Introduction
This is the most exciting week! In this week, I have learned how to program the board I have made several weeks ago. This is a practice for me to get familiar with pulse width modulation control for brushless motor.
Basically, I will make use of the "echo_hello" board I made in week 5. First, I will write the program in C, and then I will write the program onto the board to test it. Optionally, I will use Arduino to do the same thing.
During this week's practice, I have found several problems:
- My programmer only works on Linux computer. I have tried many methods but still cannot make it work on my Mac. The solution for this problem is that I got a Raspberry Pi 3 B+, and log in from my Mac with "ssh" and/or "VNC Viewer".
- The FTDI cable I got from Amazon doesn't work. It turns out that I got a FTDI that only supports Windows.
Blink LED controlled by LED
In this program, when the button is pressed, the blue LED will blink at 5Hz, otherwise it will blink at 2.5Hz.
#include <avr/io.h> #include <util/delay.h> #define set(port,pin) (port |= pin) // set port pin #define clear(port,pin) (port &= (~pin)) // clear port pin #define input(directions,pin) (directions &= (~pin)) // set port direction for input #define led_port PORTA #define led_direction DDRA #define A (1 << PA7) #define B (1 << PA3) int main(void) { //Set clock frequency CLKPR = (1 << CLKPCE); CLKPR = (0 << CLKPS3) | (0 << CLKPS2) | (0 << CLKPS1) | (0 << CLKPS0); input(led_direction,B); while (1) { if ((PINA&B)==0){// Button unpressed, blink every 100ms set(led_port,A); led_delay(); clear(led_port,A); led_delay();} else{ // Button unpressed, blink every 200ms set(led_port,A); led_delay();led_delay(); clear(led_port,A); led_delay();led_delay(); } } }
This is what it looks like when it's working:

First try with a random blinking pattern:
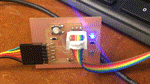
Final result for the program:
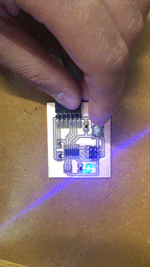
LED blink controlled by serial communication
In this program, the board will blink frequency given through serial communication.
However, I have never succedded making this program work. The problem was that it seems there's something wrong with the font on my Mac. I have tried using both Arduino and coolterm, but neither worked.
Therefore, I only attached the Arduino code for this program, and I will keep thinking about how to realize this function in c.
#include <avr/io.h> #include <util/delay.h> #include <avr/pgmspace.h> #define output(directions,pin) (directions |= pin) // set port direction for output #define set(port,pin) (port |= pin) // set port pin #define clear(port,pin) (port &= (~pin)) // clear port pin #define pin_test(pins,pin) (pins & pin) // test for port pin #define bit_test(byte,bit) (byte & (1 << bit)) // test for bit set #define bit_delay_time 8.5 // bit delay for 115200 with overhead #define bit_delay() _delay_us(bit_delay_time) // RS232 bit delay #define half_bit_delay() _delay_us(bit_delay_time/2) // RS232 half bit delay #define char_delay() _delay_ms(10) // char delay #define serial_port PORTA #define serial_direction DDRA #define serial_pins PINA #define serial_pin_in (1 << PA0) #define serial_pin_out (1 << PA1) #define LED (1 << PA7) #define BUTTON (1 << PA3) #define max_buffer 25 void flash(float freq){ /*flash the LED at fiven frequency, freq (Hz)*/ while (1) { set(serial_port,A); led_delay(); clear(serial_port,A); led_delay(); } } void get_char(volatile unsigned char *pins, unsigned char pin, char *rxbyte) { // // read character into rxbyte on pins pin // assumes line driver (inverts bits) // *rxbyte = 0; while (pin_test(*pins,pin)) // // wait for start bit // ; // // delay to middle of first data bit // half_bit_delay(); bit_delay(); // // unrolled loop to read data bits // if pin_test(*pins,pin) *rxbyte |= (1 << 0); else *rxbyte |= (0 << 0); bit_delay(); if pin_test(*pins,pin) *rxbyte |= (1 << 1); else *rxbyte |= (0 << 1); bit_delay(); if pin_test(*pins,pin) *rxbyte |= (1 << 2); else *rxbyte |= (0 << 2); bit_delay(); if pin_test(*pins,pin) *rxbyte |= (1 << 3); else *rxbyte |= (0 << 3); bit_delay(); if pin_test(*pins,pin) *rxbyte |= (1 << 4); else *rxbyte |= (0 << 4); bit_delay(); if pin_test(*pins,pin) *rxbyte |= (1 << 5); else *rxbyte |= (0 << 5); bit_delay(); if pin_test(*pins,pin) *rxbyte |= (1 << 6); else *rxbyte |= (0 << 6); bit_delay(); if pin_test(*pins,pin) *rxbyte |= (1 << 7); else *rxbyte |= (0 << 7); // // wait for stop bit // bit_delay(); half_bit_delay(); } int main(void) { //Set clock frequency CLKPR = (1 << CLKPCE); CLKPR = (0 << CLKPS3) | (0 << CLKPS2) | (0 << CLKPS1) | (0 << CLKPS0); }
After that, I have tried using Arduino as programmer to repeat the same program listed above.

Finally, I solved the problem of gibberish by changing the bit_delay_time to 8 sec instead of using 8.5 sec.