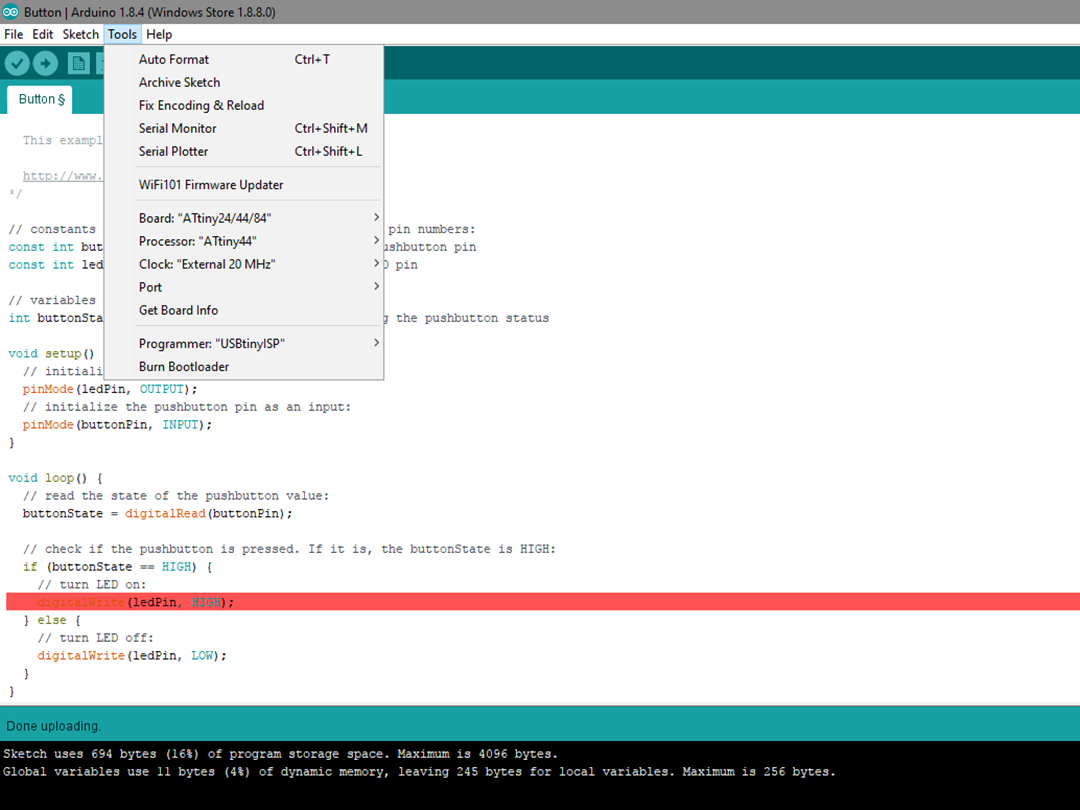
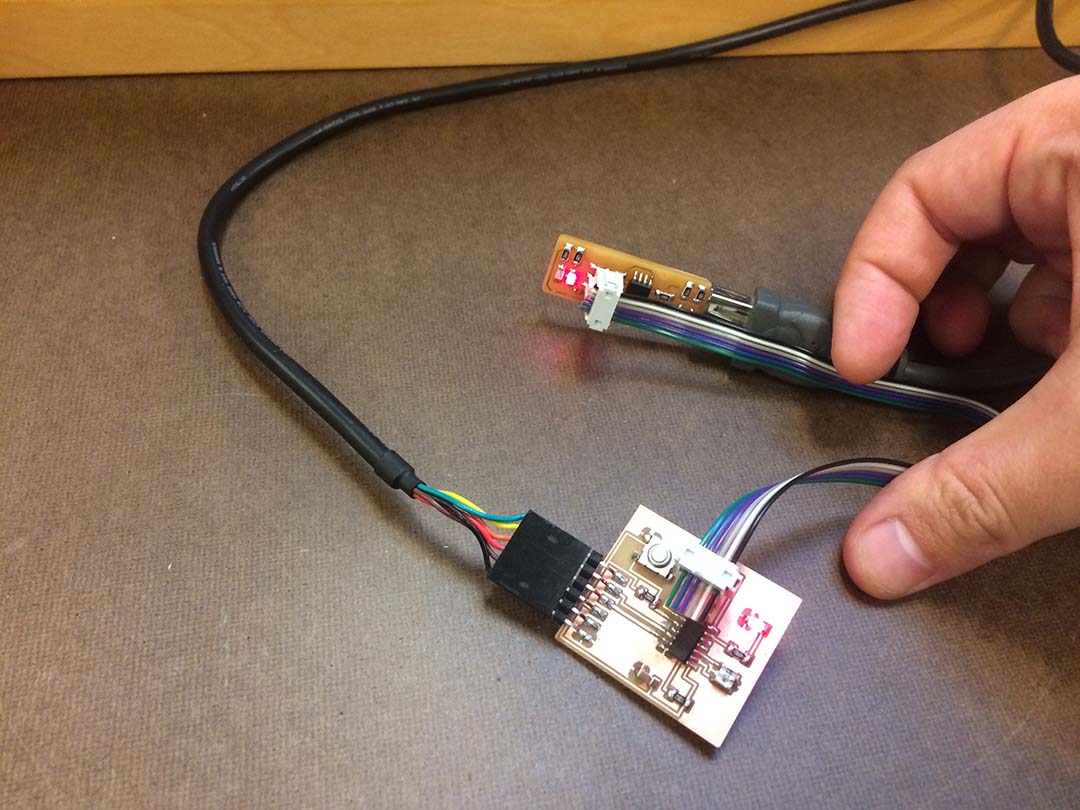
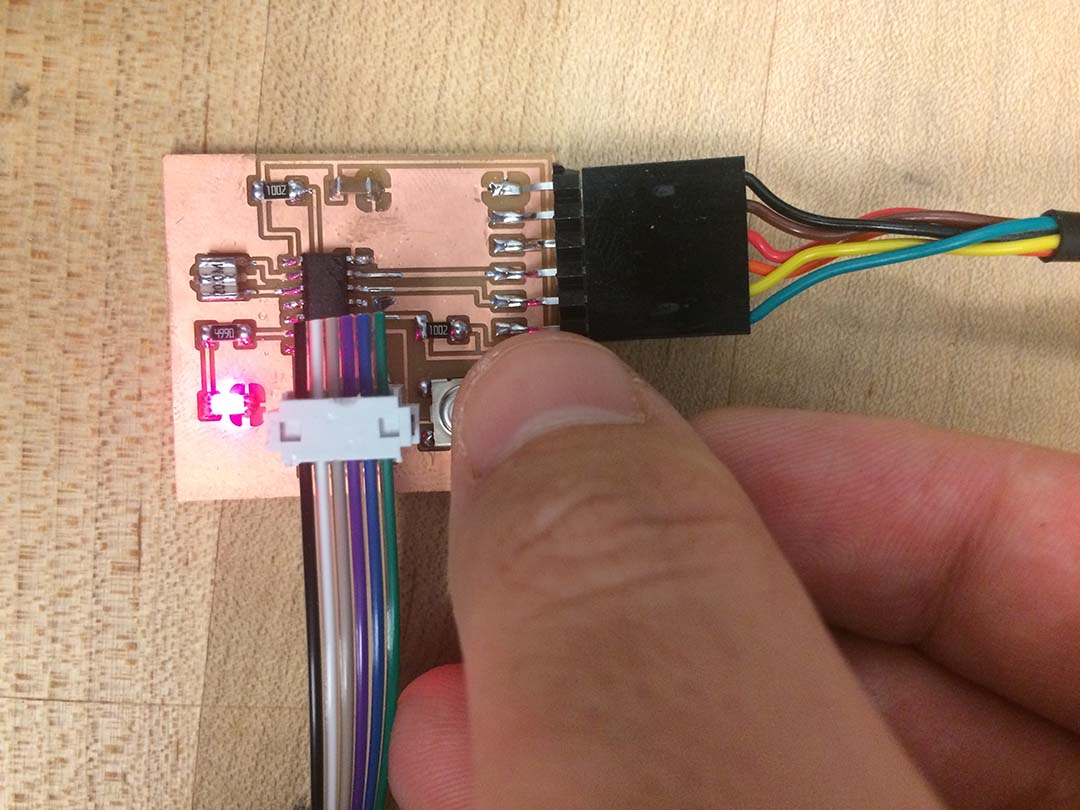
Attiny 44 Datasheet
One of the important things of reading the datasheet is to identify the name or number of the PINS that we are going to use. For example, Arduino uses diferent numbers to identify their PINS. Fo me was very useful to have these 3 images while I was programming.
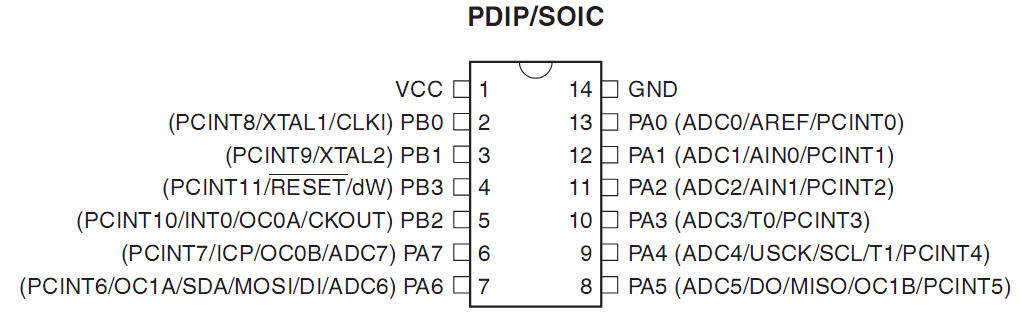
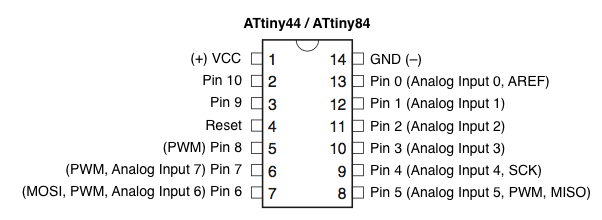
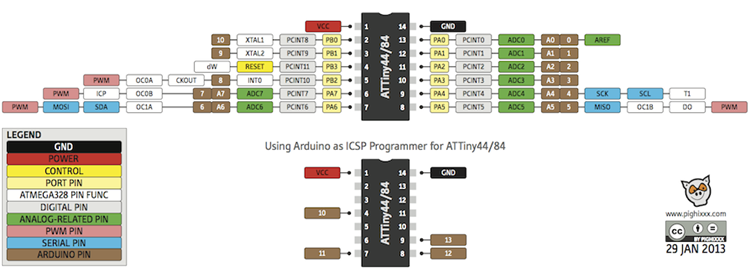
Programming in Ubuntu with AVRDUDE
I used as a template the "hello.ftdi.44.echo.c file and hello.ftdi.44.echo.c.make files, that you can get from the class site.
- Modify the .C code file.
- In the console move to the directory where the your files are.
- Compile the program.
- Tell the microchip to use the external clock so the programmer can talk to it.
- Upload the program use through the terminal.
It is important to identify the name of the pins you are using. In this case I am programming for a blinking light that stop blinking when you press the button.
C Code: Button and blink
Pin Mapping
LED 1= PA7 = Pin 7
Button = PA3 = Pin 3
*/
int main(void)
{
DDRA |= _BV(PA7); // Enable output on LED pin 7
PORTB = _BV(PA3); // Activate button
// Loop
while (1)
{
if (PINA & _BV(PA3))
{
PORTA |= _BV(PA7); // turn LED on
_delay_ms(blink_delay);
PORTA = 0; // turn LED off
_delay_ms(blink_delay);
}
else
{
PORTA = 0; // turn LED off
}
}
}
cd (your path here)/
$ sudo make -f hello.ftdi.44.echo.c.make
$ sudo make -f hello.ftdi.44.echo.c.make program-usbtiny-fuses
$ sudo make -f hello.ftdi.44.echo.c.make program-usbtiny
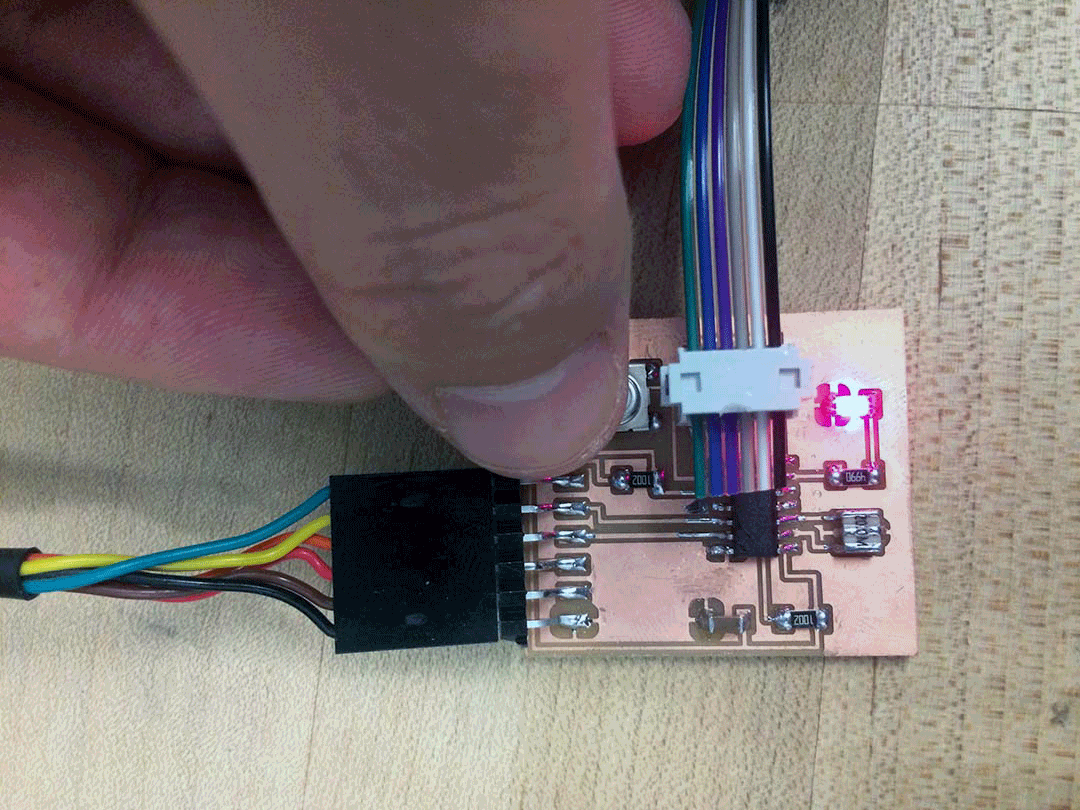
Programming in Arduino
Arduino was easier, the only things is that you need to install the package that lets the Arduino Ide talk to the ATTiny44A.
- Add Board Managers
- Install the board manager.
- Configure the Tools.
- Code.
- Upload the program use through the programmer.
Menu > Preferences - and type:
https://raw.githubusercontent.com/damellis/attiny/ide-1.6.x-boards-manager/package_damellis_attiny_index.json
https://adafruit.github.io/arduino-board-index/package_adafruit_index.json
Tools > Board > Boards Manager
Board: ATtiny24/44/84
Processor: ATtiny44
Clock: External 20MHz
Port: (Choose the correct one)
I used the example code for a Button.
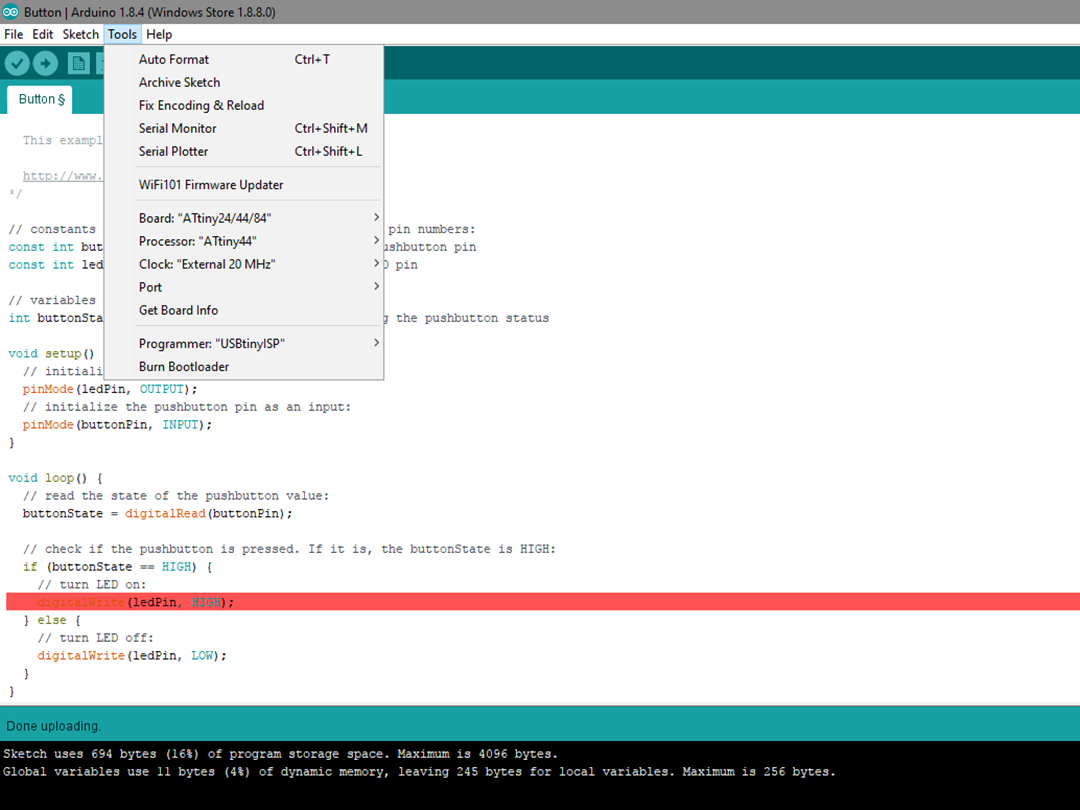