Introduction
In this week, I have learned a very important part of this course -- input devices. Basically, input devices include all kinds of sensors (like temperature sensor, light sensor, etc.) in daily life, as well as controlling devices like buttons, joysticks, etc. All the input devices share a common feature -- change in the measured parameter can be converted into a measurable quantity, like voltage change, relaxation time change and so on.
I'm planning to make use of following input devices:
- 3-axis accelerometer, which will be the sensor that determines the state of my phone. There are two options for this sensor, the analogue one and digital one. I will try both to see which one fit my needs better.
- Joysticks, which will be the controlling devices in order for me to control the position of gimbal actively. For the joystick, I will simply use the ADC to read data.
- Temperature sensor, which will be used to keep the phone warm together with the heater (made of nichrome wire). For this part, I will also use ADC directly.
Besides the three sensors I mentioned in the list, I personally want to try to make a self-designed fit band, with accelerometer and heartbeat sensor installed. The whole circuit will be made by Vinyl cutter, and embedded in silica mold.
Code for ADC on ATMega328p
Here is the code for configuring the ADC and read values and send data to computer. The code here can be applied to almost all kinds of ADC applications, and I have tried them in accelerometer, joysticks, and temperature sensors.
#include <avr/io.h> #include <stdlib.h> #include <util/delay.h> #include <avr/interrupt.h> #include <avr/pgmspace.h> void put_char(){ ... } void put_string(){ ... } //Initialization of ADC void adc_init(void) { ADCSRA |= (1<<ADPS2)|(1<<ADPS1)|(1<<ADPS0); //20MHz/128 = 156.25 kHz ADMUX |= (1<<<REFS0); //use AVCC (+5V) as reference ADCSRA |= (1<<<ADEN); //enable ADC ADCSRA |= (1<<<ADSC); //start a single conversion before actually using the adc } //Read ADC conversion in a given channel uint16_t read_adc(uint8_t channel) { ADMUX &= 0xF0; // Clear the older channel that was read ADMUX |= channel; //Defines the new ADC channel to be read ADCSRA |= (1<<ADSC); //Starts a new conversion while(ADCSRA & (1<<ADSC)); //Wait until the conversion is done return ADCW; //Returns the ADC value of the chosen channel } int main(void) { CLKPR = (1 << CLKPCE); CLKPR = (0 << CLKPS3) | (0 << CLKPS2) | (0 << CLKPS1) | (0 << CLKPS0); adc_init(); set(serial_port, serial_pin_out); output(serial_direction, serial_pin_out); while (1) { x_result = read_adc(0); y_result = read_adc(1); z_result = read_adc(2); itoa(x_result, buffer, 10); put_string(&serial_port, serial_pin_out, "Readout as: "); put_string(&serial_port, serial_pin_out, "X-axis: "); put_string(&serial_port, serial_pin_out, buffer); put_string(&serial_port, serial_pin_out, " "); ... } }
Heart beat sensor
I will talk more about the heartbeat sensor as it seems much more interesting to me. The sensor I used here is purchased from the link here.

I have tried several different designs for the sensor, and below is the latest design:
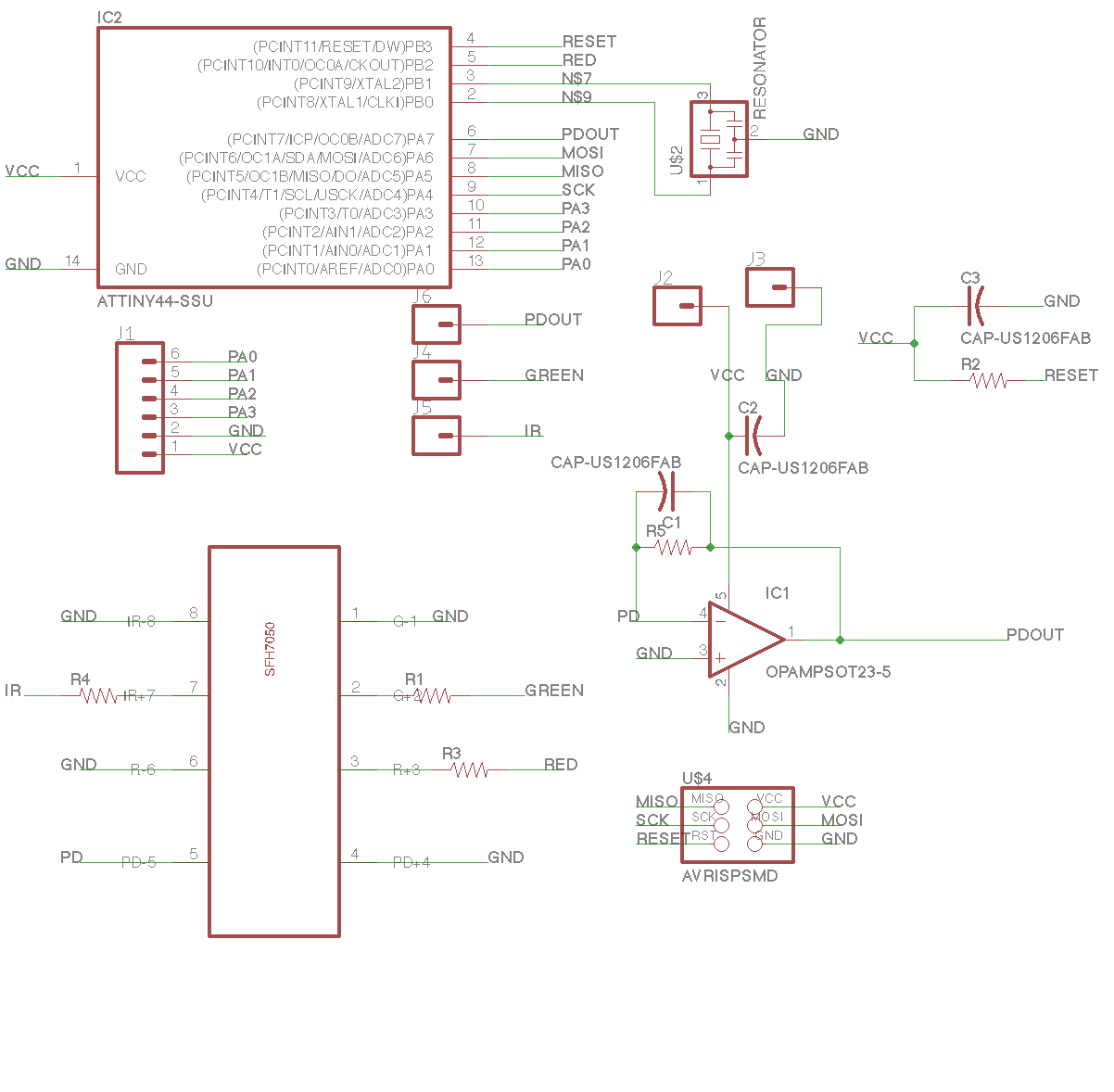
Basically, the three LEDs will blink at certain frequency, and the light reflected by my skin will be detected by the photodiode. Then the generated current will be converted to voltage and amplified by the AD8605 (which works as a preamplifier). The intensity of reflected light will be dependent on the amount of oxygen concentration in my blood, which is directly related to the heart beating process. The op-amp was set to be 100nA/V conversion ratio.
Here is the latest board I made:
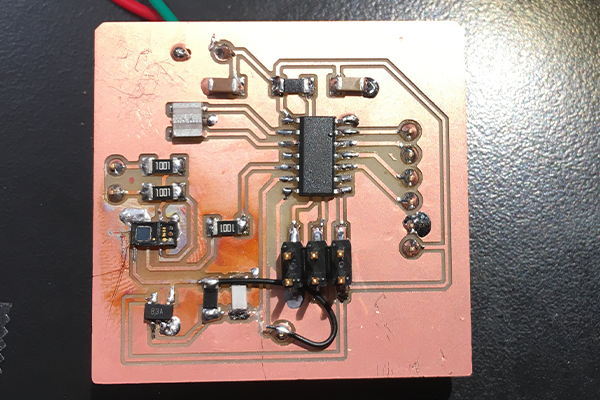
Unfortunately, the signal I got out from the sensor was too messy. I think it's because the signal itself is too noisy, which requires me to add some kind of filter or using noise cancellation methods in the ADC part. I will keep working on this small project, and here is a summary for what I'd like to have for this fit band:
- heartbeat sensor
- accelerometer
- two temperature sensors, one on skin and another outside the band
- transparent silica casting
- bluetooth modules
- (this will be the extra project in addition to my original gimbal project)